Tutorial: Tour of Heroes
The Tour of Heroes tutorial takes you through the steps of creating an Angular application in TypeScript.
The grand plan for this tutorial is to build an app that helps a staffing agency manage its stable of heroes.
The Tour of Heroes app covers the core fundamentals of Angular. You'll build a basic app that has many of the features you'd expect to find in a full-blown, data-driven app: acquiring and displaying a list of heroes, editing a selected hero's detail, and navigating among different views of heroic data.
You'll use built-in directives to show and hide elements and display lists of hero data. You'll create components to display hero details and show an array of heroes. You'll use one-way data binding for read-only data. You'll add editable fields to update a model with two-way data binding. You'll bind component methods to user events, like keystrokes and clicks. You'll enable users to select a hero from a master list and edit that hero in the details view. You'll format data with pipes. You'll create a shared service to assemble the heroes. And you'll use routing to navigate among different views and their components.
You'll learn enough core Angular to get started and gain confidence that Angular can do whatever you need it to do. You'll cover a lot of ground at an introductory level, and you'll find many links to pages with greater depth.

The end game
Here's a visual idea of where this tutorial leads, beginning with the "Dashboard" view and the most heroic heroes:
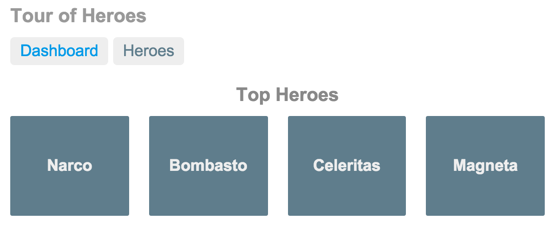
You can click the two links above the dashboard ("Dashboard" and "Heroes") to navigate between this Dashboard view and a Heroes view.
If you click the dashboard hero "Magneta," the router opens a "Hero Details" view where you can change the hero's name.
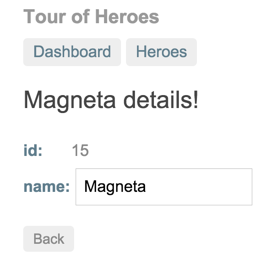
Clicking the "Back" button returns you to the Dashboard. Links at the top take you to either of the main views. If you click "Heroes," the app displays the "Heroes" master list view.
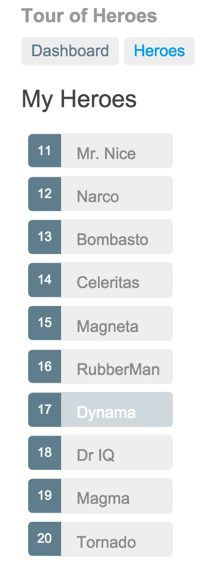
When you click a different hero name, the read-only mini detail beneath the list reflects the new choice.
You can click the "View Details" button to drill into the editable details of the selected hero.
The following diagram captures all of the navigation options.
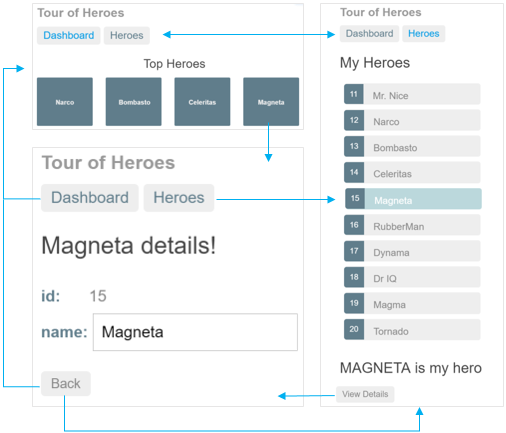
Here's the app in action:
You'll build the Tour of Heroes app, step by step. Each step is motivated with a requirement that you've likely met in many applications. Everything has a reason.
Along the way, you'll become familiar with many of the core fundamentals of Angular.
Tour of Heroes application and tutorial
In this tutorial, you build your own Angular application from the start. This is a good way to experience a typical development process as you learn Angular application-design concepts, tools, and terminology.
If you're new to Angular, try the Try it now quick-start application first. Try it now is based on a ready-made partially completed project. You can edit the application in StackBlitz and see the results in real time.
Try it now covers the same major topics —components, template syntax, routing, services, and accessing data using HTTP— in a condensed format, following best practices.
This Tour of Heroes tutorial provides an introduction to the fundamentals of Angular and shows you how to:
- Set up your local Angular development environment.
- Use the Angular CLI to develop an application.
The Tour of Heroes application that you build helps a staffing agency manage its stable of heroes. The application has many of the features that you'd expect to find in any data-driven application.
The finished application:
- Gets a list of heroes
- Displays the heroes in a list
- Edits a selected hero's details
- Navigates between different views of heroic data
This tutorial helps you gain confidence that Angular can do whatever you need it to do by showing you how to:
- Use Angular directives to show and hide elements and display lists of hero data.
- Create Angular components to display hero details and show an array of heroes.
- Use one-way data binding for read-only data.
- Add editable fields to update a model with two-way data binding.
- Bind component methods to user events, like keystrokes and clicks.
- Enable users to select a hero from a list and edit that hero in the details view.
- Format data with pipes .
- Create a shared service to assemble the heroes.
- Use routing to navigate among different views and their components.
After you complete all tutorial steps, the final application looks like this example.
Design your new application
Here's an image of where this tutorial leads, showing the Dashboard view and the most heroic heroes:
You can click the Dashboard and Heroes links in the dashboard to navigate between the views.
If you click the dashboard hero "Magneta," the router opens a "Hero Details" view where you can change the hero's name.
Clicking the "Back" button returns you to the Dashboard. Links at the top take you to either of the main views. If you click "Heroes," the application displays the "Heroes" list view.
When you click a different hero name, the read-only mini detail beneath the list reflects the new choice.
You can click the "View Details" button to drill into the editable details of the selected hero.
The following diagram illustrates the navigation options.
Here's the application in action:
© 2010–2023 Google, Inc. Licensed under the Creative Commons Attribution License 4.0. https://angular.io/tutorial/tour-of-heroes

deep dive into code
Angular Tutorial - Tour of Heroes - Part 5 (Add services)
Introduction, why services, @injectable() services, get hero data, provide the heroservice, inject the heroservice, add getheroes(), call it in ngoninit(), observable heroservice, subscribe in heroescomponent, create messagescomponent, create the messageservice, inject it into the heroservice, send a message from heroservice, display the message from heroservice, bind to the messageservice, style the messages, add messageservice to heroescomponent.
This tutorial recaps the official documentation at https://angular.io/tutorial/tour-of-heroes/toh-pt4 .
The Tour of Heroes HeroesComponent is getting and displaying fake data. Refactoring the HeroesComponent focuses on supporting the view and making it easier to unit-test with a mock service.
Components shouldn’t fetch or save data directly and they certainly shouldn’t knowingly present fake data. They should focus on presenting data and delegate data access to a service.
This tutorial creates a HeroService that all application classes can use to get heroes. Instead of creating that service with the new keyword (see https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Operators/new ), use the dependency injection that Angular supports (see https://angular.io/guide/dependency-injection ) to inject it into the HeroesComponent constructor.
Services are a great way to share information among classes that don't know each other . Create a MessageService next and inject it in these two places.
- Inject in HeroService , which uses the service to send a message
- Inject in MessagesComponent , which displays that message, and also displays the ID when the user clicks a hero
Create the HeroService
Run ng generate to create a service called hero .
The command generates a skeleton HeroService class in src/app/hero.service.ts (and a test class) as follows:
see https://angular.io/api/core/Injectable
Notice that the new service imports the Angular Injectable symbol and annotates the class with the @Injectable() decorator. This marks the class as one that participates in the dependency injection system . The HeroService class is going to provide an injectable service, and it can also have its own injected dependencies. It doesn’t have any dependencies yet.
The @Injectable() decorator accepts a metadata object for the service, the same way the @Component() (see https://angular.io/api/core/Component ) decorator did for your component classes.
The HeroService could get hero data from anywhere such as a web service, local storage, or a mock data source.
Removing data access from components means you can change your mind about the implementation anytime, without touching any components. They don’t know how the service works.
The service implementation in this tutorial continues to deliver mock heroes .
Import the Hero and HEROES and add a getHeroes method to return the mock heroes in src/app/hero.service.ts :
You must make the HeroService available to the dependency injection system before Angular can inject it into the HeroesComponent by registering a provider . A provider is something that can create or deliver a service. In this case, it instantiates the HeroService class to provide the service.
To make sure that the HeroService can provide this service, register it with the injector . The injector is the object that chooses and injects the provider where the application requires it.
By default, ng generate service registered already a provider with the root injector for your service by including provider metadata, that’s providedIn: 'root' in the @Injectable() decorator.
When you provide the service at the root level, Angular creates a single, shared instance of HeroService and injects into any class that asks for it. Registering the provider in the @Injectable metadata also allows Angular to optimize an application by removing the service if it isn’t used.
To learn more about providers, see the Providers section . To learn more about injectors, see the Dependency Injection guide .
The HeroService is now ready to plug into the HeroesComponent .
Note: This is an interim code sample that allows you to provide and use the HeroService . At this point, the code differs from the final HeroService .

Update HeroesComponent
Open the HeroesComponent class file src/app/heroes/heroes.component.ts .
- Delete the HEROES import, because you won’t need that anymore. Import the HeroService instead.
- Replace the definition of the heroes property with a declaration.
Add a private heroService parameter of type HeroService to the constructor.
The parameter simultaneously defines a private heroService property and identifies it as a HeroService injection site.
When Angular creates a HeroesComponent , the Dependency Injection system sets the heroService parameter to the singleton instance of HeroService .
Create a method getHeroes() to retrieve the heroes from the service.
File src/app/heroes/heroes.component.ts :
While you could call getHeroes() in the constructor, that’s not the best practice.
Reserve the constructor for minimal initialization such as wiring constructor parameters to properties. The constructor shouldn’t do anything . It certainly shouldn’t call a function that makes HTTP requests to a remote server as a real data service would.
Instead, call getHeroes() inside the ngOnInit lifecycle hook (see https://angular.io/guide/lifecycle-hooks ) and let Angular call ngOnInit() at an appropriate time after constructing a HeroesComponent instance.
After the browser refreshes, the application should run as before, showing a list of heroes and a hero detail view when you click a hero name.
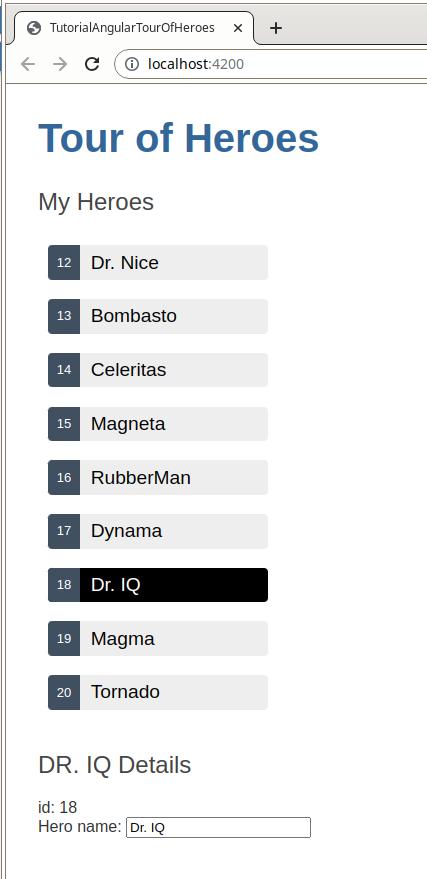
Observable data
The HeroService.getHeroes() method has a synchronous signature, which implies that the HeroService can fetch heroes synchronously. The HeroesComponent consumes the getHeroes() result as if heroes could be fetched synchronously.
This approach won’t work in a real application that uses asynchronous calls. It works now because your service synchronously returns mock heroes .
If getHeroes() can’t return immediately with hero data, it shouldn’t be synchronous, because that would block the browser as it waits to return data.
HeroService.getHeroes() must have an asynchronous signature of some kind.
In this tutorial, HeroService.getHeroes() returns an Observable so that it can use the Angular HttpClient.get method (see https://angular.io/guide/http ) to fetch the heroes and have HttpClient.get() return an Observable .
Observable is one of the key classes in the RxJS library .
In the later part of the tutorial on HTTP, you can see how Angular’s HttpClient (see https://angular.io/api/common/http/HttpClient ) methods return RxJS Observable objects. This tutorial simulates getting data from the server with the RxJS of() function.
Open the HeroService file and import the Observable and of symbols from RxJS and replace the getHeroes() method with the following:
File src/app/hero.service.ts :
of(HEROES) returns an Observable<Hero[]> that emits a single value , the array of mock heroes.
Note: The HTTP tutorial (see later part of this tutorial or https://angular.io/tutorial/tour-of-heroes/toh-pt6 ) shows you how to call HttpClient.get<Hero[]>() , which also returns an Observable<Hero[]> that emits a single value , an array of heroes from the body of the HTTP response.
The HeroService.getHeroes() method used to return a Hero[] . Now it returns an Observable<Hero[]> .
You need to adjust your application to work with that by changing HeroesComponent .
Find the getHeroes method and replace it with the following code. the new code is shown side-by-side with the current version for comparison.
Observable.subscribe() is the critical difference.
The previous version assigns an array of heroes to the component’s heroes property. The assignment occurs synchronously , as if the server could return heroes instantly or the browser could freeze the UI while it waited for the server’s response.
That won’t work when the HeroService is actually making requests of a remote server.
The new version waits for the Observable to emit the array of heroes, which could happen now or several minutes from now. The subscribe() method passes the emitted array to the callback, which sets the component’s heroes property.
This asynchronous approach works when the HeroService requests heroes from the server.
Show messages
This section guides you through the following:
- Adding a MessagesComponent that displays application messages at the bottom of the screen
- Creating an injectable, application-wide MessageService for sending messages to be displayed
- Injecting MessageService into the HeroService
- Displaying a message when HeroService fetches heroes successfully
Use ng generate to create the MessagesComponent:
ng generate creates the component files in the src/app/messages directory and declares the MessagesComponent in AppModule .
Edit the AppComponent template src/app/app.component.html to display the MessagesComponent at the bottom:
You should see the default paragraph “messages works!” from MessagesComponent at the bottom of the page.
Use ng generate to create the MessageService in src/app .
Open MessageService in file src/app/message.service.ts and replace its contents with the following:
The service exposes its cache of messages and two methods:
- One to add() a message to the cache.
- Another to clear() the cache.
In HeroService
- import the MessageService
- Edit the constructor with a parameter that declares a private messageService property. Angular injects the singleton MessageService into that property when it creates the HeroService .
Note: This is an example of a typical service-in-service scenario in which you inject the MessageService into the HeroService which is injected into the HeroesComponent .
Edit the getHeroes() method to send a message when the heroes are fetched.
The MessagesComponent should display all messages, including the message sent by the HeroService when it fetches heroes.
Open MessagesComponent and
- Edit the constructor with a parameter that declares a public messageService property. Angular injects the singleton MessageService into that property when it creates the MessagesComponent .
The messageService property must be public because you’re going to bind to it in the template. Angular only binds to public component properties.
File src/app/messages/messages.component.ts :
Replace the MessagesComponent template created by ng generate with the following.
File src/app/messages/messages.component.html :
This template binds directly to the component’s messageService :
- *ngIf - Only displays the messages area if there are messages to show.
- *ngFor - Presents the list of messages in repeated <div> elements.
- (click) - Angular event binding: Binds the button’s click event to MessageService.clear() .
The messages will look better after you add the private CSS styles to src/app/messages/messages.component.css :
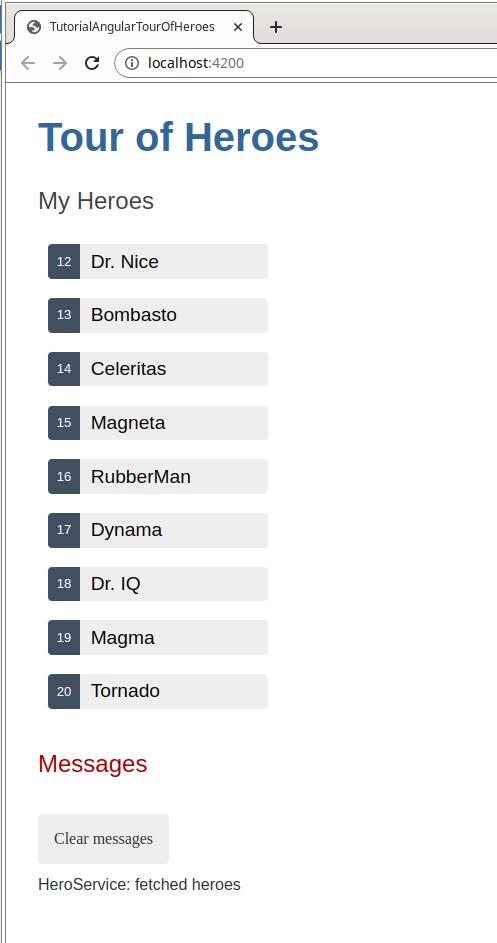
The following example shows how to display a history of each time the user clicks on a hero. This helps when you get to the next section on Routing (see https://angular.io/tutorial/tour-of-heroes/toh-pt5 ).
Refresh the browser to see the list of heroes, and scroll to the bottom to see the messages from the HeroService . Each time you click a hero, a new message appears to record the selection. Use the Clear messages button to clear the message history.
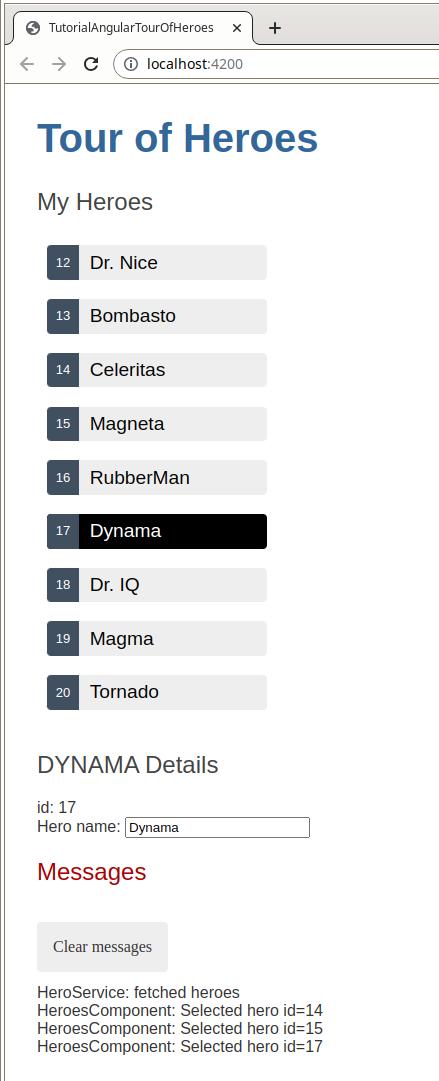
Previous ⏪ Angular Tutorial - Tour of Heroes - Part 4 (Create a feature component)
Next Angular Tutorial - Tour of Heroes - Part 6 (Add navigation with routing) ⏩
This website requires JavaScript.
Navigation Menu
Search code, repositories, users, issues, pull requests..., provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications

IMAGES
VIDEO
COMMENTS
1. I'm currently going through the Angular.io tour of heroes tutorial, making some really minor changes as I go along so I'm not 100% copying the tutorial. I've reached section six on http, more specifically I'm using the In Memory Web Api Service to get a value by id. Get hero by id. Most web APIs support a get by id request in the form api ...
to solve it, try this step. run ng generate service InMemoryData --module=app with your angular cli, then replace the code listed in the tutorial, and save it.; go to app.module.ts, and add some code in it, so the code like this.; app.module.ts. import { HttpClientModule } from '@angular/common/http'; import { HttpClientInMemoryWebApiModule } from 'angular-in-memory-web-api'; import ...
This Tour of Heroes tutorial provides an introduction to the fundamentals of Angular and shows you how to:. Set up your local Angular development environment. Use the Angular CLI to develop an application.; The Tour of Heroes application that you build helps a staffing agency manage its stable of heroes. The application has many of the features that you'd expect to find in any data-driven ...
The forRoot() configuration method takes an InMemoryDataService class that primes the in-memory database.. The in-memory-data.service.ts file takes over the function of mock-heroes.ts.Don't delete mock-heroes.ts yet. You still need it for a few more steps of this tutorial. After the server is ready, detach the In-memory Web API in next step so the application's requests can go through to ...
The Tour of Heroes tutorial takes you through the steps of creating an Angular application in TypeScript. The grand plan for this tutorial is to build an app that helps a staffing agency manage its stable of heroes. The Tour of Heroes app covers the core fundamentals of Angular. You'll build a basic app that has many of the features you'd ...
Router tutorial: tour of heroes. This tutorial provides an extensive overview of the Angular router. In this tutorial, you build upon a basic router configuration to explore features such as child routes, route parameters, lazy load NgModules, guard routes, and preloading data to improve the user experience. For a working example of the final ...
src/app/app.module.ts (imports array excerpt) content_copy @ NgModule ({imports: [HttpClientModule,],}) Simulate a data serverlink. This tutorial sample mimics communication with a remote data server by using the In-memory Web API module.. After installing the module, the application makes requests to and receive responses from the HttpClient.The application doesn't know that the In-memory Web ...
src/app/hero.service.ts content_copy getHeroes (): Hero [] {return HEROES;}. Provide the HeroServicelink. You must make the HeroService available to the dependency injection system before Angular can inject it into the HeroesComponent by registering a provider.A provider is something that can create or deliver a service. In this case, it instantiates the HeroService class to provide the service.
Angular Tour of Heroes tutorial with Angular CLI and Spring Boot - angular-tour-of-heroes/in-memory-data.service.ts at master · rpoitras/angular-tour-of-heroes
You signed in with another tab or window. Reload to refresh your session. You signed out in another tab or window. Reload to refresh your session. You switched accounts on another tab or window.
This Tour of Heroes tutorial provides an introduction to the fundamentals of Angular and shows you how to:. Set up your local Angular development environment. Use the Angular CLI to develop an application.; The Tour of Heroes application that you build helps a staffing agency manage its stable of heroes. The application has many of the features that you'd expect to find in any data-driven ...
Inject in HeroService, which uses the service to send a message Inject in MessagesComponent, which displays that message, and also displays the ID when the user clicks a hero Create the HeroService Run ng generate to create a service called hero. $ cd tutorial-angular-tour-of-heroes $ ng generate service hero CREATE src/app/hero.service.spec.ts ...
A tag already exists with the provided branch name. Many Git commands accept both tag and branch names, so creating this branch may cause unexpected behavior.
Angular Example - Tour of Heroes: Part 6
content_copy ng new angular-tour-of-heroes; ng new prompts you for information about features to include in the initial project. Accept the defaults by pressing the Enter or Return key. ng new installs the necessary npm packages and other dependencies that Angular requires. This can take a few minutes. ng new also creates the following workspace and starter project files:
Angular Example - Tour of Heroes: Part 6
Jan 8, 2018. 2. In this exercise we will use Spring Boot to build a backend from scratch for Angular Tour of Heroes, the popular Angular tutorial application. Part 2 of this series, Getting ...
Angular Demo Project. Contribute to csankhala/angular-tour-of-heroes development by creating an account on GitHub.
Stack Overflow Public questions & answers; Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers; Talent Build your employer brand ; Advertising Reach developers & technologists worldwide; Labs The future of collective knowledge sharing; About the company