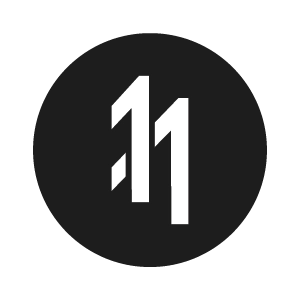

How to open a URL in Safari in Swift
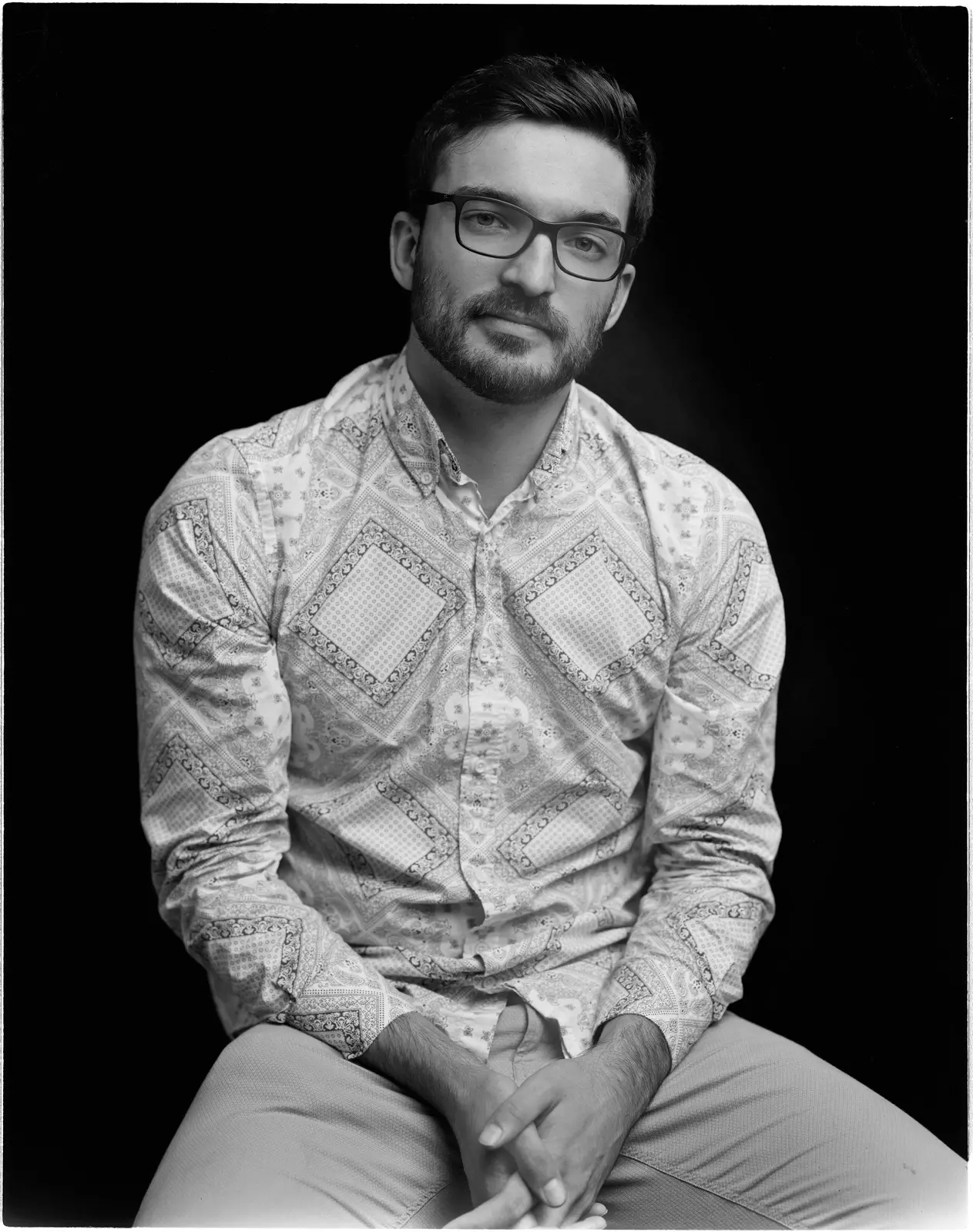
A step by step tutorial on opening a webpage in Safari from an iOS, iPadOS or MacOS app.
The following tutorial builds on our Open Source Swift starter project, which can be downloaded using the link below, and walks you through how to open up a URL in a native Safari browser when a user presses a custom button on an app using Swift.
We recommend downloading our Open Source project, checking out the main branch and carrying out the steps outlined below. All relevant changes can be found on the tutorial/open-url branch.
git clone [email protected]:delasign/swift-starter-project.git
Step One: Create the button
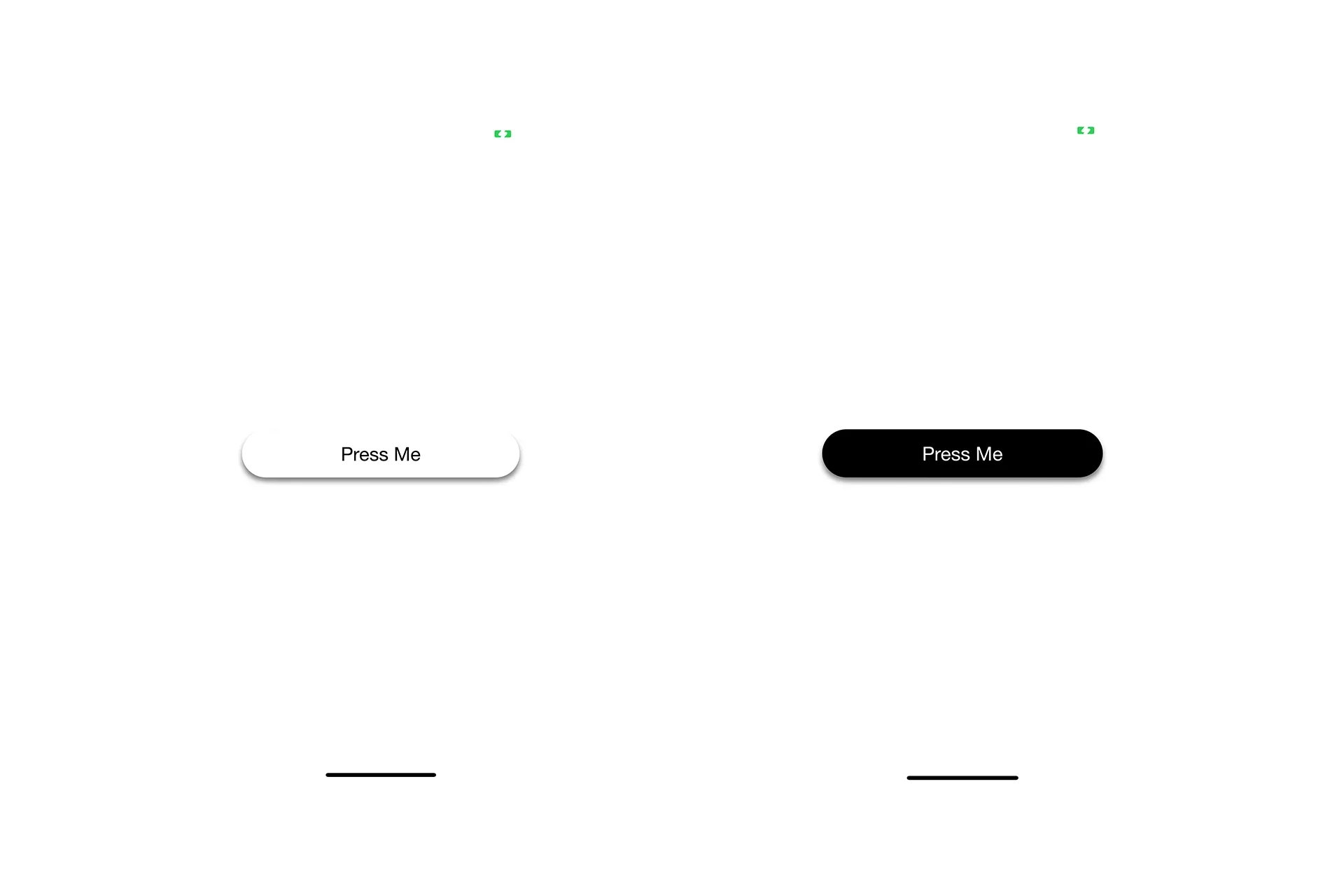
Create the button that opens the URL.
For a tutorial on how to create a custom button in Swift, please consult the article linked below.
Step Two: Create the URL Constant
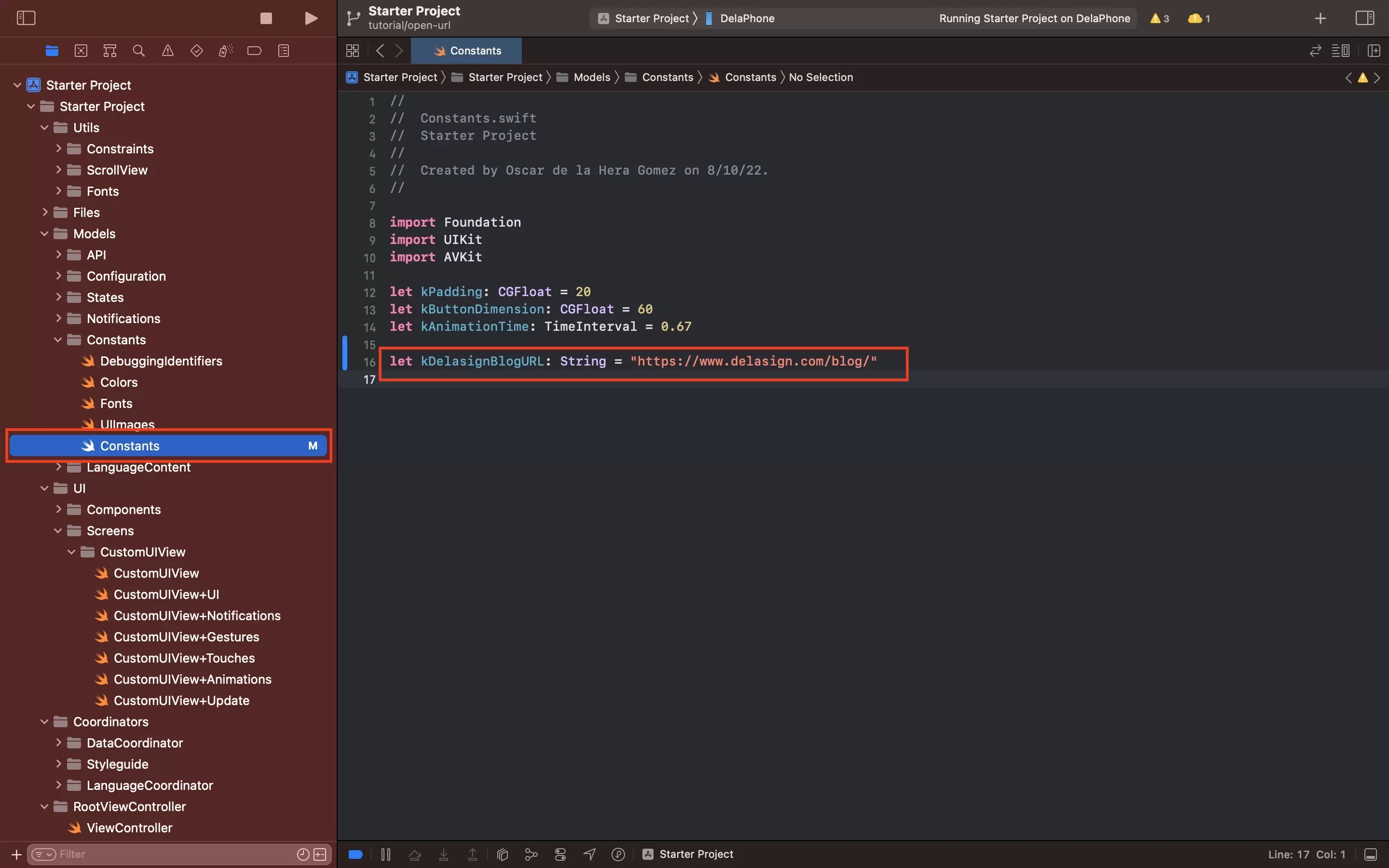
In the Constants.swift file under Models / Constants, add your url as a constant.
Please note that this is optional but recommended.
Step Three: Open the URL
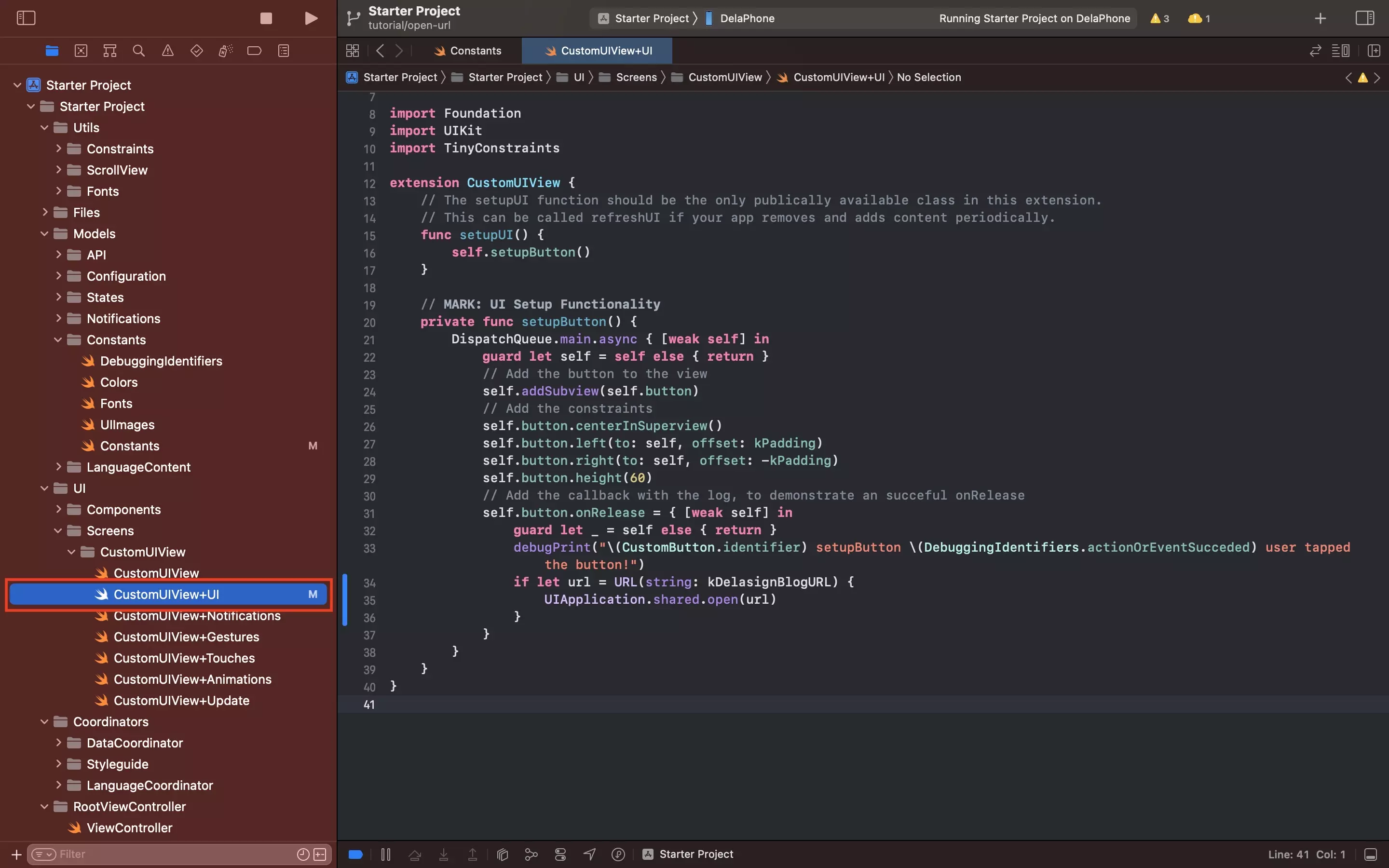
In CustomUIView+UI.swift , in the onRelease callback from the custom button - or in the location that your button action takes place - open up the URL using the code below.
Please replace YOUR_URL with the constant created in Step Two.
Step Four: Verify
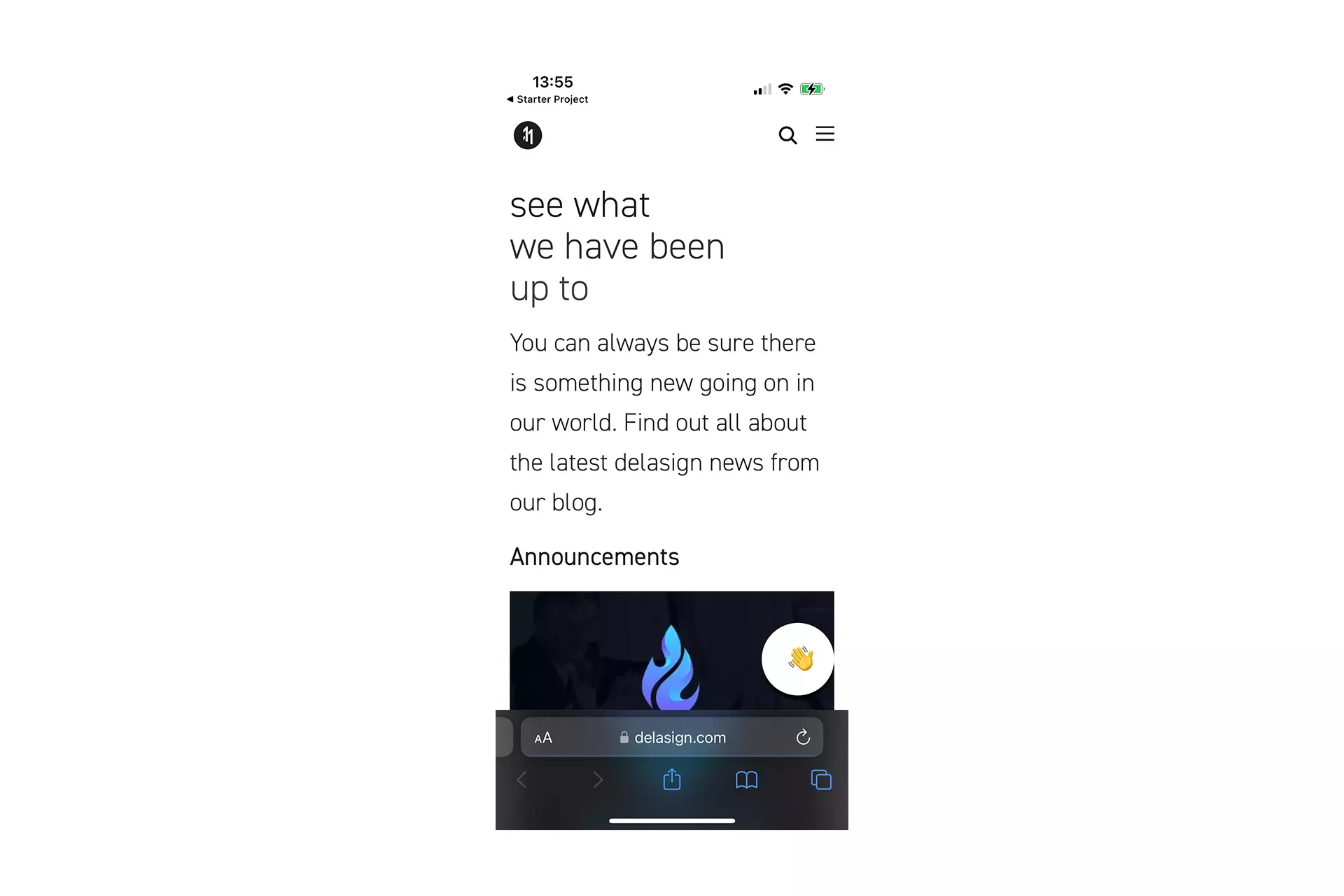
Run the app in XCode and press the button to confirm that the webpage opens in Safari.
Any Questions?
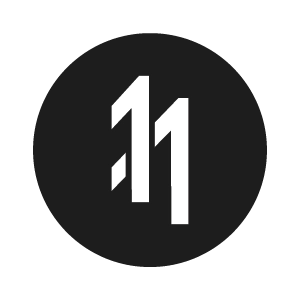
Book a Free Consultation.
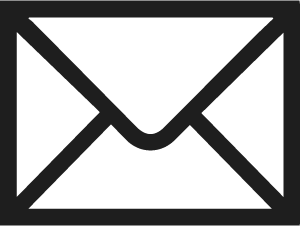
Click here to email us.
Fill in the details below to book a free consultation or to let us know about something else. whatever it is, we are here to help., how can we help you , contact details.
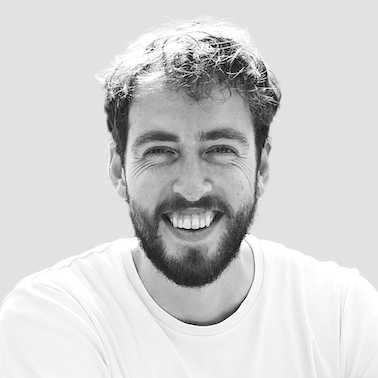
A weekly blog about Swift, iOS and Xcode Tips and Tricks
Every Tuesday, curated Swift content from the community for free.
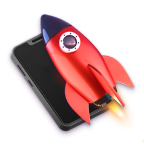
RocketSim: An Essential Developer Tool as recommended by Apple
SwiftLee > SwiftUI > SFSafariViewController in SwiftUI: Open webpages in-app
In this article
SFSafariViewController in SwiftUI: Open webpages in-app
Creating a swiftui wrapper for sfsafariviewcontroller.
- makeUIViewController which will be called to create the UIViewController instance
- updateUIViewController which will be called to update the state of the UIViewController with new information from SwiftUI
Creating a reusable view modifier
Presenting a sfsafariviewcontroller in swiftui, featured swiftlee jobs.
- Lead Software Engineer @ M7 Health • $105K - $185K
- Senior Android Engineer @ Speechify
- Test Automation Lead, Engineering Productivity @ Ordergroove • $140K - $170K
WebView in SwiftUI
There will be a time when you want to open up web content in your apps . For example, you might want to open up your terms and conditions, privacy policy, or direct users to your home page.
When we want to open web content in UIKit , we can do it in three ways .
- Open the Safari app . This will direct users out of your app to the Safari app .
- Using SFSafariViewController . This is a built-in browser that gives you the same experience as the Safari app, but you can open it within your app .
- Using WKWebView . This is the most flexible one . You can create your own in-app browser with custom controls using WKWebView .
The bad news is SwiftUI doesn't support all of that .
It only supports the first case, which is open up web content in the Safari app .
In this article, we will learn what SwiftUI supported and how to overcome the limitation with UIViewRepresentable .
How to open a URL in Safari
SwiftUI has a Link control that opens a web page from the specified URL .
You can think of it as a button where the action opens up the destination URL on the Safari app.
Here is how it looks.
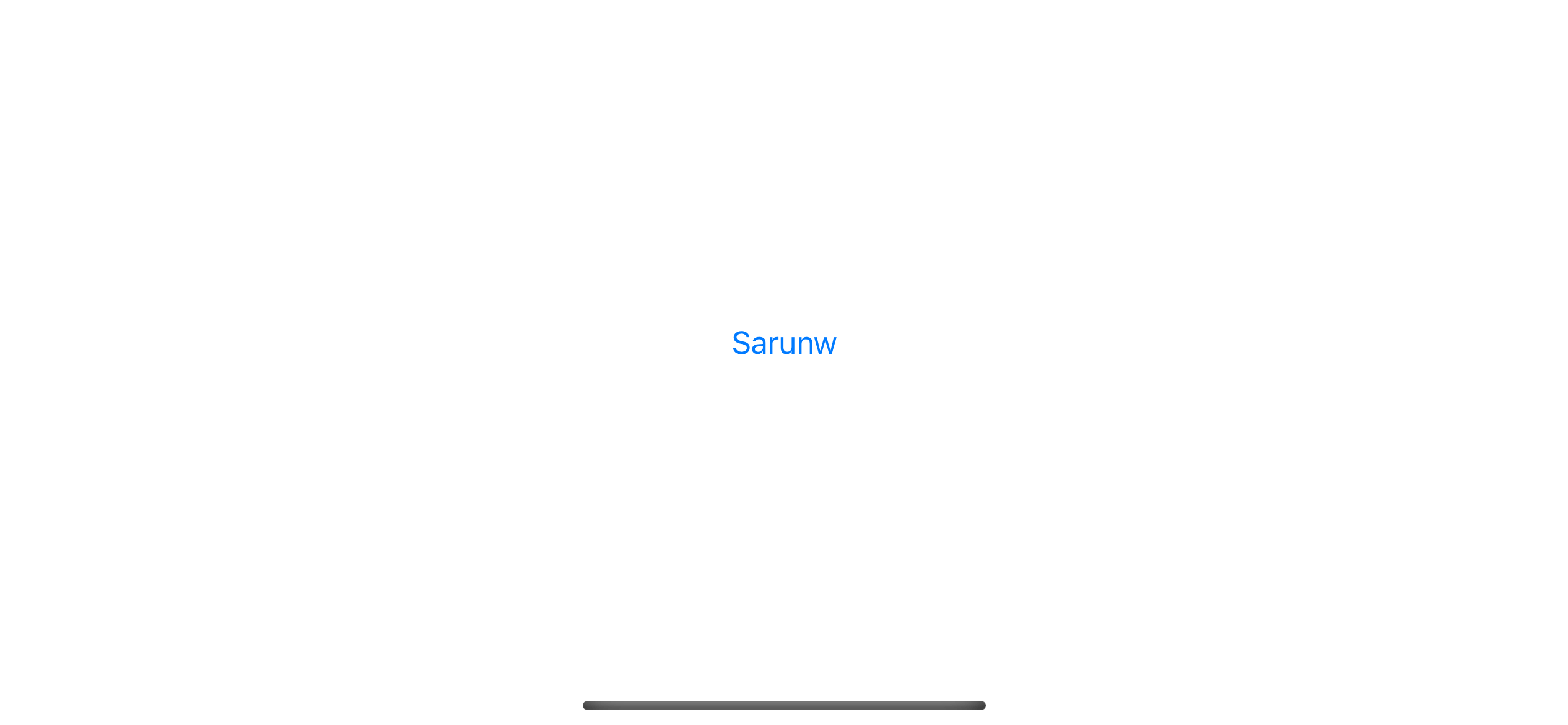
It will open up an external browser when tapped.
Customization
Link is just a normal control. You can use it like other controls.
In the following example, we use it inside a list view and change its color to black with .foregroundColor(.black) .
Link got the same treatment when presenting inside a list view, just like a Button .
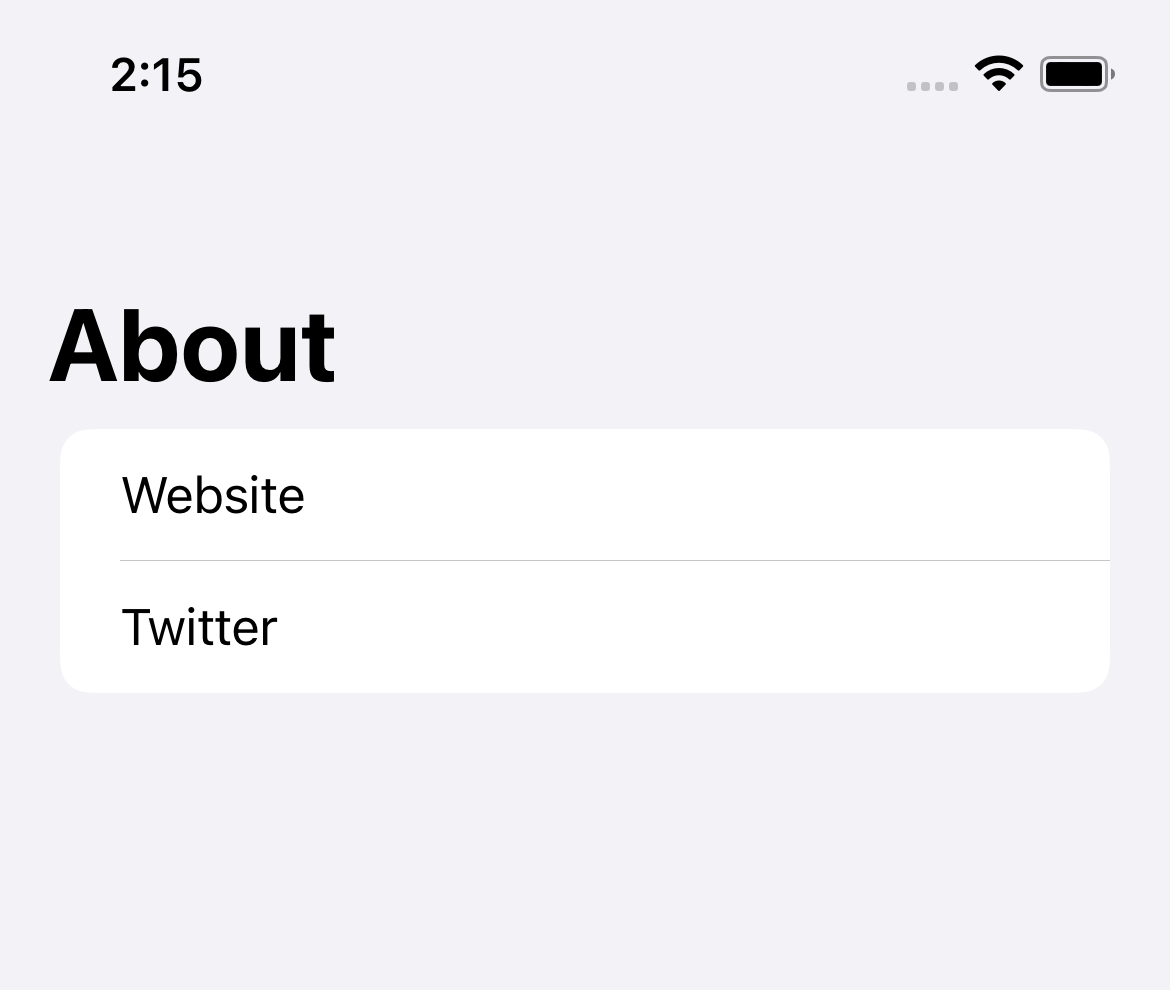
A link label doesn't need to be text.
You can use any view to represent your link by using another form of initializer that accepts a view builder .
Here is an example where I use an image as a link label .
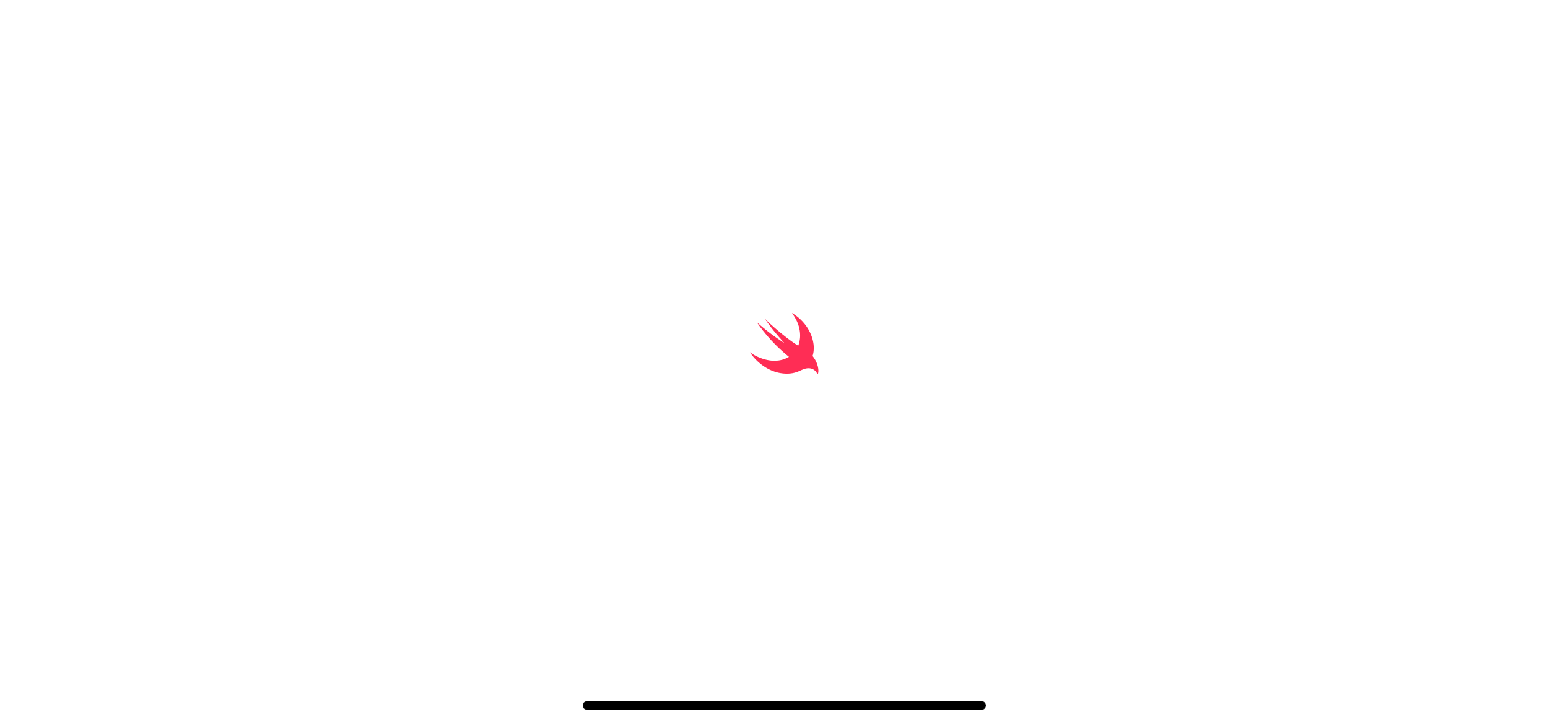
You can easily support sarunw.com by checking out this sponsor.
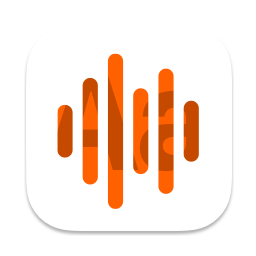
Offline Transcription: Fast, privacy-focus way to transcribe audio, video, and podcast files. No data leaves your Mac.
How to open a WebView in SwiftUI
As mentioned earlier, the current version of SwiftUI (iOS 16) doesn't have native support of WKWebView or SFSafariViewController , so we don't have a native way to present an in-app browser in SwiftUI .
To present an in-app web view in your app, you need help from UIKit and UIViewRepresentable .
There are many ways to do this, and there is no right or wrong. I will give you an easy implementation to integrate WKWebView into a SwiftUI app.
Here is how to do it.
Bridging WKWebView to SwiftUI using UIViewRepresentable
First, we need to change WKWebView into the format that SwiftUI understands.
We can do this by using UIViewRepresentable . If you are not familiar with this protocol, you can read more about it in How to use UIView in SwiftUI .
We create a new SwiftUI View, WebView , which uses WKWebView under the hood.
1 We declare a url property to store a URL we want to open. 2 makeUIView() is a required method from UIViewRepresentable . We return UIView that we want to bridge from the method. In this case, it is WKWebView . 3 updateUIView() is another requred method. This method gets called when there is a state change. In this case, we load a new URL if there is a change .
With this simple implementation, we can use WKWebView in our SwiftUI app.
Use WKWebView in SwiftUI
After you create UIViewRepresentable , you can use it like a normal SwiftUI view.
As an example, we will present a web view using a sheet presentation.
1 Declare isPresentWebView to control the presentation of the sheet. 2 Present the sheet when the button is tapped. 3 Present WebView with the URL that you want. In this case, I wrap it inside a NavigationStack and add a navigation title, but you don't need to do this.
Here is the result.
You may also like
- How to use UIView in SwiftUI 29 Sep 2022
- How to use UIKit in SwiftUI 30 Jun 2019
- How to use UIViewController in SwiftUI 12 Sep 2022
- How to use SFSafariViewController in SwiftUI 27 Apr 2023
- How to open URL in Safari in SwiftUI 24 Apr 2023
- Inset grouped List in SwiftUI 30 Dec 2019
Enjoy the read?
If you enjoy this article, you can subscribe to the weekly newsletter. Every Friday , you'll get a quick recap of all articles and tips posted on this site . No strings attached. Unsubscribe anytime.
Feel free to follow me on Twitter and ask your questions related to this post. Thanks for reading and see you next time.
If you enjoy my writing, please check out my Patreon https://www.patreon.com/sarunw and become my supporter. Sharing the article is also greatly appreciated.
There will be a time when you want to get an array of all enum cases, such as when you want to present them in a list or picker. CaseIterable protocol is what you are looking for.
Is Swift sorting stable or not?
- Sponsorship
- Become a patron
- Buy me a coffee
- Privacy Policy
Livestreams
Lite mode on
Quick links
Suggested search
Load Safari Inside App
Add to favorites
Load Safari as a fullScreenCover inside of your application
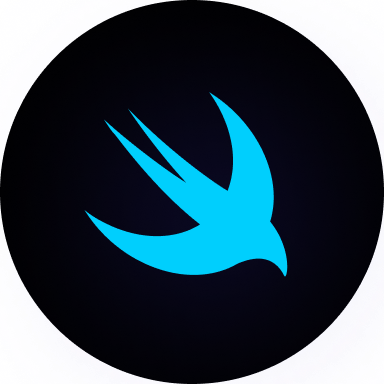
SwiftUI Handbook
Visual Editor in Xcode
Stacks and Spacer
Import Images to Assets Catalog
Shapes and Stroke
Color Literal and Image Literal
Safe Area Layout
Custom Fonts
Max Width and Frame Alignment
Shadows and Color Opacity
Mask and Transparency
Clip Shape and Smooth Corners
Tab View Pagination
Animation States
View Transitions and Animations
Animation Modifier and Timing
Transform Animations
Tap Animation with Delay
Long Press Gesture
Drag Gesture
Matched Geometry Effect
Advanced Matched Geometry Effect
Swift Package Manager
Background Blur
Lottie Animation
Lazy Grid Layout
Lazy Stacks
Navigation View
Color Picker
Date Picker
Hover Effects
Static Data
Full Screen Modal
Hide Status Bar
Redacted Placeholder
Horizontal Scroll with rotate3DEffect
Animation Repeat, Delay and Speed
Tab Selection from Child View
Status Bar Size with GeometryReader
Modal Presentation
Remote Images
Dismiss custom modal
Iterating Over an Array
Switch Statement
Format Date
UserDefaults
Hide Keyboard
Play Video with AVPlayer
Controls with AVPlayer
OnScroll listener
Link from a Text
Share Sheet
Strings in Swift
Xcode Playground
Action sheet
TabBar scroll to top
TabBar to root View
Status bar background on scroll
Create a Widget
ContainerRelativeShape
WidgetFamily sizes
SwiftUI Picker
Conditional modifier
Markdown with AttributedString
AttributeContainer
Format Date with Formatted
List Row Separators
Swipe actions
Light and Dark Modes
SwiftUI Canvas
Animations with TimelineView
SecureField
Background Animation with Gradient
Inner Shadow
SF Font Width Styles
Multiple Blending Modes
Circular Text on Path
View That Fits
Navigation Stack
Grid Layout
SVG to SwiftUI
Detect Screen Size
Custom Layout
Radial Layout
AnyLayout Transition
Core Location in SwiftUI
Radial Gradient Button Animation
SF Symbols 5 Animations
Phase Animator in SwiftUI
Keyframe Animator in SwiftUI
Specific Corner Radius
Observable Models
SwiftData Persistence
Create a new file
Create a new file called SFSafariViewWrapper.swift . In this view, import SwiftUI and SafariServices. SafariServices lets us use Safari inside of our application.
Create a SFSafariViewWrapper
Create the SFSafariViewWrapper below. It'll conform to a UIViewControllerRepresentable. It takes a URL as an argument. We're also adding two functions to this ViewWrapper : a makeUIViewController and an updateUIViewController , which are required when creating a UIViewControllerRepresentable .
Create state
Go to ContentView . In here, we'll create a private state called showSafari and we'll toggle it to show the web page in Safari, on top of our view.
Open Safari
In the body of ContentView , we'll change the text Hello, world to Open Design+Code in Safari .
OnTapGesture of that Text, we'll toggle the showSafari state.
When showSafari is true, we'll show a fullScreenCover . The content of that fullScreenCover is the SFSafariViewWrapper we created at the beginning. This takes a url as an argument, so we'll create a URL for designcode.io .
And voilà! You now have created a built-in Safari browser inside of your application when users try to open a link.
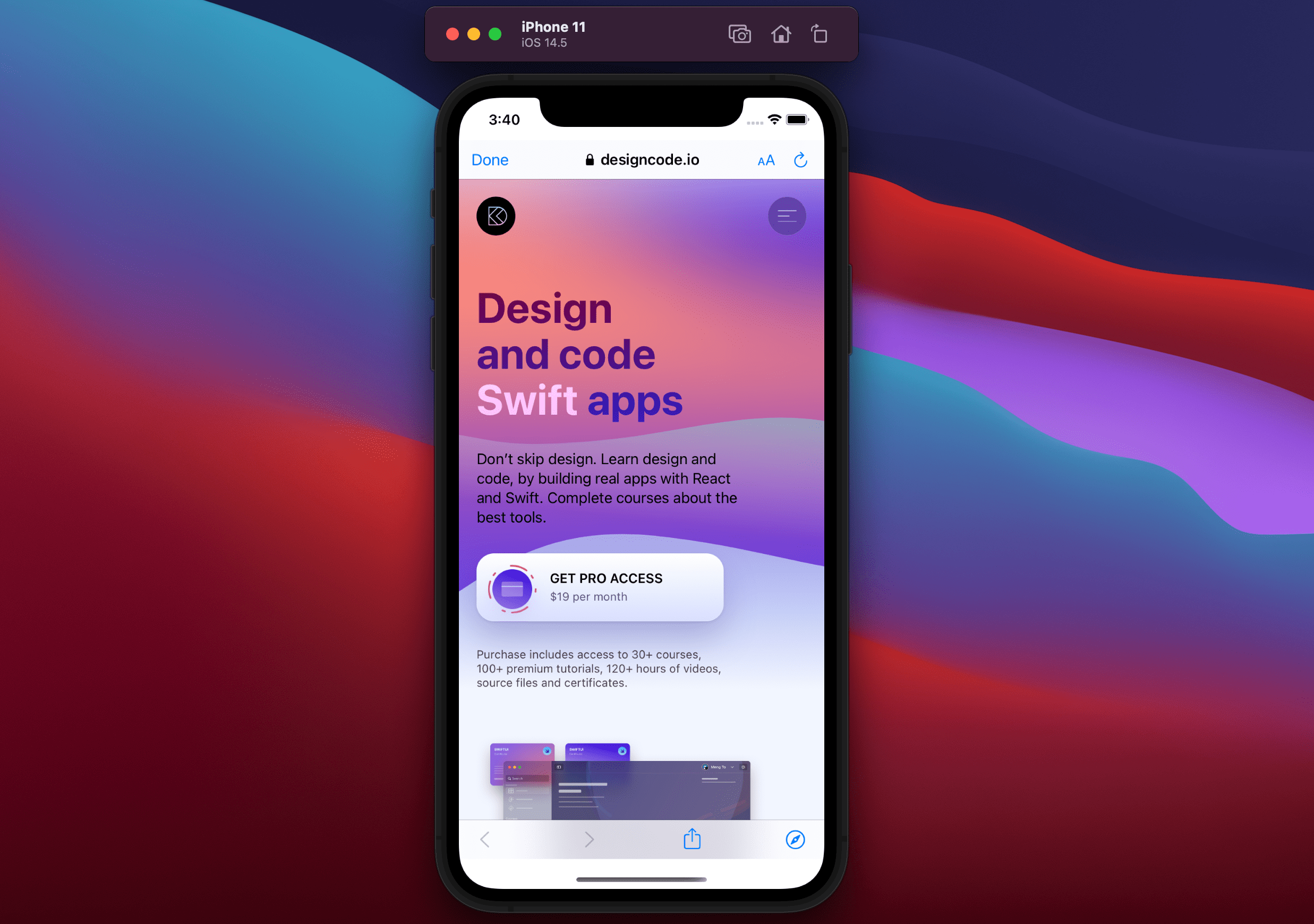
Learn with videos and source files. Available to Pro subscribers only.
Get Pro Access
$19 per month
Purchase includes access to 50+ courses, 320+ premium tutorials, 300+ hours of videos, source files and certificates.
Templates and source code
Download source files
Download the videos and assets to refer and learn offline without interuption.
Design template
Source code for all sections
Video files, ePub and subtitles
swiftui-handbook-safari-inside-app
Design your layout using the inspector, insert menu and modifiers
Learn how to use HStack, VStack, ZStack with spacing and alignment
How to import images from Figma to Xcode using PDF, PNG and JPG
How to use shapes like circle, ellipse, capsule, rectangle and rounded rectangle
How to use system icons for Apple platforms with different size, scale and multicolor
Use the color picker and images list to set your colors and images directly in the code
Learn how to create a Sidebar navigation for iOS, iPadOS and macOS
Use the Toolbar modifier to place multiple items in the navigation bar or bottom bar
How to work with the Image View and its resizable, aspectRatio, scaleToFit and resizingMode options
How to ignore the Safe Area edges in a typical layout with a background
A deep dive into how to use the Text View and its modifiers such as font, color, alignment, line spacing and multiple lines
How to set a custom font in iOS using info.plist and the font modifier
An alternative to stacks and spacer is to use frame max width and alignment to avoid the pyramid of doom
The most flexible way to add shadows to your UI in SwiftUI
How to use mask to clip the content with opacity and gradient
How to create a continuous corner radius, also known as super ellipse
Create a simple user onboarding layout using Tab View with the PageTabViewStyle
How to set up a simple animation using states, toggle, withAnimation and onTapGesture
Animate your screens using the transition modifier and preset animations
How to apply a different animation timing on separate elements using the animation modifier
How to animate a card using offset, scaleEffect, rotationEffect and rotation3DEffect
Expand and contract a button using the tap gesture with delay
Detect the long press duration to expand a button and bounce back
Learn how to create a draggable card using DragGesture, onChange, onEnded events and the offset modifier
Create a custom transition between views using the matchedGeometryEffect modifier, namespace and id
Recreate the Music app transition using matched geometry effect and learn how to pass Namespace to another view
How to install SwiftUI packages using the Swift Package Manager
How to apply a frosted glass sheet in your user interface using Apple's sample code
How to add animated assets using Lottie in SwiftUI
Learn how to build an adaptive or fixed grid layout that expands vertically or horizontally
Get better scroll performance by using LazyHStack and LazyVStack instead of HStack and VStack
Create a native navigation for your app using the nav bar, large title and swipe gesture
Learn how to open a URL in the Safari browser and how to customize your Link
How to set and customize the native color picker in SwiftUI
How to let users pick a date and time using a dropdown wheel or a calendar style
How to design for iPadOS pointer using hoverEffect and onHover
How to create reusable components by using the Extract Subview option in SwiftUI
How to synchronize states across multiple views and set the constant for your preview
Work with static data in SwiftUI.
How to present a full screen modal without the sheets UI
How to hide your app's status bar with or without animation
Create a placeholder UI while loading using the redacted modifier
How to apply a beautiful 3D transform while scrolling in SwiftUI
How to loop and delay your animation using repeat, repeatForever, speed and delay
How to programmatically link to another tab from any child view in SwiftUI
Using SafeAreaInsets, you can get the height of the status bar, tab bar dynamically
Use the .sheet modifier to show a modal
Load images from the Internet in your SwiftUI application with SDWebImage
Create an "OnTapOutside" listener on dismiss a custom modal
Use ForEach to loop through an array in a View and in a function
Learn how to use switch statements in Swift
Transform how dates are displayed in your application by using a Date Extension and DateFormatter
Cache your user's data with UserDefaults
Use a View extension to dismiss the keyboard when the user taps outside of the keyboard area
Add a video or an audio player to your SwiftUI application by using AVPlayer and AVKit
Play, pause, change the video speed, get the current time or the duration, and add subtitles to a video, all using AVPlayer
Use SwiftUITrackableScrollView to add a listener when the user scrolls
Open a web page in Safari when the user clicks on a link in your SwiftUI application
Call Apple's share sheet when the user clicks on a button
Learn the basics of Strings in Swift and how to manipulate them
Use Xcode Playground to test your Swift functions and save time
Use the newly introduced AppStorage to add to UserDefaults
Show an action sheet with multiple options for the user to choose from
Programmatically scroll to top when the user taps more than once on the the tab bar
Learn how to programmatically go back to the root View when the user taps on the tab item twice
Learn how to add a background color on the status bar while the user is scrolling
Add widget to your existing SwiftUI project with custom data
Adding an adaptive radius to a widget
Adding support for various family sizes in a widget
Create a wheel picker using SwiftUI's built-in Picker, to allow the user to select from multiple choices
Learn different ways to add a conditional modifier to your SwiftUI view
Use AttributedString to generate formatted text from markdown
Style AttributedStrings with AttributeContainer
Load remote images using AsyncImage
Use SwiftUI 3.0's .formatted() function to format a date
Customize list row separators
Easily add swipe actions to a row in a list
Effortlessly search through a list
Automatically adapt to light and dark mode
Create efficient and powerful 2D drawings
Synchronize animations with timed events
Create a text field with hidden text that is perfect for password fields
Play with unit points, gradients, masking, blurs and a timer to create a beautiful gradient animation
Apply multiple inner shadows on a Text, SF Symbol or Shape in iOS 16
Implement SF Font Compressed, Condensed and Expanded width styles using a font extension
Combine Difference, Hue and Overlay blend modes to create a text that has great contrast on both light and dark backgrounds
Create a text that follows a circle path by using GeometryReader, PreferenceKey and calculating the angles
Use ViewThatFits to make your layout adaptive without using GeometryReader or conditions
Create a navigation stack list with data and multiple destinations
Present a bottom sheet UI natively and control the sizes and drag zones
SwiftUI 4 makes creating charts with the Chart view easy and efficient, providing vivid visuals for data without 3rd-party libs
Grid Layout provides developers with the power to design custom layouts, tables and grids, with control over element size and positioning
Learn how to turn Figma shapes into SwiftUI code and create beautiful card designs with linear gradients and grid layouts
Use UIScreen.main.bounds, GeometryReader, and PreferenceKey to detect and track screen sizes with SwiftUI
Make your own designs with the CustomLayout protocol and change the point values by using affine transformations
Create a custom radial layout by calculating the center point and radius of a view
Transition between different layouts and animate them with AnyLayout while keeping the same structure and content
Learn how Core Location Manager shows where a device is and its direction. Our guide helps you use it easily for compass directions.
Create a custom button with radial gradients and a glow animation
Dive into SF Symbols 5: Master animated icons for a dynamic, engaging app experience
Create multi-stage Animations with ease
Learn how to leverage SwiftUI's keyframe animator to bring your app to life with captivating animations
Learn how to create precise and unique corner radii using various techniques in SwiftUI
Simplify app development and eliminate boilerplate code with observable view models in SwiftUI
Learn SwiftData fundamentals - define models, set up persistence, fetch, query, and modify data with code examples
Meet the instructor
We all try to be consistent with our way of teaching step-by-step, providing source files and prioritizing design in our courses.
Stephanie Diep
iOS and Web developer
Developing web and mobile applications while learning new techniques everyday
7 courses - 35 hours
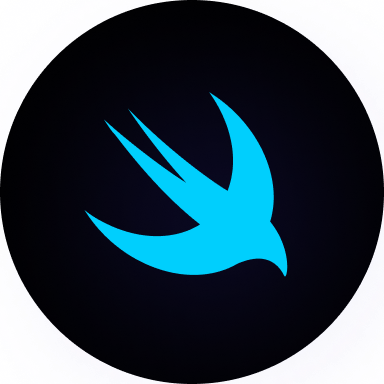
Build Quick Apps with SwiftUI
Apply your Swift and SwiftUI knowledge by building real, quick and various applications from scratch
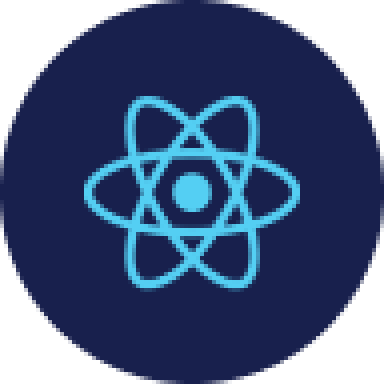
Advanced React Hooks Handbook
An extensive series of tutorials covering advanced topics related to React hooks, with a main focus on backend and logic to take your React skills to the next level
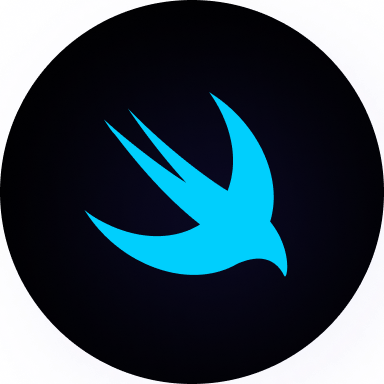
SwiftUI Concurrency
Concurrency, swipe actions, search feature, AttributedStrings and accessibility were concepts discussed at WWDC21. This course explores all these topics, in addition to data hosting in Contentful and data fetching using Apollo GraphQL
SwiftUI Combine and Data
Learn about Combine, the MVVM architecture, data, notifications and performance hands-on by creating a beautiful SwiftUI application
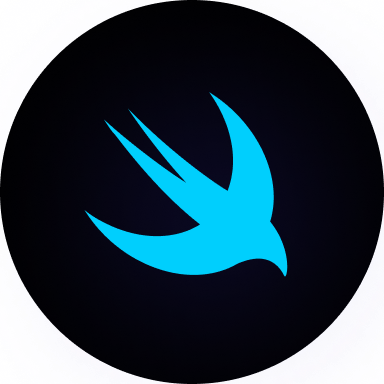
SwiftUI Advanced Handbook
An extensive series of tutorials covering advanced topics related to SwiftUI, with a main focus on backend and logic to take your SwiftUI skills to the next level
React Hooks Handbook
An exhaustive catalog of React tutorials covering hooks, styling and some more advanced topics
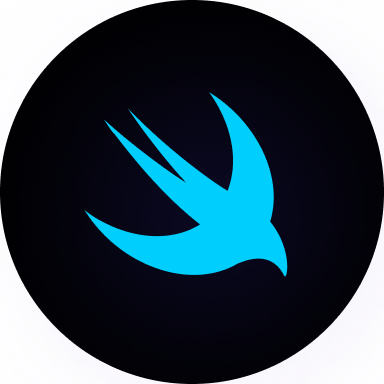
A comprehensive series of tutorials covering Xcode, SwiftUI and all the layout and development techniques
Site made with React, Gatsby, Netlify and Contentful. Learn how .
Design+Code © 2024
Need help? Contact Us
how to open safari in my swift program
- Custom Apps
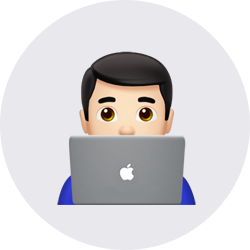
Thanks a lot, it helped)

SafariPanzerBattle 17+
Rightcode inc., designed for ipad, screenshots, description.
Safari Panzer Battle is an adrenaline-fueled action combat game set in the wild. Players control various animals, utilizing skill and strategy to engage in intense shootouts. Mighty elephants, robust rhinos, and swift cheetahs each bring their unique abilities and weapons to the battlefield. Players must skillfully dodge enemy attacks and retaliate with precise aim. The battlegrounds range from deep jungles to vast savannas, each requiring different strategies. Finding hidden paths for surprise attacks or using the terrain for defense offers limitless tactical possibilities. Furthermore, players can strengthen their characters with each battle, gaining more powerful attacks and defensive skills as they progress. The goal is to rise to the top in a world where diverse animals compete, using one's own skill and wit. Safari Panzer Battle, with its intuitive gameplay and strategic depth, will captivate gamers of all ages. Whether competing with friends or honing skills alone, aim for the apex of the animal kingdom in this epic battle.
Version 1.0.1
Fixed minor bugs.
App Privacy
The developer, RightCode Inc. , indicated that the app’s privacy practices may include handling of data as described below. For more information, see the developer’s privacy policy .
Data Used to Track You
The following data may be used to track you across apps and websites owned by other companies:
- Identifiers
Data Linked to You
The following data may be collected and linked to your identity:
Data Not Linked to You
The following data may be collected but it is not linked to your identity:
- Diagnostics
Privacy practices may vary, for example, based on the features you use or your age. Learn More
Information
English, Japanese
- Developer Website
- App Support
- Privacy Policy
More By This Developer
- a. Send us an email
- b. Anonymous form
- Buyer's Guide
- Upcoming Products
- Tips / Contact Us
- Podcast Instagram Facebook Twitter Mastodon YouTube Notifications RSS Newsletter
New AI Features Reportedly Coming to Safari in iOS 18
Safari will gain a series of AI-powered features and UI enhancements in iOS 18 , AppleInsider reports .
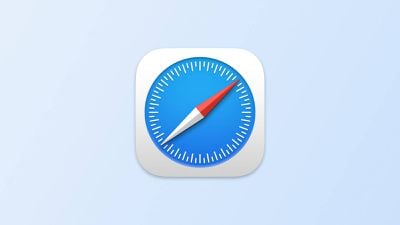
- An "Intelligent Search" browser assistant tool that leverages Apple's on-device AI technology to identify key topics and phrases on webpages for summarization purposes.
- A "Web Eraser" tool that allows users to remove unwanted portions of webpages easily. Erasure is persistent, remaining even when revisiting a site unless changes are reverted.
- A new, quick-access menu emerging from the address bar that consolidates page tools, bringing over some functions that currently sit in the Share Sheet and placing them alongside the new tools.
The iPadOS and macOS versions of Safari are also expected to align further. These new features are purportedly undergoing evaluation alongside internal builds of iOS 18 and macOS 15 ahead of their unveiling at Apple's Worldwide Developer's Conference (WWDC) in June. Earlier this month, backend code on Apple's servers suggested that a new Safari browsing assistant is in the works, corroborating this report.
Farther in the future, Apple is said to be working on a more powerful version of Visual Look Up that allows users to obtain information on products when browsing through images. The feature is expected to be released sometime in 2025.
Last week, Bloomberg 's Mark Gurman said that iOS 18 will "overhaul" many of Apple's built-in apps , including Notes, Mail, Photos , and Fitness. Apple's next-generation operating systems are almost certain to be previewed during Apple's WWDC keynote on June 10, and the updates should be widely released in the fall.
Get weekly top MacRumors stories in your inbox.
Top Rated Comments

A "Web Eraser" tool that allows users to remove unwanted portions of webpages easily. Erasure is persistent, remaining even when revisiting a site unless changes are reverted.
Popular Stories
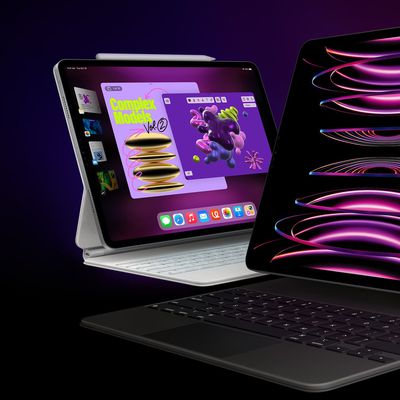
Apple Event Rumors: iPad Pro With M4 Chip and New Apple Pencil With Haptic Feedback
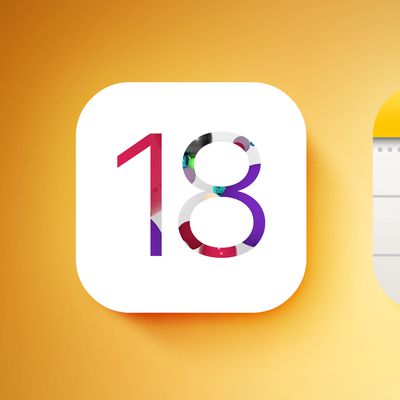
iOS 18 Rumored to 'Overhaul' Notes, Mail, Photos, and Fitness Apps
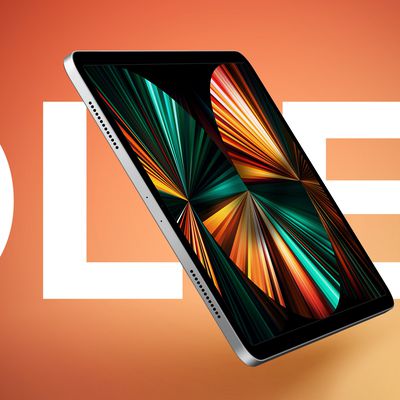
Apple to Use 'Best OLED Panels on the Market' for Upcoming iPad Pro
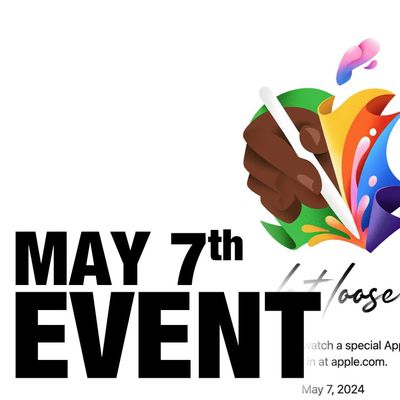
Apple Announces 'Let Loose' Event on May 7 Amid Rumors of New iPads
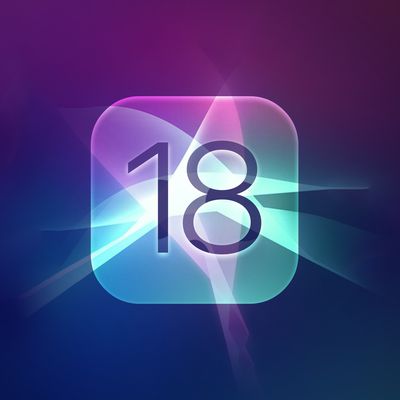
iOS 18 Rumored to Add These 10 New Features to Your iPhone
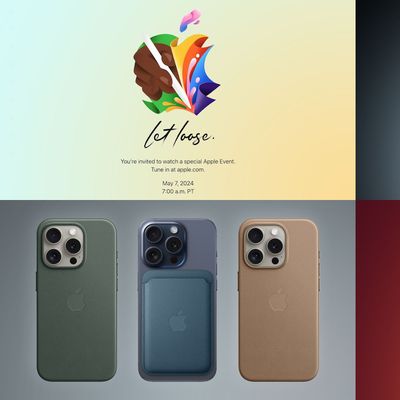
Top Stories: Apple Announces 'Let Loose' Event With New iPads and More Expected
Next article.
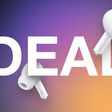
Our comprehensive guide highlighting every major new addition in iOS 17, plus how-tos that walk you through using the new features.
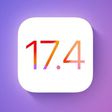
App Store changes for the EU, new emoji, Podcasts transcripts, and more.
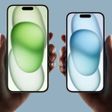
Get the most out your iPhone 15 with our complete guide to all the new features.
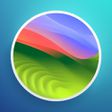
A deep dive into new features in macOS Sonoma, big and small.
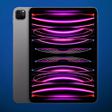
Revamped models with OLED displays, M3 or M4 chip, and redesigned Magic Keyboard accessory.
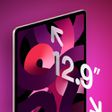
Updated 10.9-inch model and new 12.9-inch model, M2 chip expected.
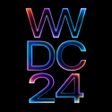
Apple's annual Worldwide Developers Conference will kick off with a keynote on June 10.
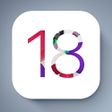
Expected to see new AI-focused features and more. Preview coming at WWDC in June with public release in September.
Other Stories
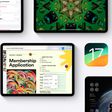
20 hours ago by Tim Hardwick
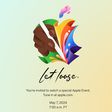
5 days ago by Tim Hardwick
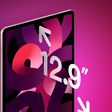
6 days ago by Joe Rossignol
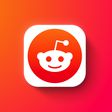
6 days ago by MacRumors Staff
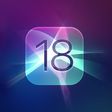
1 week ago by Joe Rossignol
trending now

Mark Consuelos tells wife Kelly Ripa he kissed another woman in...

'Vanderpump Rules' pausing production, won't film this summer to...

ABC News weatherman Rob Marciano fired over ‘anger’ issues,...

Jessica Simpson says it’s easy to ‘blow money’ on vacation...

Melissa Gorga celebrates end of Teresa Giudice friendship in...

Melissa McCarthy responds to Barbra Streisand boldly asking if...

Benny Blanco says he makes Selena Gomez her favorite steak to get...

Kyle Richards' daughter Farrah had $1M in goods, stepdad Mauricio...
- Click to share on Facebook (Opens in new window)
- Click to share on Twitter (Opens in new window)
- Click to email a link to a friend (Opens in new window)
- Click to copy URL
Leah McSweeney’s sober dating app hosts first event
- View Author Archive
- Get author RSS feed
Thanks for contacting us. We've received your submission.

Leah McSweeney’s sober dating app, Club Pillar, hosted its first in-person event with artistes of abstinence including Cat Marnell, TikTok star Kelsey Soles and McSweeney herself all on the scene.
The bash at Bisou in Tribeca featured sober singles, 1980s grooves, burlesque dancers, mocktails from Aplos and QR-coded condoms.
We’re told the “ambience matched the application’s aesthetic: red, sexy and mysterious.”

You (and a guest!) could win a VIP experience in NYC for Virtual Reali-Tea Live!
The app calls itself the “first sober private members community” and says it “seeks to redefine dating and social experiences for those committed to a sober lifestyle or looking to cut back on drinking.”
CEO Jessie Urvater told us of the party, “this is the only beginning . . . We will have three more events in NYC before [the official] launch and each event will be filled with surprises and stimulations.”
The event marked something of a fresh start for McSweeney, we’re told, after leaving the “Real Housewives of New York City.” As an adviser and “creative lead” on the project , we hear she saw it as a chance to move on and put life as a reality star behind her.

McSweeney is currently suing Bravo over her time on the show.
Announcing the project on Instagram in February, the Married to the Mob designer wrote, “My sober journey has been long and tumultuous and somewhat public (at least the last 4 years of it). I have experienced first-hand the stigma and judgment surrounding alcohol use disorder but also the stigma surrounding sobriety or choosing NOT to drink.”

She added that as “the first sober private members community,” Club Pillar “seeks to redefine dating and social experiences for those committed to a sober lifestyle or looking to cut back on drinking.”
McSweeney said that the community will use an invite-only model designed to create “a selective, safe group of members.”
“This is not only for people in recovery but people who just don’t love getting wasted or drinking,” she added.
Share this article:

Advertisement

IMAGES
VIDEO
COMMENTS
Paul Hudson @twostraws June 17th 2021. If you want the user to exit your app and show a website in Safari, it's just one line of code in Swift. I'll make it three here because I'll create the URL in the code too, then safely unwrap it: if let url = URL(string: "https://www.hackingwithswift.com") { UIApplication.shared.open(url) }
A step by step tutorial on opening a webpage in Safari from an iOS, iPadOS or MacOS app. Subscribe The following tutorial builds on our Open Source Swift starter project, which can be downloaded using the link below, and walks you through how to open up a URL in a native Safari browser when a user presses a custom button on an app using Swift.
BUILD THE ULTIMATE PORTFOLIO APP Most Swift tutorials help you solve one specific problem, but in my Ultimate Portfolio App series I show you how to get all the best practices into a single app: architecture, testing, performance, accessibility, localization, project organization, and so much more, all while building a SwiftUI app that works on iOS, macOS and watchOS.
swift 3 open URLs in safari or native app. 0. How create an iPhone app that just starts a website-1. How can I load a website by clicking a UIImage View? 0. Open Itunes app link in uiwebView. Related. 2. Open WebView URL in Browser. 0. Open WebView links in Safari View Controller. (Xcode, Swift) 2.
This short video will show you how to open a link from your own iOS Application using Swift!Project Code:https://github.com/ajaygandecha/Youtube-Video-FilesF...
In today's video I show you how to use the new Link view in SwiftUI and iOS 14. This will open up a website in Safari directly from your app. This tutorial w...
SFSafariViewController can be used to let your users open webpages in-app instead of in an external browser. While the view controller works great for UIKit, getting it to work in a SwiftUI app might be challenging. Whenever you're running into cases where a UIKit solution is available only, you want to know how to write a wrapper and make ...
A Safari app extension is uniquely useful because it can communicate with a native app. Sharing data between an app and Safari lets you integrate app content into Safari or send web data back to the app, enabling a unified experience for a web version and a native version of an app. Note. Previously, Safari extensions provided the communication ...
2 Then, we can call this wherever we want to open a URL. openURL environment value. Check whether the URL can be open . URL that we can use in openURL isn't limited to website URLs. You can use this to open a custom URL scheme, e.g., open the Google map app (comgooglemaps://).
When we want to open web content in UIKit, we can do it in three ways. Open the Safari app. This will direct users out of your app to the Safari app. Using SFSafariViewController. This is a built-in browser that gives you the same experience as the Safari app, but you can open it within your app. Using WKWebView. This is the most flexible one ...
In the body of ContentView, we'll change the text Hello, world to Open Design+Code in Safari. // ContentView.swift Text ("Open Design+Code in Safari"). padding () ... Build Quick Apps with SwiftUI. Apply your Swift and SwiftUI knowledge by building real, quick and various applications from scratch. 11 hrs.
There's 2 ways of opening a link with SwiftUI, on is using Link and Other one is using openURL environment key. Let's start with the first option using Link: Link's struct receives a title ...
If you want the user to exit your app and show a website in Safari, it's just one line of code in Swift.For more answers and solutions to common Swift proble...
Support universal links. Take the following steps to support universal links: Create a two-way association between your app and your website and specify the URLs that your app handles, as described in Supporting associated domains. With universal links, users open your app when they click links to your website within Safari and WKWebView, and ...
Updated for Xcode 15. When your user taps a URL shown inside a SwiftUI Text or Link view, it will open in Safari by default. However, you can customize this behavior by replacing the openURL environment key - you might want to handle the link entirely, or perhaps pass it back to the system to open once your custom action completes.. For example, this code adjusts both a Link and a Text view ...
The system provides a default open URL action with behavior that depends on the contents of the URL. For example, the default action opens a Universal Link in the associated app if possible, or in the user's default web browser if not. You can also set a custom action using the environment(_: _:) view modifier.
And there must be a way to call local browser through swift code, because the in app 'WeChat', when we click a link in that app, the system will open a safari browser and show the content of the link. My question is: how? Thanks for your help, Best Regards. Yours, Alex.Zhang. macOS
Lions Have Sex on Top of Safari Truck Full of People, Wild Video Cut Into Kim Kardashian's New Pink 'Do And See More Hot Stars Rockin' Pink On Top! Travis Kelce Covers Taylor Swift's Arm With ...
Taylor Swift and Travis Kelce got all dolled up and loved up Saturday evening ... as they attended a gala held by Patrick Mahomes' charity.
7. With JavaScript, you can open a URL in a specific browser tab. How do you accomplish the same thing with Swift from an iOS app, i.e., open URL in Safari/Chrome in a specific tab. The UIApplication open function doesn't list information about the options parameter, which seems like it might let you specify a named tab in which to open the URL.
Safari Panzer Battle is an adrenaline-fueled action combat game set in the wild. Players control various animals, utilizing skill and strategy to engage in intense shootouts. Mighty elephants, robust rhinos, and swift cheetahs each bring their unique abilities and weapons to the battlefield. Players…
Forget anti-heroes ... a grandma in New Mexico is a full-blown hero after shooting an intruder who was on the run from cops -- right in the middle of Taylor Swift's "Eras" flick!
Bloomberg's Mark Gurman today said that iOS 18 will "overhaul" many of Apple's built-in apps, including Notes, Mail, Photos, and Fitness. Gurman did not reveal any specific new features planned ...
American lawmakers have tried for years to ban TikTok, concerned that the video app's links to China pose a national security risk. Sapna Maheshwari, a technology reporter for The Times ...
1. The file is likely sandboxed and not accessible. Depending what it is, you could always run a local web server in your app which Safari can access. - Darren. Jul 17, 2022 at 16:07.
Leah McSweeney's sober dating app, Club Pillar, hosted its first in-person event with artistes of abstinence including Cat Marnell, TikTok star Kelsey Soles and McSweeney herself all on the scene.
Check out the clip ... Travis surprises the whole crowd with his appearance -- shrieks and gasps follow the man onstage, and -- while he leaves the DJing to the professionals -- he certainly gets ...
We've successfully integrated deep linking into our iOS application using Universal Links. However, when users click on a link shared in an Instagram story, we currently redirect them to our website instead of opening the app. Our client prefers a dynamic approach: opening the app if it's installed and redirecting to the website if it's not.
16. Simple solution using SFSafariViewController which available in a separate package. import SafariServices. Swift 4, iOS 9.0+. let url = URL(string: "your URL here") let vc = SFSafariViewController(url: url) present(vc, animated: true) If you need more control - use Configuration parameter ( Swift 4, iOS 11.0+ ): Example:
download the app App Store Google Play contact; ... Lions Have Sex on Top of Safari Truck Full of People, Wild Video ... Travis Kelce Covers Taylor Swift's Arm With Kisses At Las Vegas Gala.