The trick to viewport units on mobile
Viewport units have always been controversial and some of that is because of how mobile browsers have made things more complicated by having their own opinions about how to implement them.
Case in point: should the scrollbar be taken into account for the vw unit? What about a site’s navigation or page controls — should those count in the calculation? Then there are physical attributes of the devices themselves (hello, notch !) that can’t be overlooked.

First, a little context
The spec is pretty vague about how viewport units should be calculated. With mobile devices, we’re often concerned with the vertical height, so let’s look specifically at viewport height ( vh ):
vh unit Equal to 1% of the height of the initial containing block.
So yeah, no clear guidance there when it comes to handling device and browser-specific differentiations.
vh was initially calculated by the current viewport of your browser. If you opened your browser and started to load a website, 1vh was equal to 1% of your screen height, minus the browser interface.
But! If you start scrolling, it’s a different story. Once you get past a piece of the browser interface, like the address bar, the vh value would update and the result was an awkward jump in the content.
Safari for iOS was one of the first mobile browsers to update their implementation by choosing to define a fixed value for the vh based on the maximum height of the screen. By doing so, the user would not experience jumps on the page once the address bar went out of view. Chrome’s mobile browser followed suit around a year ago .
As of this writing, there is a ticket to address this in Firefox Android .
While using a fixed value is nice, it also means that you cannot have a full-height element if the address bar is in view. The bottom of your element will be cropped.

CSS Custom Properties: The trick to correct sizing
The idea struck me that CSS Custom Properties and a few lines of JavaScript might be the perfect way to get the consistent and correct sizing I needed.
In JavaScript, you can always get the value of the current viewport by using the global variable window.innerHeight . This value takes the browser’s interface into account and is updated when its visibility changes. The trick is to store the viewport value in a CSS variable and apply that to the element instead of the vh unit.
Let’s say our CSS custom variable is --vh for this example. That means we will want to apply it in our CSS like this:
OK, that sets us up. Now let’s get the inner height of the viewport in JavaScript:
We told JavaScript to grab the height of the viewport and then drilled it down into 1/100th of that total so we have a value to assign as our viewport height unit value. Then we politely asked JS to create the CSS variable ( --vh ) at the :root .
As a result, we can now use --vh as our height value like we would any other vh unit, multiply it by 100 and we have the full height we want.
There is another fix for this that has come along more recently. Matt Smith documents it here . The trick is min-height: -webkit-fill-available; on the body as a progressive enhancement over 100vh , which should work on iOS devices.
Whoa, there! One more little detail.
While our work might look done at this point, those of you with an astute eye for detail may have caught that the JavaScript fires but never updates the size of our element when the viewport’s height changes. Go ahead and try resizing the demo above.
We can update the value of --vh by listening to the window resize event. This is handy in case the user rotates the device screen, like from landscape to portrait, or the navigation moves out of view on scroll.
⚠️ Updating the value of --vh will trigger a repaint of the page and the user may experience a jump as a result. Because of this, I’m not advising that this trick should be used for every project or to replace all usage of the vh unit but only when you may need your users to have an exact viewport unit value.
Also, you may want to implement a debounce method for the resize event to avoid triggering to many events while the user is resizing their browser’s window. You can learn more about it with this article: Debouncing and Throttling Explained Through Examples
You can now resize the demo above and notice that the CSS variable is updated accordingly.
While I recently used this technique on a project and it really helped, you should always think twice when replacing the browser’s default behaviors. (For example, this comes up a lot with ::focus .) Also, browsers tend to update very fast these days, so beware that today’s solution may not work tomorrow.
In the meantime, I hope this article helps! 👋
Here’s a proposal for vhc and vwc units that may be a savior in all this.
You should really use some kind of throtteling when listening to the resize event especially if it is triggering a repaint — for example like documented here: https://devdocs.io/dom_events/resize
Thanks for your feedback, I added a small note the debounce technique but I didn’t want to add extra code into the demos to keep them clear ✌️
Haven’t tried that yet but I struggled with that problem for months! Thank you very much will try this to fix my website soon.
Could you elaborate your use case? You need an in-flow full-height element at the top of the page?
I’ve read somewhere (I think, on nngroup.com) that it’s best to make such an element slightly smaller, so that the user knows that they can scroll down. (Apparently, some users will assume that there is no content below.)
That’s exactly the use case where I needed it. The first screen of the website was supposed to be full-height on mobile but we got the bottom cropped (which was the client’s logo). You could also use this trick if you have a modal that should be 100vh and you don’t want your users to loose the bottom part because of the browser’s interface.
I was struggling with this exact issue a month ago and came up with similar solution :D
I wasn’t doing –vh in root but in that element that needed vh unit only and with jQuery as the project was in jQuery. But the concept is the same.
Similar fix width modal overlay, when body tag overflow hidden:
in js: function getScrollbarWidth() { return window.innerWidth – document.documentElement.clientWidth; } document.documentElement.style.setProperty(‘–scrollbar-width’, ${getScrollbarWidth()}px );
in css: body.modal-opened { padding-right: calc(var(–scrollbar-width)); }
Just a side node – probably it’s better to call variable –vh100, as long it is “100vh”, not a single unit.
If you only need full-height elements, yes you could skip the calc part and set the variable to 100% of window.innerHeight. But if you need an element to be 50vh or else, you can use the variable and multiple it like so: height: calc(var(--vh, 1vh) * 50);
A really nice solution.
However there is a problem – if any script execution fails, JS fails to load or loading takes a long time, you’re going to have an unusable site.
Add a .js class to the body and make the calc height apply only when JS has loaded – The 100vh is both the fallback and non-js/slow loading version
There is already a fallback in the CSS in case the JavaScript doesn’t run. In this line: height: calc(var(--vh, 1vh) * 100); there is var(--vh, 1vh) where 1vh is a fallback. This is not really mentioned in the article but CSS Custom Properties can have fallback if the property is not defined. You can read more about this here: https://developer.mozilla.org/en-US/docs/Web/CSS/var You could also add a default value on the root in your CSS.
I use the following code, never had an issue with 100vh not actually occupying the whole height.
This gets rid of the default behaviour. To me it looks like the issue you are having is caused by it.
After that IIjust manually add margins and padding as needed.
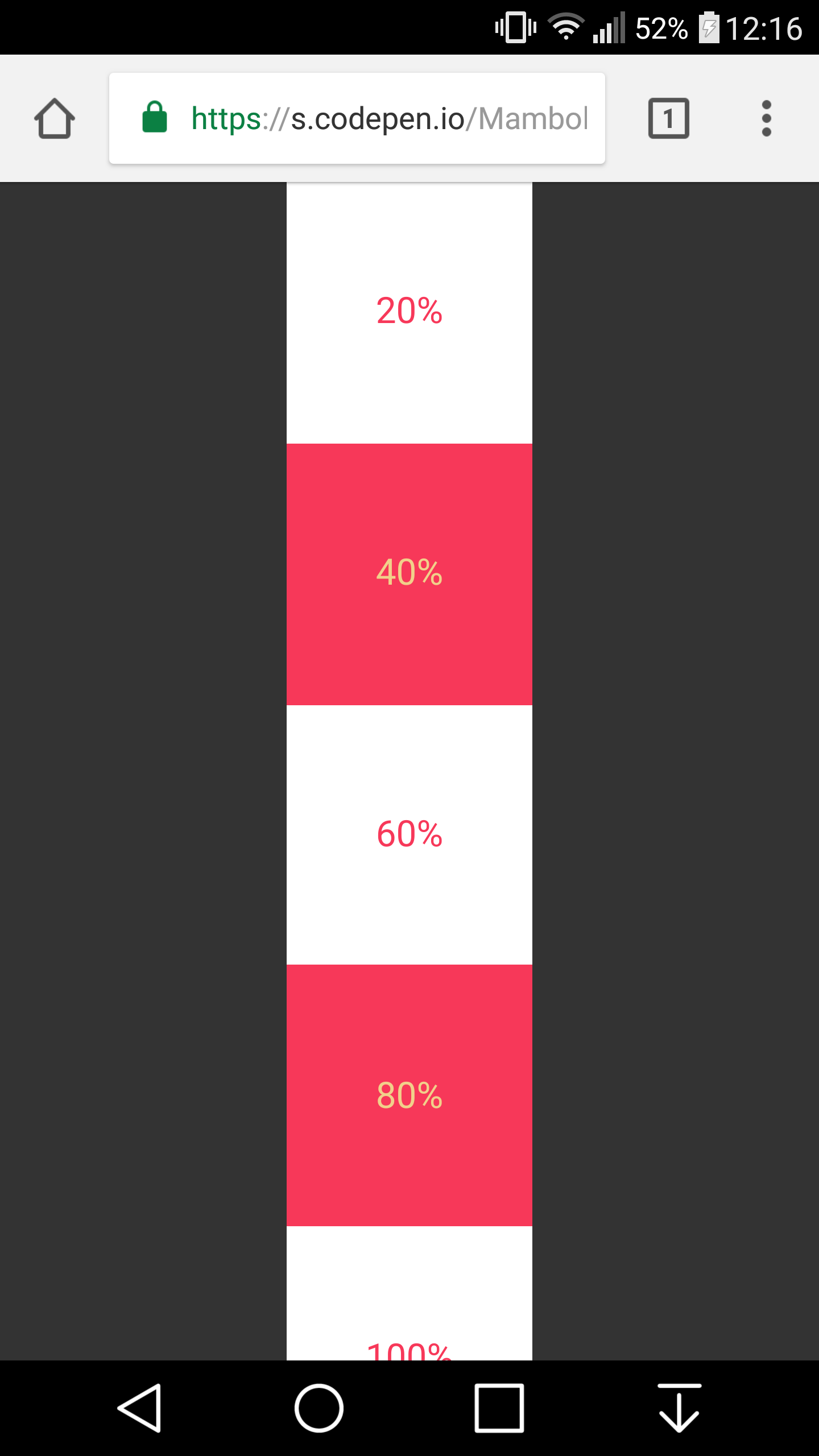
I got that working on chrome on my mobile device, but it is not working for Safari :(
Louis, please ignore my first comment, after looking into issue myself I have discovered more than I had hoped for. I always assumed that viewport height would be.. you know viewport height. Not the the mess it actually is.
So I have been researching a bit.. it appears that only solution that is somewhat reliable is the one you write about in your post maybe with some media queries… I am currently looking into it.
Meanwhile I made a little demo which seems to work fine, sort of… Ill try to use orientationchange event listener to handle the change of orientation and manually adjust height of pages which are below first 2 screen heights, because the URL bar will be always hidden at that point.
http://www.patriklegard.com/app
THANK YOU – this issue has irritated me for ages, and it seems obvious now but it actually never occurred to me to solve the problem this way using innerheight.
I wouldn’t recommend using the resize event though since the height of the element is then forced to change as you scroll on mobile(especially evident on safari ios). Meaning if there’s a background image on the element that is set to cover it makes the background position change, and will also affect any absolute positioned things inside that element too.
To avoid this issue I let the script only update my vh var when the resize is substantial enough(or in this case any landscape mode, mostly the desktop users)
I am running into this issue on an aside with a sticky footer. I always want the footer to be visible since it contains the cancel and submit buttons but depending on scrolling it might show correcrly and it might not. Do you have any suggestions on a sticky footer in an aside on a mobile device?
Just in case somebody else runs into this issue, apparently in Chrome, window.innerHeight doesn’t return the correct viewport height if you’re in Device Mode in Dev Tools. I was trying to use this method on a personal website of mine but was stumped when I went into device mode to check how it looks on iOS and the console log showed a different value for innerHeight then the device viewport height. Firefox and Safari showed correct values but Chrome did not.
I found this article which seems to clarify the reason: https://developers.google.com/web/updates/2017/09/visual-viewport-api
I needed to console log window.visualViewport.width if I wanted Chrome to use the visual viewport of the device. But if you’re actually on your mobile device innerHeight works fine, it’s just that when you’re emulating a mobile device on your laptop in Chrome dev tools innerHeight is not going to work as you may expect.
oops I meant window.visualViewport.height
Just nitpicking but might as well swap that let for const .
- Web Development
Fixing the iOS Toolbar Overlap Issue with CSS Viewport Units
One of the most exciting aspects of web development in recent years has been the continued growth and improvement of CSS. Flexbox and grid revolutionized how we build webpage layouts. Custom properties (aka, CSS variables) opened up new possibilities for theming. Newer selectors like :has() , :is() , and :where() have lead to more powerful and concise CSS. Container queries are now a reality and looking ahead, native CSS nesting is on its way ( decisions concerning its syntax notwithstanding).
But all of these new and upcoming features means that CSS is becoming increasingly complicated. Inevitably, then, some new features might fall through the cracks and get overlooked. (Which is why I’m of the opinion that “full-time CSS engineer” ought to be a thing, but that’s a topic for another post.) Speaking personally, I’m still pretty ignorant concerning the benefits of newer color formats like hwb() and lch() . Which brings me to viewport units.
Put simply, viewport units allow you to size a page’s elements relative to the size of the browser’s viewport , which contains everything that is currently visible on a webpage. (The viewport is basically the browser window minus any UI elements like the navigation and search bar.)
Consider this very simple example:
The vh stands for “viewport height,” so an element set to 100vh will be 100% of the viewport’s height. If that element’s height is set to 50vh , then it’ll be 50% of the viewport’s height, and so on. The 100vh is often used when you want an element to fill up the entire browser window. For instance, I use this technique on special features (like my recent David Zindell post ) to make their headers fill up the entire viewport with a splashy image. This gives them some extra visual oomph that sets them apart from my “normal” posts.
Not All Viewports Are the Same
This approach works well except for one pretty prominent scenario. If you’re viewing such a layout in Safari on an iOS device, that 100vh element fills up the viewport, but its bottom portion is then covered by a toolbar that includes the next/previous navigation and other controls. (See Figure A.)
Note: Although I’m focusing on iOS Safari, this issue also occurs in iOS Chrome. It doesn’t occur in other iOS browsers like Brave, DuckDuckGo, Firefox, and Opera. (More on that in a moment.) I haven’t tested this in any Android browsers.
In other words, Safari doesn’t seem to take its own UI into consideration when drawing its viewport. Thus, a 100vh element doesn’t behave the way it seems like it should, i.e., filling up the space between the URL bar and the bottom toolbar. (Remember that a browser viewport is the browser window minus any UI elements.)
There are, of course, reasons for why Apple opted for this approach. And reading the developer’s explanation — the viewport’s height changes dynamically because any toolbars disappear or minimize when you scroll — they seem perfectly valid. But that doesn’t mean I liked how it looked. It was hard to believe that this was still an issue the Year of Our Lord 2023.
Various Google searches returned different solutions, including some JavaScript-based workarounds . Using JavaScript to fix visual layout issues, however, always feels hack-y to me. Call me old-fashioned, but I like to keep my CSS and JavaScript nice and separate, and reserved for those things that they do best (e.g., CSS for layout, JavaScript for interactivity).
That aforelinked article also pointed me to Matt Smith’s article about -webkit-fill-available , which seemed promising at first. Unfortunately, it wasn’t applicable to my situation. I didn’t want the post header to simply fill up the entire available space because I also needed to take into account the height of my site’s header, which contains the logo, nav, and search.
Here’s what my original CSS looked like:
The site header is 6 rems high, so I use the calc function to subtract that from the 100vh to dynamically calculate the post header’s new height. But, as pointed out before, iOS doesn’t respond to 100vh the way you might think it would. What I really needed was a new type of CSS unit — and fortunately, I found it.
New Viewport Units
Back in November, Google’s Web.dev blog covered three new viewport units: the “large,” “small,” and “dynamic” viewport units . These units were created specifically to work with viewports whose size might change due to dynamic toolbars — which was the exact problem I was facing .
- The “large” viewport units assume that any dynamic toolbars (e.g., Safari’s bottom bar) are retracted and hidden , and calculate the viewport’s size accordingly. (This is akin to Safari’s aforementioned default behavior.)
- The “small” viewport units assume that any dynamic toolbars are expanded and visible , and calculates the viewport’s size accordingly.
- The “dynamic” viewport units sit in-between the “large” and “small” units, and react automatically to the dynamic toolbar’s behavior.
At first glance, a “dynamic” viewport unit seemed like the solution. After all, who doesn’t like a web design that automatically responds, all on its own, to a given situation? With that thought in mind, I updated my CSS:
In addition to the original selector, I added a feature query via @supports that basically says if the browser recognizes and supports the height: 100dvh declaration, then run the following CSS. (This is an example of progressive enhancement , i.e., starting with the basics and then adding on more advanced code that modern browsers will recognize.) That CSS is virtually identical to my original CSS, except I’m now using 100dvh instead of 100vh . (The dvh stands for “dynamic viewport height.”)
The first time I loaded the page, the problem seemed to be solved: the post header now filled up the space between Safari’s toolbars without anything cut off or hidden. But then I scrolled a little bit.
When you scroll down in iOS, the browser’s toolbars disappear or reduce in size, thus increasing the height of the browser’s viewport. Conversely, scrolling back to the top causes the toolbars to reappear or return to their original size, thus decreasing the viewport’s height. This behavior caused some distracting (IMO) changes to the post header: the background image expanded while the text shifted down in response to the additional height.
Interestingly, this “dynamic” approach is the behavior employed by the iOS versions of Brave, DuckDuckGo, Firefox, and Opera. In other words, toolbar overlap appears to be a non-issue for them, at least as far as Opus is concerned.
So after giving it some more thought, I replaced 100dvh with 100svh — i.e., the “small” viewport height — which assumes that any toolbars are always expanded.
Here’s my final code:
You can see the results — that is, the entire post header — in Figure B. Upon scrolling, the post header doesn’t take advantage of the increased viewport height, so it’s not a truly “full-height” element. However, it doesn’t have any weird shifting, either, but looks the same all the time. And I always prefer such stability in my web designs.
For what it’s worth, Firefox, Brave, et al . ignore the 100svh setting altogether, and instead, always stick with the “dynamic” handling of the viewport and post header heights. That’s a little frustrating, but since they represent a relatively minuscule amount of Opus ’ overall traffic, I’m not going to sweat it.
Final Thoughts
Along with the aforementioned color formats, viewport units are one of those aspects of CSS that has always felt rather abstract to me. (Then again, I still have trouble wrapping my mind around how srcset works, and that’s used all the time for responsive images.) The problems they seek to address have often seemed rather niche to me, compared to the issues that I’m trying to solve 95% of the time.
Of course, now I have to eat a little crow because I found myself in just such a “niche” situation. Which is to say, I’m glad that really smart people have spent time thinking through these situations, rarefied as they might seem, to find and propose potential solutions.
I’m also glad that browser makers are quick to implement them; browser support for these new viewport units is pretty good, with Opera being the only major holdout. (Which means that I’ll probably remove the @supports feature query in the not-too-distant future and use the 100svh as the default CSS.)
Finally, while Safari’s behavior was initially frustrating, I do believe they made the better choice concerning how to handle dynamic toolbars and viewport heights now that I’ve seen how Firefox et al . handle them. I’d rather have part of the design covered up by default (but fixable, if needed, with the right CSS) then see the page rearrange itself as you scroll. The latter behavior is unexpected and thus distracting, two things that can create a poorer user experience — which is something I try to avoid in every aspect of my designs.
Does Safari 15 finally fix viewport height?
Written by Luke Channings on June 11, 2021
The design for Safari 15 has been very controvercial, and has changed significantly since the beta that this article was based on Sadly, one of the casualties of the evolving design was the main thrust of this article: env(safe-area-inset-bottom) is no longer dynamically the height of the address bar in the final release.
TL;DR : No , but if you subtract env(safe-area-inset-bottom) from 100vh you'll get what you want .
Safari 15's UI is a radical departure from the previous version — and from web browsers in general — but does it fix the viewport height problem?
What is the viewport height problem again?
Mobile Safari has had problems related to 100vh not behaving like web developers expect 1 2 pretty much since the beginning. The main crux of the issue is that Mobile Safari's UI Chrome shrinks when you scroll, and expands again when you activate it. That means 100vh ("100% the height of the viewport") can't be a static number.
Let's start by understanding the definition of the vh unit 3 :
vh is defined as Equal to 1% of the height of the initial containing block . — Anthony Frehner
And here's the best explanation of the 100vh issues in Mobile Safari that I've seen so far,
The core issue is that mobile browsers (I’m looking at you, Chrome and Safari) have a “helpful” feature where the address bar is sometimes visible and sometimes hidden, changing the visible size of the viewport. Rather than adjusting the height of 100vh to be the visible portion of the screen as the viewport height changes, these browsers instead have 100vh set to the height of the browser with the address bar hidden. The result is that the bottom portion of the screen will be cut off when the address bar is visible, thus defeating the purpose of 100vh to begin with. — David Chanin , Avoid 100vh On Mobile Web
Let's put this new Safari to the test
I have a simple HTML page based on the example given in David's article. It has a header at the top and a button at the bottom, all wrapped in a 100vh container.
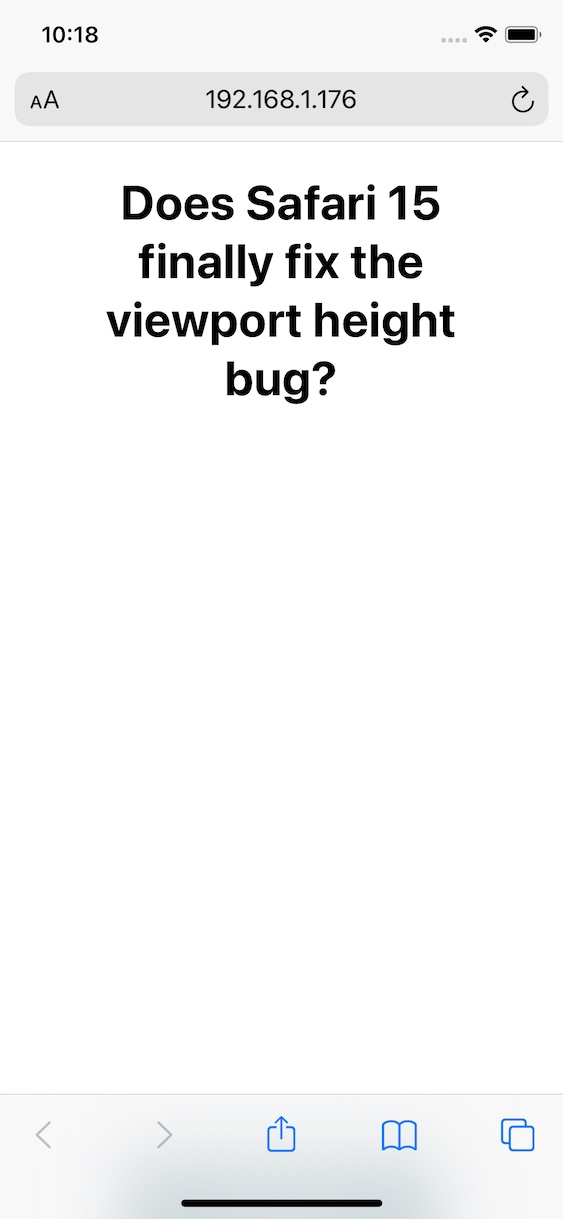
Safari's new floating address bar is displayed above our test button, which is more-or-less exactly the same behaviour as iOS 14.
So - Safari 15 does not change the behavour of 100vh 😢.
So what's the solution then?
It makes sense to me that the WebKit team wouldn't change the behaviour of the viewport unit, it's already well defined.
Do you remember when Apple introduced env() and safe-area-inset so that web developers could avoid their content being shown behind the notch 4 ?
Well in Safari 14, safe-area-inset-bottom is 0px whether the UI chrome is active or inactive, which is something that has annoyed me for a while.
safe-area-inset-bottom is 0px when the UI chrome is inactive in Safari 15 on iOS, and then the height of the collapsed chrome minus the height of the expanded chrome when the bar is expanded.
That means that to get a button to float at the bottom of the page, always above the UI Chrome, all you have to do is use calc(100vh - env(safe-area-inset-bottom)) .
Wrapping up
So not only does safe-area-inset-bottom work in Safari 15, it's animated !
I've been hoping that something to remedy the viewport height bug was coming since Jen Simmons (who joined the Safari / WebKit team in June 2020) was asking for feedback regarding viewport height issues.
Hey everyone who’s been frustrated that VH units in CSS don’t do what you need… can you describe your usecase? What layout are you creating? With which layout mechanism? What do you need? Screenshots & sample code appreciated. — Jen Simmons ( @jensimmons ) May 15, 2021
Have a feeling I’m going to be talking about Environment Variables a lot this week. They are really cool & supported! https://developer.mozilla.org/en-US/docs/Web/CSS/env() https://caniuse.com/css-env-function padding-bottom: calc(1rem + env(safe-area-inset-bottom)); -or- height: calc(100vh - env(safe-area-inset-bottom)); — Jen Simmons ( @jensimmons ) June 7, 2021
- https://bugs.webkit.org/show_bug.cgi?id=141832 ↩
- https://css-tricks.com/the-trick-to-viewport-units-on-mobile/ ↩
- https://github.com/w3c/csswg-drafts/issues/4329 ↩
- https://webkit.org/blog/7929/designing-websites-for-iphone-x/ ↩
iOS 15 Safari: How to change the address/search bar design on iPhone
By Michael Potuck
August 18, 2021
Apple’s latest iOS release comes with an all-new Safari design that places the address/search bar at the bottom of the screen by default. But you can switch back to the classic iOS Safari design with a top bar. Here’s how to change the iOS 15 Safari address/search bar on iPhone.
Apple made its way through multiple iterations of the new iOS 15 Safari design and with the sixth beta , it included the option to switch between the controversial bottom address/search bar and the classic iOS Safari top address/search bar on iPhone.
The latest release also includes the classic bottom menu bar instead of packing all of Safari’s buttons into the unified address/search/tab bar. iOS 15 is currently available as a public and developer beta with the official release set for fall 2021.
How to change iOS 15 Safari address/search bar on iPhone • Running iOS 15, open Safari on your iPhone • In the bottom address/search bar, tap the “aA” icon on the left • Tap Show Top Address Bar To change back to the bottom bar design • Tap the “aA” icon in the top address/search bar • Choose Show Bottom Tab Bar Alternatively, you can also change the iOS 15 address/search bar by heading to the Settings app > Safari > swipe down and choose “Single Tab.”
Here’s how it looks to change the Safari address/search bar on iPhone:
And here’s how it looks to change it back to the bottom address/search bar:
The other way to change the address/search bar design is in the Settings app. In addition to changing between the bottom and classic top address/search bar designs, you can choose to enable/disable website tinting (matches the top iPhone status bar to websites’ colors) and enable/disable the landscape tab bar, however, the latter doesn’t seem to be working at the time of writing in iOS 15 beta 6.
Did you find this guide helpful? Read more 9to5Mac tutorials:
Latest iOS 15 beta fixes the Safari address bar and lets you move it back to the top
A return to a more traditional design.
By Mitchell Clark
Share this story
:format(webp)/cdn.vox-cdn.com/uploads/chorus_asset/file/19713461/acastro_200207_3900_Safari_0001.0.jpg)
Apple has updated Safari’s design in the latest iOS 15 beta (Developer Beta 6), making the address bar look and act more like what users are used to and giving you the option to put it at either the top or bottom of the screen. Safari’s redesign, especially on iPhones, has been controversial since it was first announced , but it seems like Apple is starting to walk back the floating design that’s persisted throughout the beta.
MacStories founder and podcaster Federico Viticci has posted screenshots of the new design on Twitter, showing off the changes. If the user chooses to have the address bar on the bottom, it sits above a row of controls, including back and forward buttons, a share button, and the tab button. (In previous versions of the beta, these buttons were one with the address bar.) If the user chooses the option to have the address bar at the top, Safari will look similar to how it did in iOS 14.
Apple has been tweaking and changing Safari’s new design since it made its debut in the first beta. The updates have been relatively small — buttons were added back into the address bar (making it a minefield of touch targets), and Apple made the address bar easier to get to when you’re typing in a URL or search term . However, the incremental updates haven’t appeased those calling for a return to a more traditional design, one that doesn’t include a floating element that hovers above a webpage’s content.
Options should help please those who (kind of) liked the change, and those who didn’t
The option to change where the address bar resides should please those who thought its new place at the bottom was a good one (regardless of how its actual design was executed), as well as those who prefer to have it at the top. It may make things tough for web designers, who will have to deal with the possibility of something butting up against either the top or bottom of their webpage, but it’s a nice option for users to have.
According to Viticci , the iPad version of Safari hasn’t changed with this update, though Apple introduced an option that more or less let you go back to a traditional design in Beta 4.
EcoFlow’s $200 PowerStream is so clever, you might buy a $4,000 solar generator
Ventje turns vw’s id buzz into a very charming e-camper, icq is shutting down after almost 28 years, google scrambles to manually remove weird ai answers in search, there’s no easy 3d printer, but bambu has won me over.

More from Tech
:format(webp)/cdn.vox-cdn.com/uploads/chorus_asset/file/24365737/Wyze_Cam_OG_Telephoto_8.jpg)
Wyze cameras let some owners see into a stranger’s home — again
:format(webp)/cdn.vox-cdn.com/uploads/chorus_asset/file/24049860/226292_Apple_Watch_SE_PHO_akrales_0047.jpg)
Here are the best Apple Watch deals right now
:format(webp)/cdn.vox-cdn.com/uploads/chorus_asset/file/24247717/lp_logo_3.0.jpg)
OpenAI can’t register ‘GPT’ as a trademark — yet
:format(webp)/cdn.vox-cdn.com/uploads/chorus_asset/file/25184511/111323_PlayStation_Portal_ADiBenedetto_0013.jpg)
Sony’s portable PlayStation Portal is back in stock
How to use the Smart Search bar in Safari on iPhone and iPad
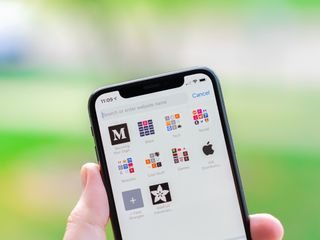
- How to search the web using the Smart Search bar
- How to search bookmarks and web history using the Smart Search bar
How to search the text on a specific web page using the Smart Search bar
How to change your default search browser in safari, how to search safari from search on the home screen in ios 15.
The Smart Search bar in iOS 15 is a hybrid of the old address and search bars melded into one universal place to type and go. You can access your default search provider, your browsing history, saved bookmarks, and even specific words on web pages, all through the unified Smart Search bar at the top of your Safari browsing window. It makes searching for something on your best iPhone more straightforward. Here's how to use the Smart Search bar in Safari on iPhone and iPad.
How to search the web using the Smart Search bar on iPhone and iPad
- Launch Safari from the Home screen.
- Tap in the Smart Search bar at the top or bottom of your browser.
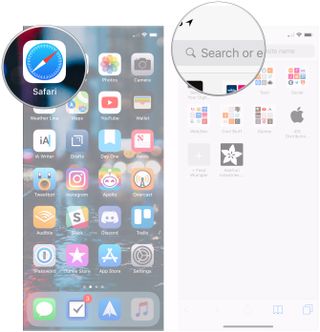
- Type in some keywords or a phrase you would like to search for.
- Tap on the Go button at the bottom right of your screen.
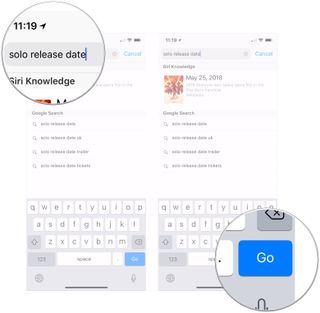
That's it! Safari will now use your default search engine to scour the internet for you.
How to search bookmarks and web history using the Smart Search bar on iPhone and iPad
- Launch Safari from your Home screen.
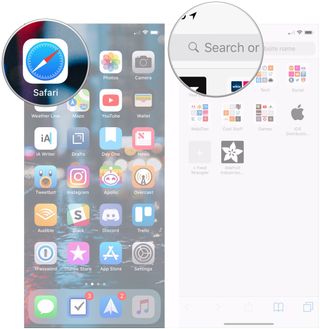
- Type in some keywords you would like to search the internet for (I searched for "apple").
- Tap on the site or bookmark you would like to navigate to under the Bookmarks and History heading.
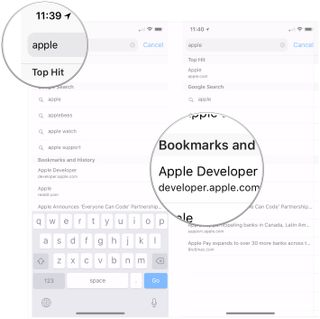
- Open Safari on your iPhone or iPad.
- Open a web page in Safari.
- Tap the Smart Search bar .
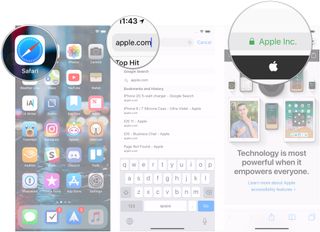
- Type the word or phrase that you want to find on the page.
- Tap the word or phrase under On this Page.
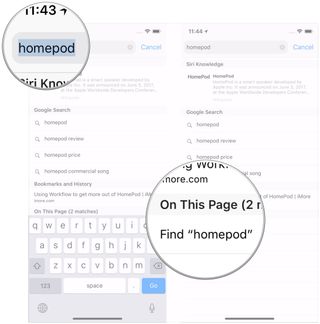
- Tap the navigation buttons to jump to each instance of the word if there's more than one.
- Tap Done when you're finished.
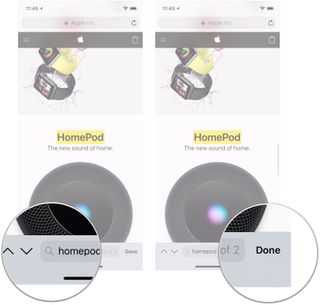
Everyone has their favorite search engine. Make sure yours is programmed as the default and use the Smart Search bar in Safari.
- Tap on Settings from your Home screen.
- Tap on Safari (you may have to scroll down to find it).
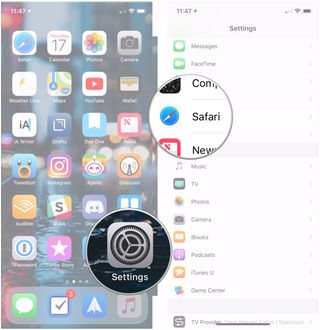
- Tap on Search Engine .
- Tap on your preferred search engine from the list.
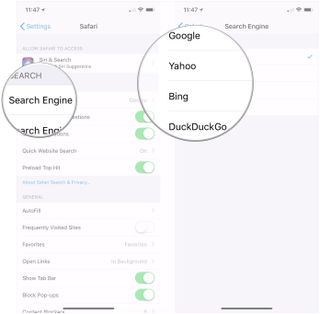
Next time you open up Safari and type something into the Smart Search bar, it will use your newly-set search engine.
In iOS 15 , super-powered the native search functionality available to the left of the Home screen. When you enter a search term in the Search feature, you'll see suggested web searches and even websites based on your term.
- Swipe right on your Home screen to navigate to search.
- Enter your search term in your search bar.
- Tap the search result you want.
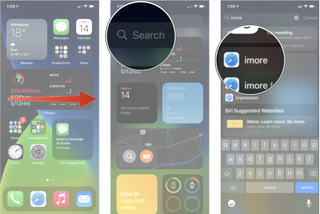
As you can see in the example above, the search terms that iOS found for "iMore" will automatically open Safari and search the web using that term.
The Smart Search bar can be at the top or bottom
If you're on iOS 15, you may notice that Apple switched the search/address bar from the top of the screen in Safari to the bottom of the screen. There's no functionality difference between the two options, but if you prefer the Smart Search bar to be at the top rather than the bottom, you can change the location of the address bar in Settings .
Master your iPhone in minutes
iMore offers spot-on advice and guidance from our team of experts, with decades of Apple device experience to lean on. Learn more with iMore!
Updated March 2022: Updated for the most recent version of iOS 15.
Luke Filipowicz has been a writer at iMore, covering Apple for nearly a decade now. He writes a lot about Apple Watch and iPad but covers the iPhone and Mac as well. He often describes himself as an "Apple user on a budget" and firmly believes that great technology can be affordable if you know where to look. Luke also heads up the iMore Show — a weekly podcast focusing on Apple news, rumors, and products but likes to have some fun along the way.
Luke knows he spends more time on Twitter than he probably should, so feel free to follow him or give him a shout on social media @LukeFilipowicz .
iOS 18 to bring redesigned home screen according to Apple insider
WWDC 2024 to reportedly showcase Project Greymatter, Apple's idea of AI for the masses
Apple still looking to sign deal with Google for Gemini, despite OpenAI announcement likely coming in June - report
Most Popular
- 2 iOS 18 to bring redesigned home screen according to Apple insider
- 3 WWDC 2024 to reportedly showcase Project Greymatter, Apple's idea of AI for the masses
- 4 Feeling distracted? This iPhone app has a cute and effective approach to procrastination
- 5 Apple Vision Pro earns prestigious UK award, even though it hasn't launched there yet
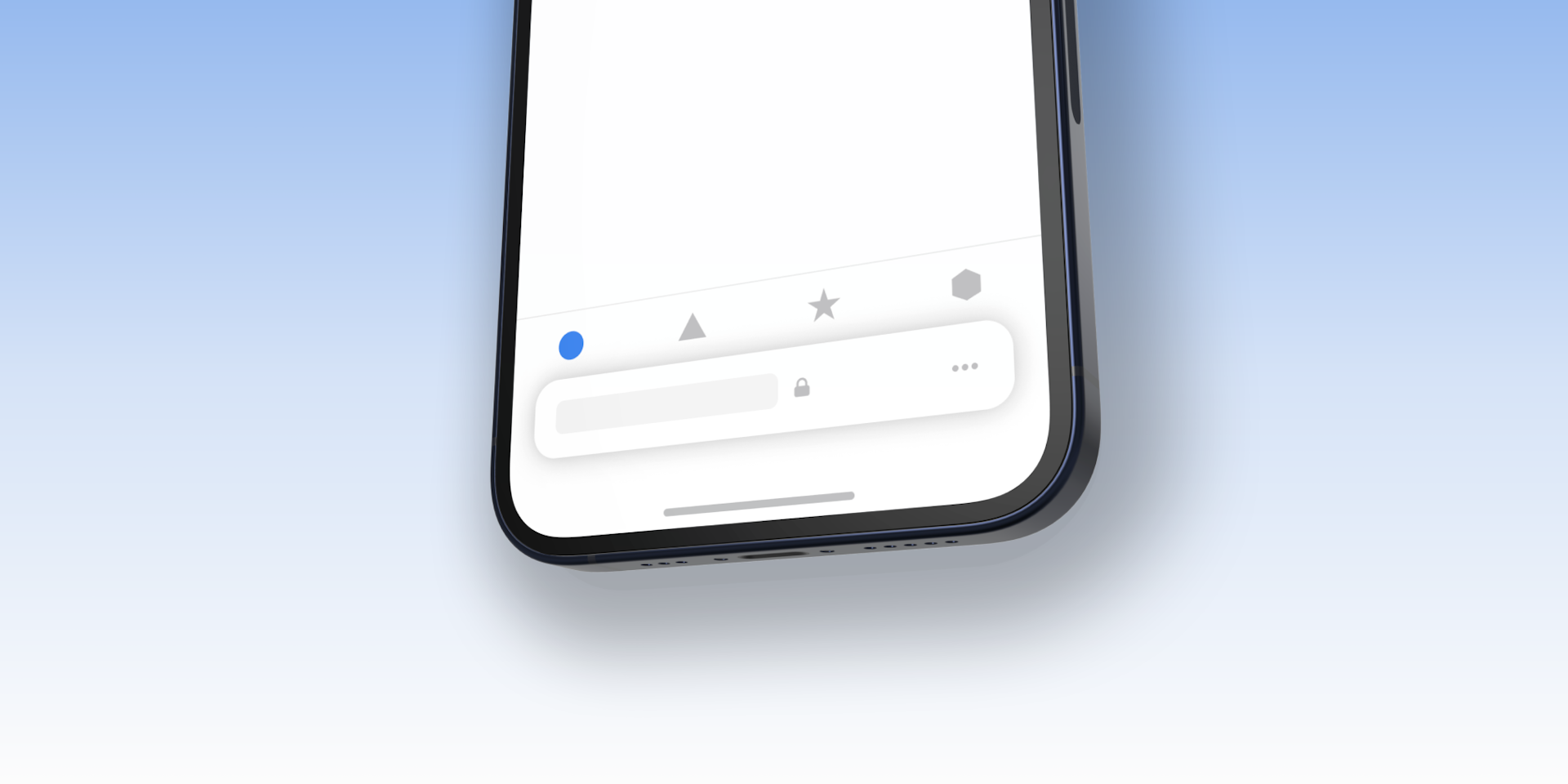
Using Bottom Tab Bars on Safari iOS 15
Jun 17, 2021 (Updated Sep 23, 2021)
Apple recently announced the latest version of Safari on iOS 15 with a completely new design featuring a bottom floating tab bar. What does this mean for web developers and designers?
Safari on iOS has had a problem for a long time when it comes to building websites and web apps with bottom-aligned navigation due to the browser toolbar's dynamic height. As you scroll the toolbar disappears making any element that is fixed to the bottom of the screen look great, but as soon as you try to tap any link inside the browser toolbar appears again.
Sorry, your browser doesn't support embedded videos.
This makes for a really poor UX so designers and developers have mostly resorted to user "hamburger" menus instead. This is a shame as bottom tab bars increase discoverability by not hiding links behind a tap and are also easier to reach one-handed on todays large mobile devices.
Updates with Safari 15
Apple reverted some of these changes in the final iOS 15 release. The user can now choose between the old UI (top bar) or the new one. If they choose the new bottom bar UI it does not float as much greatly improving overlap issues. While you might not need safe areas anymore if you're lucky, I would still recommend to implement it as I've seen it cause issues anyways.
The new Safari 15 now has a tab bar floating at the bottom of the screen. At first it might seem like this makes it even harder to create tab bar navigations, and by browsing the web using iOS 15 it's easy to spot issues like this:
Fixing Tab Bar Overlap with Safe Areas
Thankfully solving this issue is very easy by using the env() CSS function together with safe-area-inset-bottom . This API was shipped with iOS 11 making it possible to customize how websites render when using devices with a notch. By inspecting pinterests code we can see that their tab bar has a fixed position anchored to the bottom, the relevant parts look something like this:
To respect the safe area and make sure that nothing from the browser UI overlaps let's add another bottom property with env(safe-area-inset-bottom) as a value. This function works like a CSS variable, returning the minimum amount of inset needed to keep your UI from overlapping with the browser's. We keep the old style rule as a fallback browsers that do not support it:
Now when scrolling nothing overlaps:
Be sure to use env() every time something is anchored to the bottom of the screen or overlap will likely appear. env() can also be combined with css calc() or min() and max() , so if your design needs more padding you can add it like this:
You can learn more about respecting the safe-area in this excellent article published on the webkit blog or Apple's WWDC session called Design for Safari 15 (Relevent part starts around 13 minutes in).
The best way to see if you got it right is to use a physical device with iOS 15, but if you don't have access to one you can download the Xcode 13 beta from Apples developer portal and use an iOS 15 simulator by going to Xcode > Open Developer Tool > Simulator
Tab Bar UX in iOS 15
Remember the issue in previous versions of Safari where you had to click twice when using bottom tab bars? Once for showing the safari toolbar and another tap for actually triggering your link? That is no longer an issue 🙌. Safari 15 now respects and follows links or buttons, which is a big improvement! Check out how much better Twitter's tabbar works when switching tabs on Safari 15:
Even if tab bars now technically work better than before we still have to consider the design and UX to create something that people understand and that looks good. The browser UI is now very bottom-heavy and placing more actions next to it might feel cluttered. What do you think? Let me know on twitter @samuelkraft .
I'm excited to see how everyone adapts to the new UI and if we will see a return of tab bars on the web or not.
Get an email when i write new posts. Learn animation techniques, CSS, design systems and more
Related posts
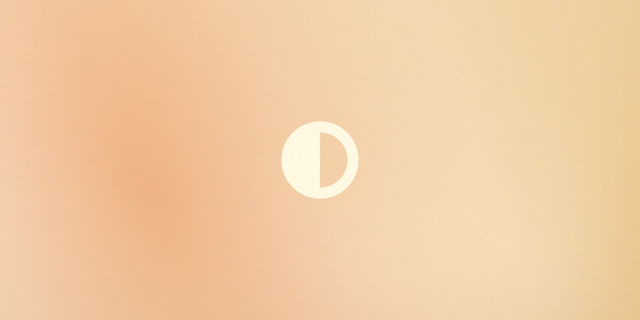
Dec 18, 2021
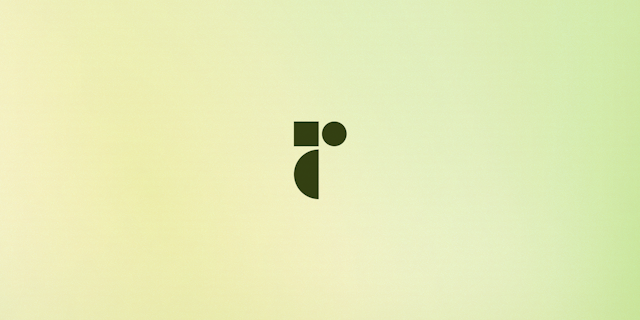
Dec 15, 2021
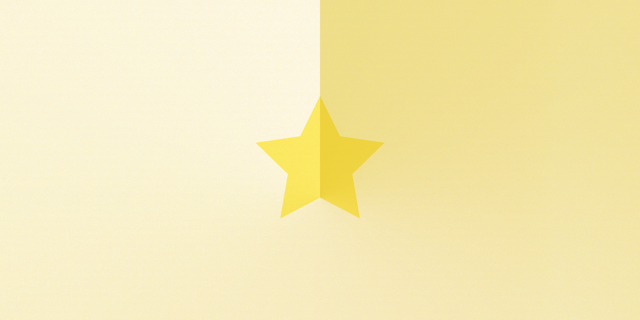
Sep 07, 2021
- a. Send us an email
- b. Anonymous form
- Buyer's Guide
- Upcoming Products
- Tips / Contact Us
- Podcast Instagram Facebook Twitter Mastodon YouTube Notifications RSS Newsletter
How to Set Safari's Address Bar to the Top or Bottom of the Screen
During the beta phase of iOS 15, Apple added a new Safari design element that moves the URL and tab interface to the bottom of the screen, a decision that was immediately controversial with iPhone users.
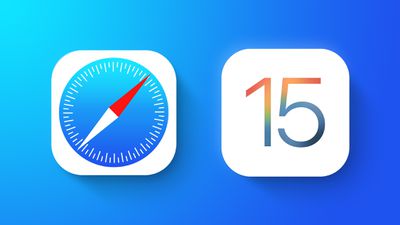
If you can't get on with the address bar living at the bottom of the screen and would prefer to have it in its original position at the top, like it was in iOS 14, follow these steps.
- Launch Safari on your iPhone.
- Tap the " aA " icon in the left side of the address bar.
- Tap Show Top Address Bar in the popup menu.
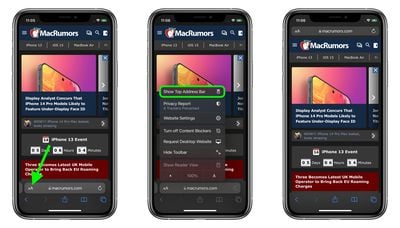
You can also control this design change in Settings -> Safari , under the "Tabs" section. To take the URL bar to the top of the Safari interface, select Single Tab .
Get weekly top MacRumors stories in your inbox.
Popular Stories
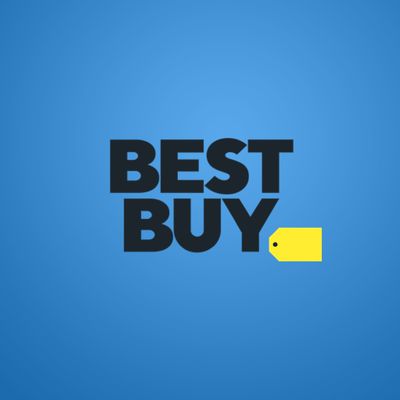
Best Buy's Memorial Day Sale Has Record Low Prices on iPads, MacBooks, and Much More
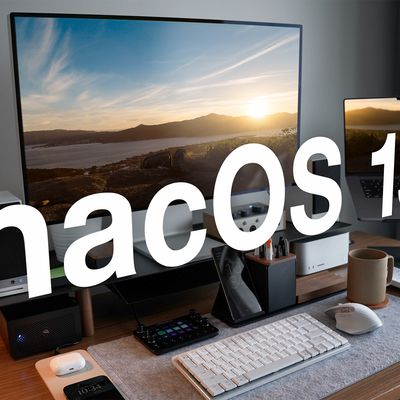
macOS 15 System Settings to Get Design Overhaul
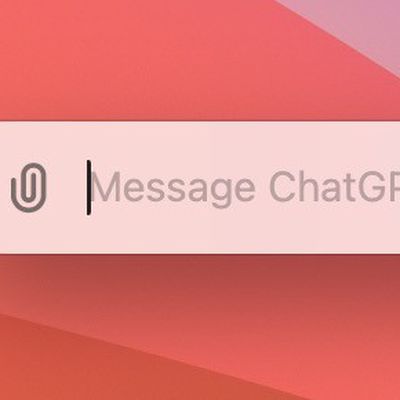
5 Reasons to Use OpenAI's ChatGPT App for Mac
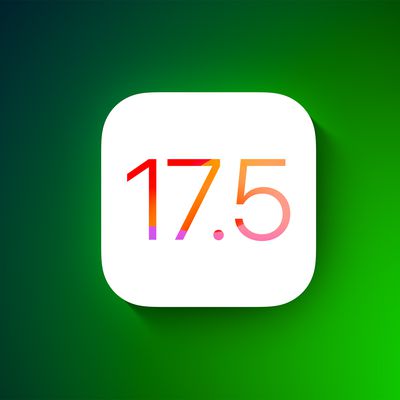
Apple Sheds More Light on iOS 17.5 Bug That Resurfaced Deleted Photos
Next article.
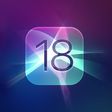
Our comprehensive guide highlighting every major new addition in iOS 17, plus how-tos that walk you through using the new features.
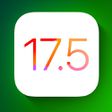
Apple News+ improvements, cross-platform tracker alerts, website app downloads for the EU, and more.
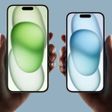
Get the most out your iPhone 15 with our complete guide to all the new features.
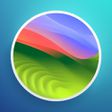
A deep dive into new features in macOS Sonoma, big and small.
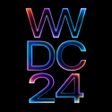
Apple's annual Worldwide Developers Conference will kick off with a keynote on June 10.
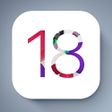
Expected to see new AI-focused features and more. Preview coming at WWDC in June with public release in September.
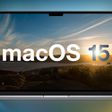
AI-focused improvements, new features for Calculator and Notes, and more.
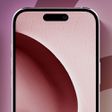
Action button expands to all models, new Capture button, camera improvements, and more.
Other Stories
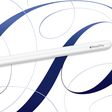
3 days ago by Tim Hardwick
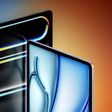
4 days ago by Juli Clover
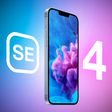
5 days ago by Tim Hardwick
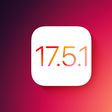
6 days ago by Tim Hardwick
Safari: How to Get Search Bar Back to the Top & Disable Landscape Tab View
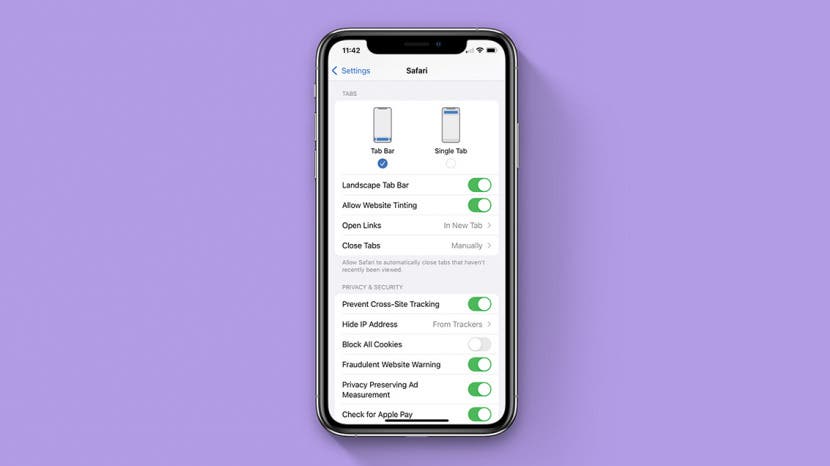
With iOS 15 Safari, the app has a whole new layout which brings many updates to the way you browse in the app. In Safari, the address bar is now located at the bottom of your iPhone screen, and in landscape orientation tabs will now appear in a list across the top. If you find these updates inconvenient and want to revert to the previous iOS 14 Safari layout, we'll show you how!
Related: How to Hide Apps on the iPhone & Find Them Later
Move Address Bar to the Top of Safari
Remove the tab bar from safari landscape view.
Moving the search bar to the bottom of the screen has been one of the biggest changes to Safari in iOS 15. Luckily, if you don't like this change, you can switch it back! Safari now has two tab modes: Single Tab and Tab Bar. With Single Tab mode, your Apple address bar is displayed at the top of Safari and you must tap the tab icon to switch between tabs. With the Tab Bar mode, your URL address bar is displayed at the bottom, and you can swipe between open Safari tabs. Below, we'll cover how to get the URL bar back to the top of the page in Safari.
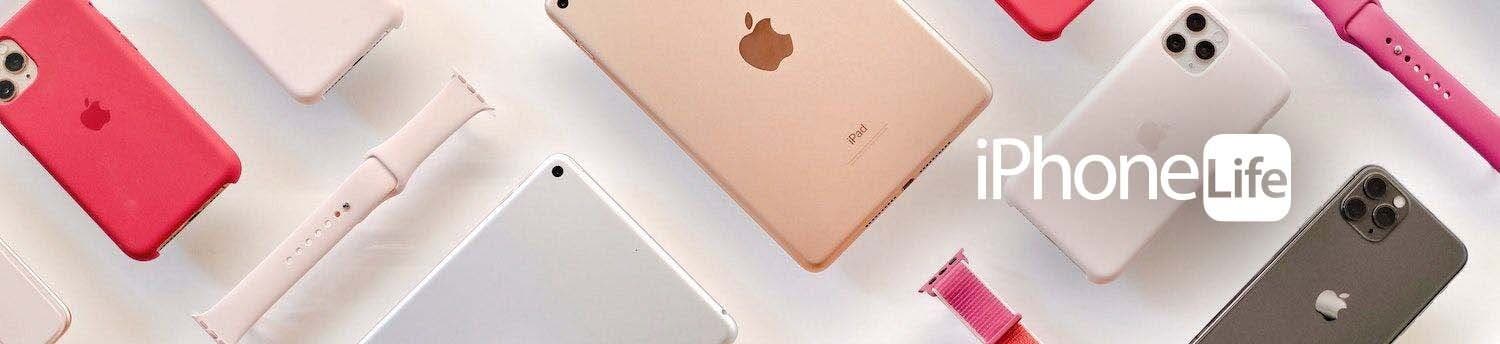

How to Move Address Bar to the Top in Safari (Shortcut)
Perhaps you find yourself accidentally swiping between tabs or you find it disorienting to have the URL bar at the bottom of your screen. Whatever your reason, this is the fastest way to switch into Single Tab mode and move the search bar to the top of Safari while browsing.
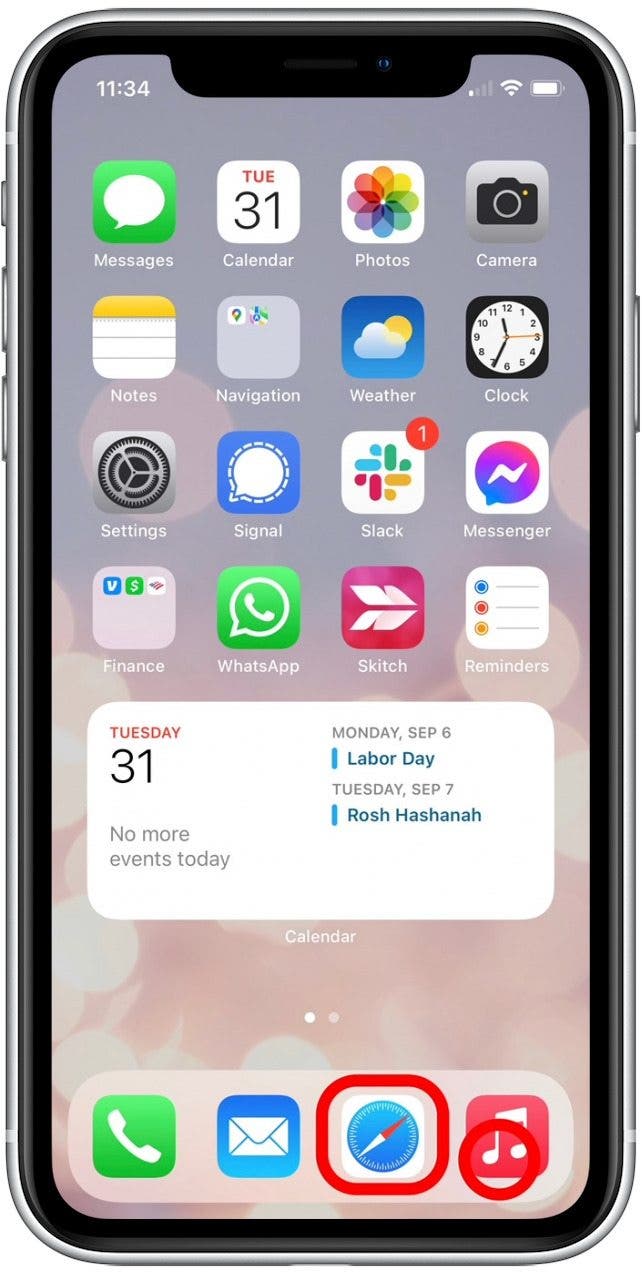
Now your Apple search bar will be displayed at the top of the screen. If you ever change your mind and want to revert back to the Tab Bar mode (being able to swipe between tabs is pretty handy!), follow the steps above and tap Show Bottom Tab Bar.
How to Place the Website Search Bar at the Top in Settings
This is a different method to adjust the same setting shown in the shortcut above. Below, we'll cover how to move your iPhone or iPad search bar to the top in Settings.
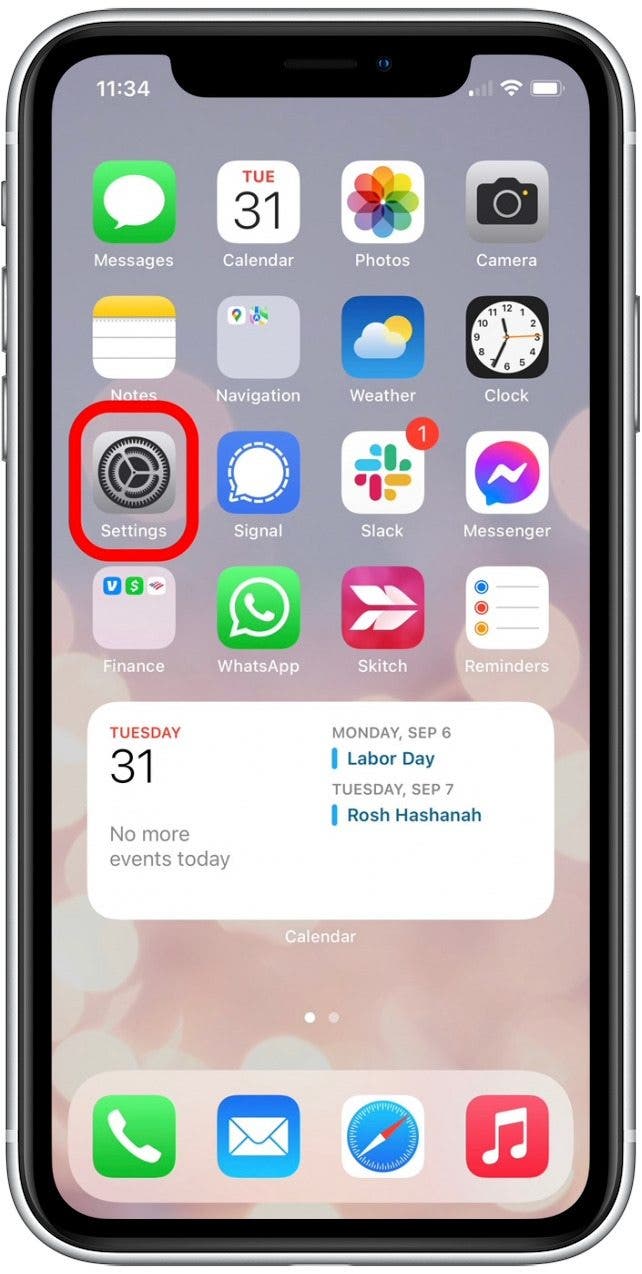
The landscape tab bar in Safari displays open tabs across the top of your screen and allows you to tap and switch between them, much the same way you would on your laptop. This is a handy update, as it means you no longer have to tap the Tab icon to switch tabs while browsing in landscape view. However, if you feel the landscape tab bar is crowding your screen and cramping your style, you can disable this feature:
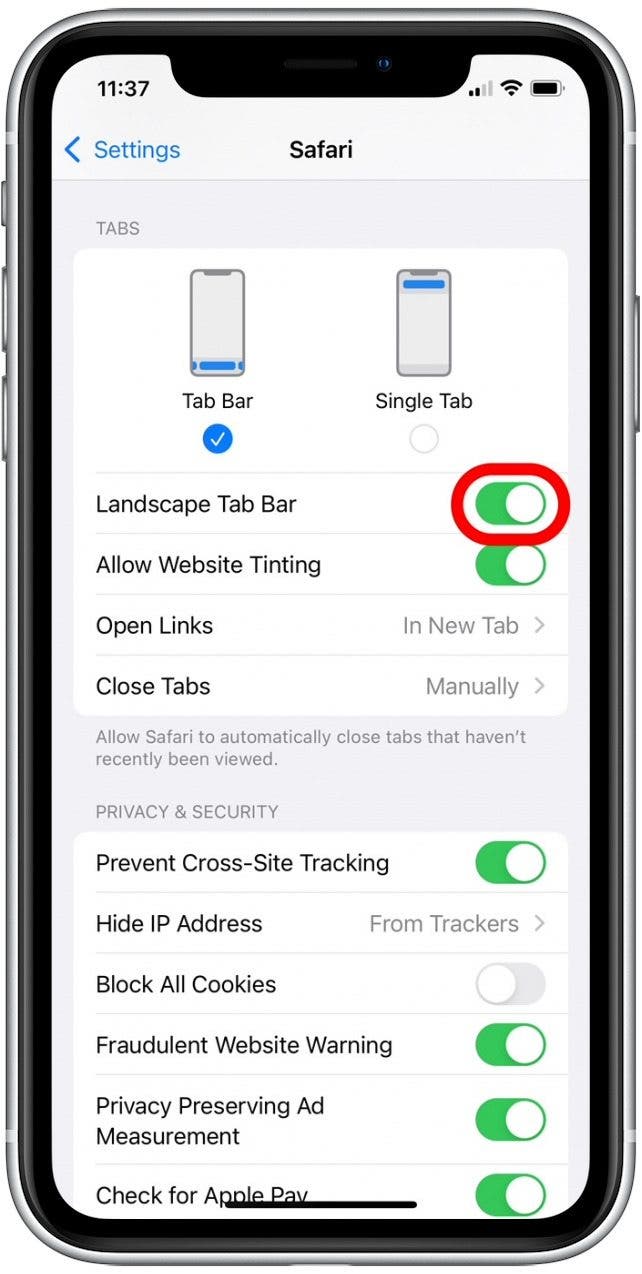
That's all there is to it! Once you've adjusted these iOS 15 Safari settings, you'll be back to the previous browsing layout you know and love. You can also learn how to customize the Safari Start page to show the information you need.
Author Details
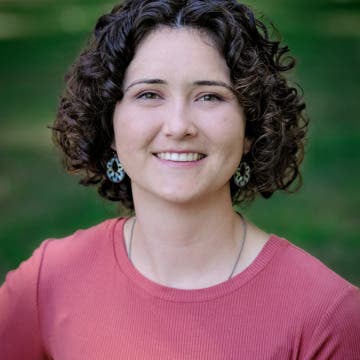
Ashleigh Page
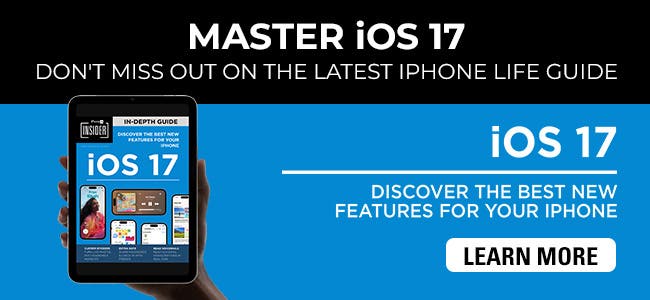
Article Spotlight
New podcast episode: all about apple's latest ipad drop.
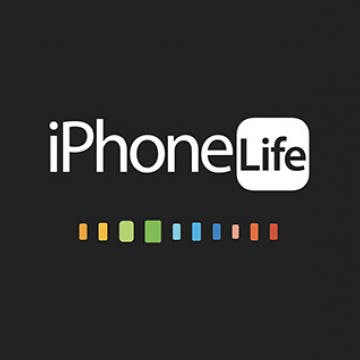
In the 211th episode, David and Donna cover all the new iPads that Apple announced at its online 'Let Loose' event on May 7. From the AI-focused M4 chip to a haptic touch-enhanced Apple Pencil Pro, listen in to find out if you should buy one of the new supercharged iPads!
Featured Products
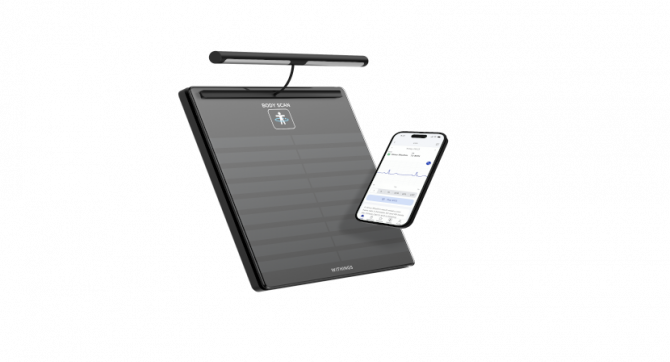
Get your ultra-precise body assessment with the new Body Scan from Withings. The Body Scan from Withings offers a revolutionary body assessment directly from your home in just 90 seconds. Through a simple weigh-in, the Body Scan helps you reach your weight goals quicker and build muscle mass faster. It can even detect cardiac anomalies. See Withings deals!
Most Popular
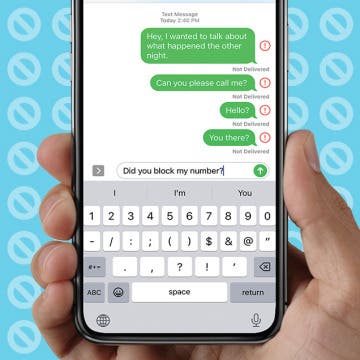
How to Tell If Someone Blocked Your Number on iPhone
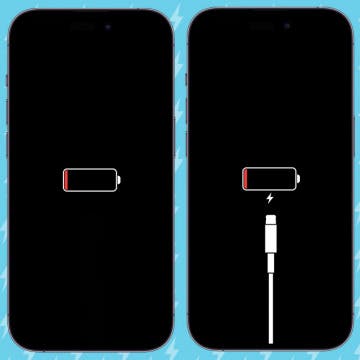
How to Tell If a Dead iPhone Is Charging
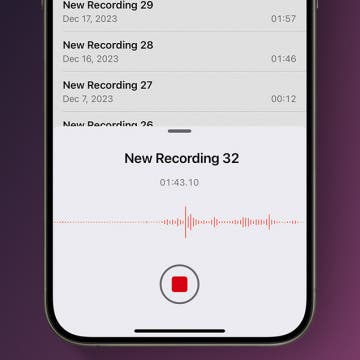
How to Record a Phone Call on an iPhone for Free
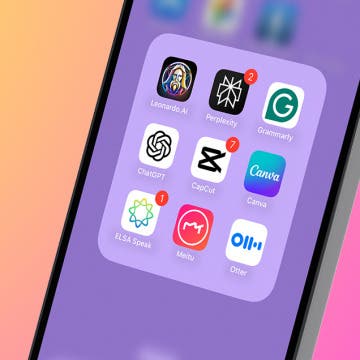
10 Best AI Apps for iPhone in 2024
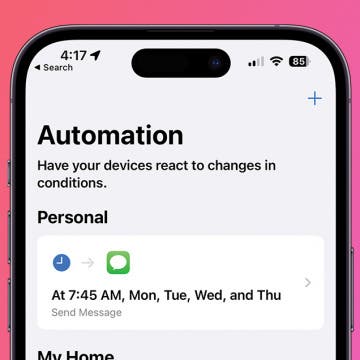
How to Schedule a Text Message on iPhone
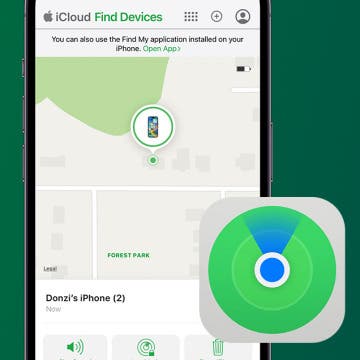
How To Find My iPhone From Another iPhone
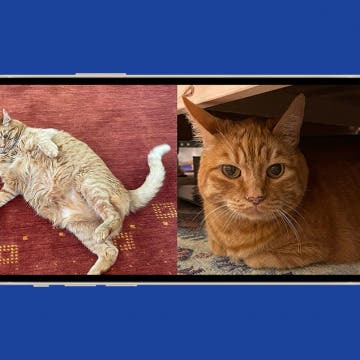
How To Put Two Pictures Together on iPhone
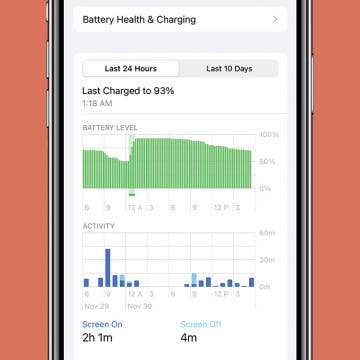
10 Simple Tips To Fix iPhone Battery Drain
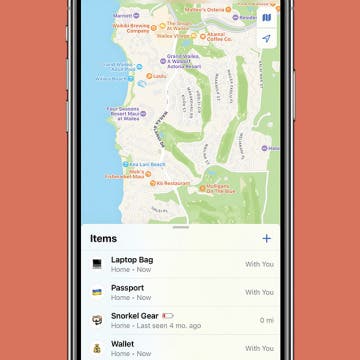
How to Refresh AirTag Location Manually & More Often
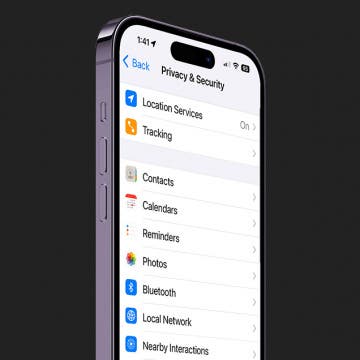
Can iPhones Get Viruses? How to Detect & Remove Malware (iOS 17)
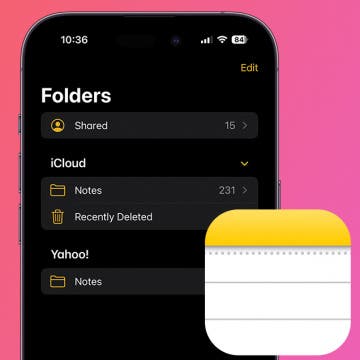
iPhone Notes Disappeared? Recover the App & Lost Notes
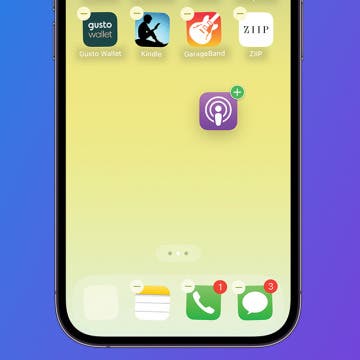
How To Get an App Back on Your Home Screen
Featured articles, why is my iphone battery draining so fast 13 easy fixes.
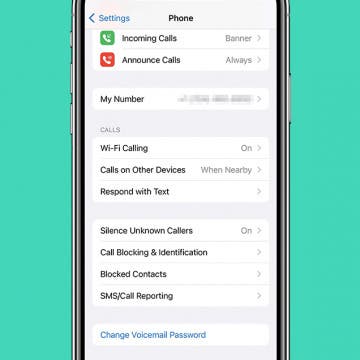
Identify Mystery Numbers: How to Find No Caller ID on iPhone
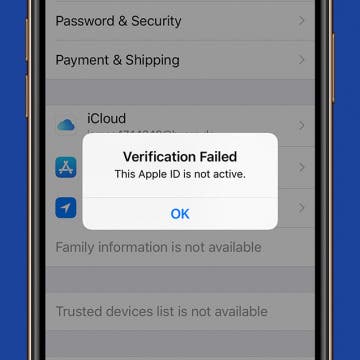
Apple ID Not Active? Here’s the Fix!
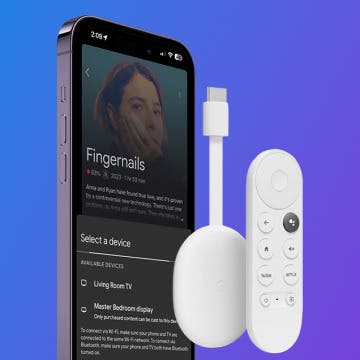
How to Cast Apple TV to Chromecast for Easy Viewing
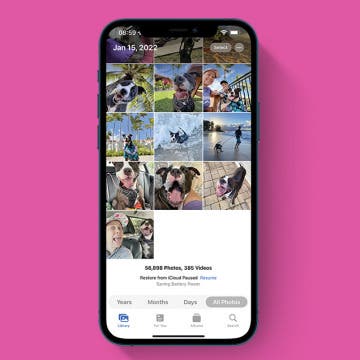
Fix Photos Not Uploading to iCloud Once & for All (iOS 17)
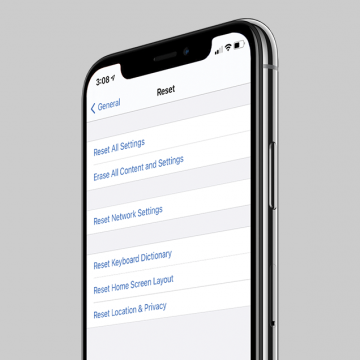
There Was an Error Connecting to the Apple ID Server: Fixed
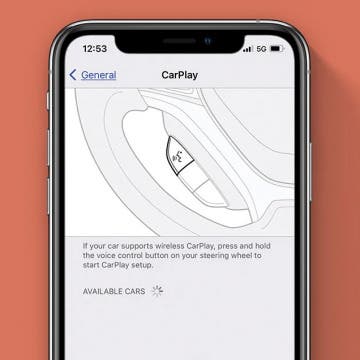
CarPlay Not Working? 4 Ways to Fix Apple CarPlay Today
Check out our sponsors.
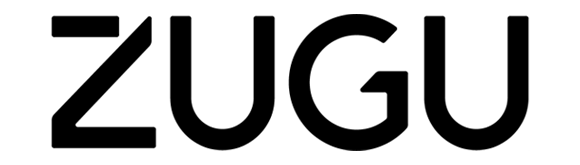
- Each email reveals new things you can do with your phone (and other devices) with easy-to-follow screenshots.
- Enter your email to get your first tip immediately!
This iPhone Setting Will Make It Easy To Switch Between Pages On Safari
E ven though Safari is Apple's official browser app for its devices, you can actually change your iPhone's default web browser to a third-party option. If you do, though, you may miss out on some of the features and upgrades Apple's made to Safari over the last few years. For instance, in 2022 with iOS 16, Apple added passkeys, a more secure way of logging in to apps and service while browsing the web than standard passwords. Meanwhile, those who've recently upgraded to last year's iOS 17 can enjoy an overall faster search that provides more relevant related suggestions. The company also added a feature to lock private browsing tabs between uses.
With the imminent release of iOS 18 this fall, it can be easy to overlook iPhone features that came out long ago. For Safari, there are little known features like the ability to create tab groups. Apple's also added features to change the location of the Safari address bar, a setting that initially irked iPhone users . This setting though actually unlocks a pretty useful and seemingly underutilized feature that makes it easy to page-hop on the browser app.
Read more: 12 Smart Gadgets You Didn't Know Existed
How To Change The Tab Bar Location
Since Apple released iOS 15 in 2021, iPhone users have had the option to pick between two address bar placements for the mobile browser's interface: the original that places the search field at the top of the screen, or a newer "tab bar" layout that places it at the bottom of the screen instead. Here's how you can make modifications to how Safari looks like on your iPhone:
- Launch the Settings app.
- Scroll down and go to Safari.
- Under the Tabs section, select Single Tab to place the address bar up top. Otherwise, pick Tab Bar to have the address bar placed at the bottom.
The top tab bar option is likely what a lot of mobile browser users are accustomed to as top-of-page is often the default placement of address bars across a variety of browser apps, including those used on computers. If you want more flexibility in your use of the browser app on your iPhone though, there is merit to picking the lesser known page layout instead. Not only is it more thumb-friendly, but it adds certain browsing features that are only accessible when you do so.
Surfing Safari's Tab Bar Layout
If you opt for the bottom Tab Bar view in the Settings app, you can easily switch from one web page to another by swiping left or right on the address bar at the bottom of the screen. If you have several browser tabs open at once, you can essentially flip through them, as if you're reading a book. Meanwhile, if you choose to stick with Safari's Single Tab layout, you can only view one page at a time. You'll also need to click on the Tabs switcher icon to see all your open pages or any tab groups that you may have.
Based on testing, embracing the unconventional bottom-of-the-screen address bar placement has a few perks. It makes it more convenient to use Safari and operate your iPhone one-handed . You can quickly type in new searches or websites into the search field since it's closer to the thumb of the hand holding your device. It's also easier to scroll through your tab group carousel — that's also located at the bottom of the iPhone screen — when you hit the Tab switcher icon to view all your open tabs.
Read the original article on SlashGear
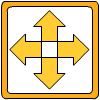
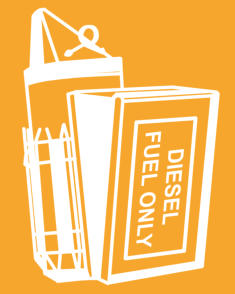
Image Unavailable

- To view this video download Flash Player

XL Flag Elektrostal Moscow oblast | landscape flag | 2.16m² | 23sqft | 120x180cm | 4x6ft - 100% Made in Germany - long lasting outdoor flag
Purchase options and add-ons, about this item.
- 100% Made in Germany » ... because the first impression last, quality flag for representative purposes *****
- State-of-the-art High-Tech Outdoor Fabric » One air-permeable 110 GSM Polyester to keep wind forces low and lifetime high
- Mirrored Back » Image printed on the front, mirrored image 100% visible on the rear side
- Landscape flag | 2.16m² | 23sqft | 120x180cm | 4x6ft
- Show your pride for your hometown with the Elektrostal flag! Made with quality materials and vibrant colors, this flag is the perfect way to display your patriotism and love for your city. Fly it proudly at home, at events, or even in your car. Get yours today and show your Elektrostal pride!
- The flag of Elektrostal, Moscow Oblast, is a striking combination of Old Glory red, representing strength and courage at 81%, complemented by a subtle touch of light grey at 5% for balance and harmony. The bold black stripe at 3% adds a touch of sophistication, while the shimmering gold stripes at 3% each symbolize prosperity and success. The flag is completed with a touch of very dark grey at 1%, representing the city s resilience and
- Elektrostal Moscow oblast
Product information
Warranty & support, looking for specific info, product description.
Flag: Elektrostal Moscow oblast landscape flag | 2.16m² | 23sqft | 120x180cm | 4x6ft Elektrostal Moscow oblast Elektrostal obwód moskiewski , flaga ???????????? ?????????? ??????? Since we know how important your external presentation is, we print our Elektrostal Moscow oblast flag for your representative appearance using the most modern machines in Germany. To ensure your maximum flexibility, we have equipped the flags with quality metal eyelets, to let you simply attach these flags to any flagpole. To let you use the flags for a long time, we have strengthened the flag using double safety seams and a tear proof strap at the side of the pole. Due to the quality of this business flag, you show a particular degree of the closeness to Elektrostal Moscow oblast. Details about this flag This landscape Elektrostal Moscow oblast flag is a quality product Made in Germany made of 110g/m² gloss polyester. This Elektrostal Moscow oblast flag is wind- and weather-resistant and highly durable. The flag colors are intensive and UV-resistant. This flag is specially made for outer space. This Elektrostal Moscow oblast flag will be delivered with a double safety-seam as well as with 2 metal eyelets to hoist at the flag pole. The metal eyelets give you great flexibility for placing this flag on any flagstaff. The mast side is reinforced with a white hem. The quality flag material and the metal eyelets will take care of a long endurance of this Elektrostal Moscow oblast flag. If required, the flag can be washed at 60 degrees Celsius. Recommended height of flag pole Elektrostal Moscow oblast flags of 2.16m² | 23sqft | 120x180cm | 4x6ft look best with flagpoles of around 6m | 18ft height. Need a bigger size or an other configuration? We can provide bigger sizes, other configurations, exclusive indoor ...
Customer reviews
Customer Reviews, including Product Star Ratings help customers to learn more about the product and decide whether it is the right product for them.
To calculate the overall star rating and percentage breakdown by star, we don’t use a simple average. Instead, our system considers things like how recent a review is and if the reviewer bought the item on Amazon. It also analyzed reviews to verify trustworthiness.
No customer reviews
- Amazon Newsletter
- About Amazon
- Accessibility
- Sustainability
- Press Center
- Investor Relations
- Amazon Devices
- Amazon Science
- Sell on Amazon
- Sell apps on Amazon
- Supply to Amazon
- Protect & Build Your Brand
- Become an Affiliate
- Become a Delivery Driver
- Start a Package Delivery Business
- Advertise Your Products
- Self-Publish with Us
- Become an Amazon Hub Partner
- › See More Ways to Make Money
- Amazon Visa
- Amazon Store Card
- Amazon Secured Card
- Amazon Business Card
- Shop with Points
- Credit Card Marketplace
- Reload Your Balance
- Amazon Currency Converter
- Your Account
- Your Orders
- Shipping Rates & Policies
- Amazon Prime
- Returns & Replacements
- Manage Your Content and Devices
- Recalls and Product Safety Alerts
- Conditions of Use
- Privacy Notice
- Consumer Health Data Privacy Disclosure
- Your Ads Privacy Choices
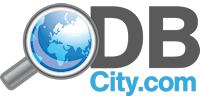
- Bahasa Indonesia
- Eastern Europe
- Moscow Oblast
Elektrostal
Elektrostal Localisation : Country Russia , Oblast Moscow Oblast . Available Information : Geographical coordinates , Population, Area, Altitude, Weather and Hotel . Nearby cities and villages : Noginsk , Pavlovsky Posad and Staraya Kupavna .
Information
Find all the information of Elektrostal or click on the section of your choice in the left menu.
- Update data
Elektrostal Demography
Information on the people and the population of Elektrostal.
Elektrostal Geography
Geographic Information regarding City of Elektrostal .
Elektrostal Distance
Distance (in kilometers) between Elektrostal and the biggest cities of Russia.
Elektrostal Map
Locate simply the city of Elektrostal through the card, map and satellite image of the city.
Elektrostal Nearby cities and villages
Elektrostal weather.
Weather forecast for the next coming days and current time of Elektrostal.
Elektrostal Sunrise and sunset
Find below the times of sunrise and sunset calculated 7 days to Elektrostal.
Elektrostal Hotel
Our team has selected for you a list of hotel in Elektrostal classified by value for money. Book your hotel room at the best price.
Elektrostal Nearby
Below is a list of activities and point of interest in Elektrostal and its surroundings.
Elektrostal Page
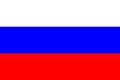
- Information /Russian-Federation--Moscow-Oblast--Elektrostal#info
- Demography /Russian-Federation--Moscow-Oblast--Elektrostal#demo
- Geography /Russian-Federation--Moscow-Oblast--Elektrostal#geo
- Distance /Russian-Federation--Moscow-Oblast--Elektrostal#dist1
- Map /Russian-Federation--Moscow-Oblast--Elektrostal#map
- Nearby cities and villages /Russian-Federation--Moscow-Oblast--Elektrostal#dist2
- Weather /Russian-Federation--Moscow-Oblast--Elektrostal#weather
- Sunrise and sunset /Russian-Federation--Moscow-Oblast--Elektrostal#sun
- Hotel /Russian-Federation--Moscow-Oblast--Elektrostal#hotel
- Nearby /Russian-Federation--Moscow-Oblast--Elektrostal#around
- Page /Russian-Federation--Moscow-Oblast--Elektrostal#page
- Terms of Use
- Copyright © 2024 DB-City - All rights reserved
- Change Ad Consent Do not sell my data
Served by the Norwegian Meteorological Institute and NRK
Elektrostal
Current conditions, weather forecast for the next 10 days, monday 27 may, tuesday 28 may, wednesday 29 may, thursday 30 may, friday 31 may, saturday 1 june, sunday 2 june, monday 3 june, tuesday 4 june, wednesday 5 june.

IMAGES
VIDEO
COMMENTS
Switch to 'Single Tab mode' (address bar at top) in Safari settings. Scroll the page up and down to make the address bar show and hide. Notice the box will have bottom padding only when the home screen indicator is visible (the white bar at the bottom of the screen). answered Sep 9, 2021 at 0:54. Simon_Weaver.
Set the CSS height of your root container element (let's call it rootElement) to the height of the view port:.root-element { height: 100vh; } Then, when the page renders, run this code to update rootElement height to the viewport height minus the size of the browser UI bars (for example, on iOS Safari: top address bar, bottom navigation bar…):
Safari for iOS was one of the first mobile browsers to update their implementation by choosing to define a fixed value for the vh based on the maximum height of the screen. By doing so, the user would not experience jumps on the page once the address bar went out of view. Chrome's mobile browser followed suit around a year ago.
If you're viewing such a layout in Safari on an iOS device, that 100vh element fills up the viewport, but its bottom portion is then covered by a toolbar that includes the next/previous navigation and other controls. (See Figure A.) Note: Although I'm focusing on iOS Safari, this issue also occurs in iOS Chrome. It doesn't occur in other ...
Well in Safari 14, safe-area-inset-bottom is 0px whether the UI chrome is active or inactive, which is something that has annoyed me for a while. safe-area-inset-bottom is 0px when the UI chrome is inactive in Safari 15 on iOS, and then the height of the collapsed chrome minus the height of the expanded chrome when the bar is expanded.
Running iOS 15, open Safari on your iPhone. In the bottom address/search bar, tap the "aA" icon on the left (when on a website) Tap Show Top Address Bar. To change back to the bottom bar ...
Apple's latest iOS release comes with an all-new Safari design that places the address/search bar at the bottom of the screen by default. But you can switch back to the classic iOS Safari design ...
Aug 17, 2021, 12:27 PM PDT. Illustration by Alex Castro / The Verge. Apple has updated Safari's design in the latest iOS 15 beta (Developer Beta 6), making the address bar look and act more like ...
Launch Safari from the Home screen. Tap in the Smart Search bar at the top or bottom of your browser. Navigating to Search bar in Safari on iPhone: Open Safari, tap Smart Search button (Image credit: iMore) Type in some keywords or a phrase you would like to search for. Tap on the Go button at the bottom right of your screen.
Apple's Safari app has undergone a number of changes during the iOS 15 beta testing process. Apple started out with a radical new design that moved the address bar from the top of the app's ...
Safari on iOS has had a problem for a long time when it comes to building websites and web apps with bottom-aligned navigation due to the browser toolbar's dynamic height. As you scroll the toolbar disappears making any element that is fixed to the bottom of the screen look great, but as soon as you try to tap any link inside the browser ...
iOS, iPadOS. You can display a search field in a navigation bar or within your content area. When you use system-provided components to include a search field in a navigation bar, it automatically receives the appropriate appearance and behaves as people expect. For example, the search bar can hide until people swipe down to reveal it ...
Open your iPhone's Settings. Step 2: Step 2. Scroll down to "Safari" and tap it. Step 3: Step 3. Under "Tabs," switch "Tab Bar" to "Single Tab." Up until iOS 15, Safari's search and address bar ...
Launch Safari on your iPhone. Tap the " aA " icon in the left side of the address bar. Tap Show Top Address Bar in the popup menu. You can also control this design change in Settings ...
Open Safari and navigate to a web page. Tap the 'aA' icon in the address bar. Tap Show Top Address Bar . Now your Apple search bar will be displayed at the top of the screen. If you ever change your mind and want to revert back to the Tab Bar mode (being able to swipe between tabs is pretty handy!), follow the steps above and tap Show Bottom ...
Recommended by Our Editors. Open Settings and scroll down to Safari. You'll see two icons. Tap the one on the right called Single Tab to put the address bar back at the top of Safari. The icons ...
Here's how you can make modifications to how Safari looks like on your iPhone: Launch the Settings app. Scroll down and go to Safari. Under the Tabs section, select Single Tab to place the address ...
Welcome to the 628DirtRooster website where you can find video links to Randy McCaffrey's (AKA DirtRooster) YouTube videos, community support and other resources for the Hobby Beekeepers and the official 628DirtRooster online store where you can find 628DirtRooster hats and shirts, local Mississippi honey and whole lot more!
Amazon.com : magFlags XL Flag Elektrostal Moscow oblast | landscape flag | 2.16m² | 23sqft | 120x180cm | 4x6ft - 100% Made in Germany - long lasting outdoor flag : Outdoor Flags : Patio, Lawn & Garden
Elektrostal Geography. Geographic Information regarding City of Elektrostal. Elektrostal Geographical coordinates. Latitude: 55.8, Longitude: 38.45. 55° 48′ 0″ North, 38° 27′ 0″ East. Elektrostal Area. 4,951 hectares. 49.51 km² (19.12 sq mi) Elektrostal Altitude.
How to set full height in web for safari, look like has some extra safe area from safari (ios) when search bar is hiding. can't fully scroll the page. here is the demo: html. ios. reactjs. safari. flutter-web. edited Apr 29, 2022 at 1:50. asked Apr 26, 2022 at 2:07. Darmawan Z.
Monday 3 June. 24° / 17°. 0 mm. 5 m/s. Open hourly forecast. Updated 10:26. Forecast as PDF Forecast as SVG. Weather forecast for Elektrostal for the next 10 days.