

Fixing Focus for Safari

In 2008, Apple’s Safari team had a problem: web developers had noticed that links, buttons, and several input elements were not matching the :focus selector when clicked. Similarly, clicking on those elements did not fire a focus or focusin event. The developers dutifully submitted bugs against Webkit and were waiting for help.
That help never came.
The reason is that macOS didn’t transfer focus to operating system and application buttons when they were clicked and the stakeholders decided to be consistent with the operating system instead of with their competitors’ browsers. The discussions have lasted 13 years and are still ongoing, but the team at Apple is adamant: buttons, links, and non-text inputs won’t get focus.
Helpfully, the team agreed that a workaround would be to implement :focus-visible , the CSS selector that matches elements that are awaiting input; they are focused but not activated. Clicking a button or link both focuses and activates it, but tabbing into them will match this focus-only selector. This partial solution would indeed help, but it stalled in April of 2021, three years after it was started and 11 years after it was suggested.
This failure has started to snowball. Safari introduced :focus-within in early 2017, beating most of their competitors to market with this newly standardized pseudo selector. But it wouldn’t match any container when a button, link, or non-text input was clicked. In fact, if the user were focused on a text input (matching :focus-within selector for its container) and then clicked on one of those troublesome elements, the focus left the container entirely.
Edit (8/29/2022) Safari has implemented :focus-visible in version 15.4. The bugs described in this article for :focus still exist, though. Clicking any problematic element does not fire focus events, and does not match the :focus or :focus-within selectors.
It’s clear that Apple’s Webkit/Safari team are not going to fix this issue. There is an excellent polyfill available for :focus-visible from the WICG. There is a good polyfill available for :focus-within as well. But I have not found a polyfill for :focus , so I wrote one:
Edit (1/22/2024) I created a test suite and support matrix for all the different ways that an element can be made click focusable or rendered unfocusable (there are 108 test cases so far!). Safari is by far the least supported but even Firefox and Chrome/Edge had failing tests. Notably, no browser properly handles click focusability on the generated control elements for <audio> and <video> elements. Building out this test suite also showed that the previous polyfill I made was insufficient for all of the use-cases. I wrote a new, more comprehensive polyfill here .
Polyfill Breakdown
The heart of this polyfill is the Element.focus() method call (on line #112 in the gist above) that dispatches the focus and focusin events. Once the browser sees these events associated with an element, this element matches the :focus selector.
There are a few events we could listen to in order to dispatch that focus event when the element is clicked: mousedown , mouseup , and click .
A note on events If you’re not familiar with how events work in the browser, there are two phases. The capturing phase starts at the topmost element, <html> , and proceeds down through the DOM towards the element that was clicked. The bubbling phase begins after the capture phase ends, and it starts at the lowermost element that was clicked and proceeds upwards (bubbles) toward the HTML element. Any node in this pathway can attach an event listener that is triggered when the event hits that node.
Any of these event listeners can stop the event from continuing and attach listeners for following events that could prevent them from firing. This is important. We don’t want some other event listener to prevent our listener from firing. That means we want to attach to the earliest event, mousedown , and during the capturing phase. This is done on line #81 in the gist above.
However, Element.focus() fires synchronously, meaning that the focus and focusin events would fire before the blur and focusout events. The correct order of events is:
- mousedown [targeting the clicked element]
- blur [targeting the previously active element]
- focusout [targeting the previously active element]
- focus [targeting the clicked element]
- focusin [targeting the clicked element]
- mouseup [targeting the clicked element]
- click [targeting the clicked element]
We can use a zero second setTimeout call (line #56 in the gist above) to enqueue the Element.focus() call after all of the currently queued events. This moves our forced focus event after the blur and focusout events, but this also moves it after the mouseup and click events, which is not ideal.
To ensure that the mouseup and click events fire after our forced focus event, we need to capture and resend them. We don’t want to capture every mouseup and click event, so we need to add the listeners only when we are going to force a focus event (line #41–42) and remove them when we’re done (line #58–59). Lastly, we need to re-dispatch those events with all the same data as they originally had, such as mouse position (line #67).
Lastly, we need to only do all of this work in Safari (see line #80) and only when the unsupported elements are clicked. The wonderful folks at allyjs.io have provided a very useful test document for checking what elements get focus. After comparing the results of clicking each of these elements in different browsers, I found the following elements needed this polyfill:
- anchor links with href attribute
- non-disabled buttons
- non-disabled textarea
- non-disabled inputs of type button , reset , checkbox , radio , and submit
- non-interactive elements (button, a, input, textarea, select) that have a tabindex with a numeric value
- audio elements
- video elements with controls attribute
The function isPolyfilledFocusEvent (line #22–36) checks for these cases.
I hope this helps.
And I hope Safari supports this stuff natively sometime.
EDIT (15/1/2022): Real-world production use of the polyfill has revealed two bugs. First, the redispatched events should be dispatched from the event targets rather than from the document. Events not fired from the previous target are stripped of their target property and do not trigger default behaviors like navigating on link clicks.
Second, the capturing of possible out-of-order mouseup and click events needs to be handled differently. By adding the listeners dynamically, they were being added after any listeners that were added after the polyfill runs. This means that the other listeners would trigger first before the event being captured, and then they would trigger again when the event was redispatched. The solution is to add the polyfill’s listeners for mouseup and click immediately, and toggle a capturing flag on and off instead of attaching and removing listeners.
EDIT (21/1/2022): More production use has revealed another bug. Clicking on an already focused element should not fire another focus (or focusin) event. However, the polyfill was blindly firing a focus event on every click, or mousedown, of an affected element. To prevent this from happening, we check if the event target element is the same as the document’s active element before proceeding with the polyfilled focus behavior.
A side effect of not firing focus on every click is that Safari returns focus to the body element if it decides that the target element isn’t focusable. To stop this, we call preventDefault() on the mousedown handler. (How did I know that would work? I didn’t. It took some trial and error to find out when and how Safari was moving focus to the body.)
EDIT (23/1/2022): Clicks on label elements should redirect focus to the labeled element. In order to detect that a click was occurring on a label, we have two choices: (1) on every mousedown event that we are listening to, we could climb the DOM tree all the way to the body element to see if the event triggered on an element in a label, or (2) detect when the pointer is inside a label before the mousedown event. Option 1 would work but it would be very expensive, especially on large pages. For option 2, we had to find how to detect the user was inside a label before the mousedown event fired.
The event mouseenter fires before the mousedown event (even on touch devices!) and it fires for each element that the mousedown event would bubble through. That means that each element has a mouseenter (and mouseleave ) event that it is the target of. (Since we’re using delegated listeners, we cannot rely on currentTarget because that would always be null.)
We added a function to get the redirected focus target when the user is inside a label element, and we use that element to detect if it already has focus and to focus on it if not.
EDIT (17/2/2022): We needed to account for possibly nested children in focusable elements that accept children, such as buttons, anchor tags or arbitrary elements with a tabindex attribute. Since we were traversing the DOM looking for focusable parents anyway, we decided to remove the mouseenter and mouseleave listeners we were using for discovering focusable label elements and fold that use case into the new strategy. We also discovered a bug in our check for the tabindex attribute: the HTMLElement property tabIndex reports a value of -1 if the attribute has not been explicitly set. The solution is to use .getAttribute('tabindex') to determine if the value has been explicitly set.
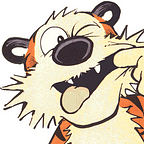
Written by Nick Gard
Web Developer, Oregonian, husband
More from Nick Gard and ITNEXT
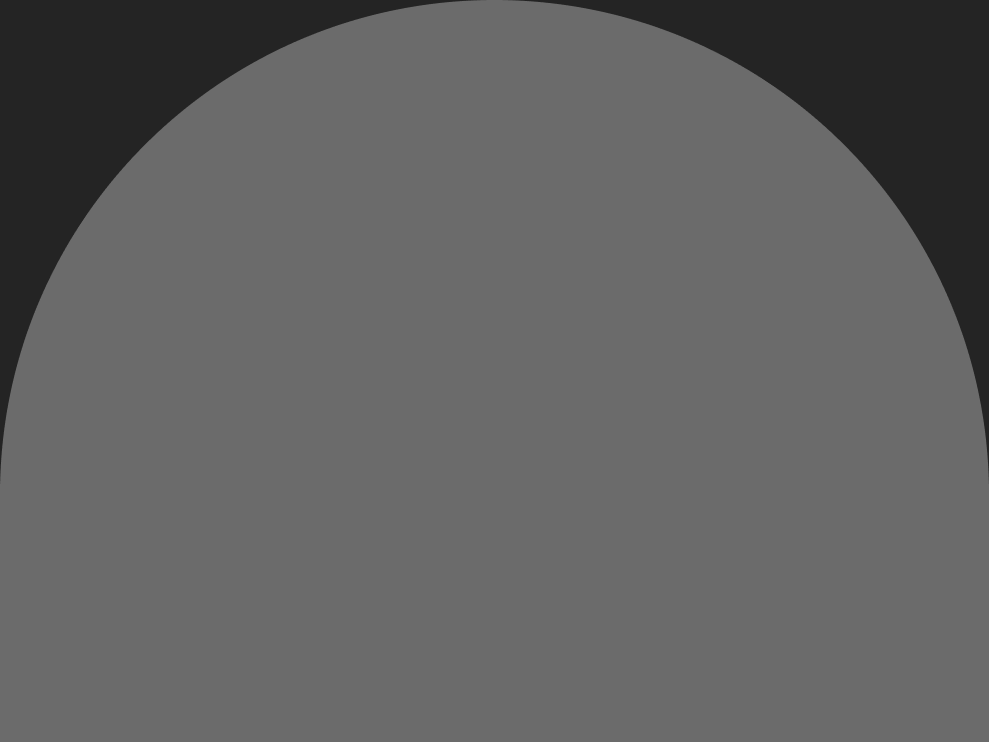
Tuples in JavaScript
No, javascript doesn’t have tuples. but with array destructuring, we can pretend it does in languages where tuples actually exist, their….

Niels Cautaerts
Containers: has the pendulum swung too far?
Containerization has revolutionized the software industry, but using them blindly for everything without considering their drawbacks or….

10 CLI Tools That Made the Biggest Impact
On transforming my terminal-based workflow.
Boolean Logic in JavaScript
Part 1: boolean operators & truth tables, recommended from medium.

Alexander Nguyen
Level Up Coding
The resume that got a software engineer a $300,000 job at Google.
1-page. well-formatted..

Today I Interviewed for a Lead Front-End Role
And they asked me a couple of tough questions.

General Coding Knowledge

Stories to Help You Grow as a Software Developer
Coding & Development

Tech & Tools

Coding Beauty
5 amazing new JavaScript features in ES15 (2024)
5 juicy es15 features with new functionality for cleaner and shorter javascript code in 2024..
JavaScript in Plain English
Microsoft is ditching React
Here’s why microsoft considers react a mistake for edge..
Minko Gechev
Angular Blog
Angular v18 is now available!
Today we are excited to share the next milestone in the evolution of angular over the past three releases we’ve introduced a lot of new….
Async Await Is The Worst Thing To Happen To Programming
I recently saw this meme about async and await..
Text to speech
Focus Mode Not Working? Try These 8 Troubleshooting Tips
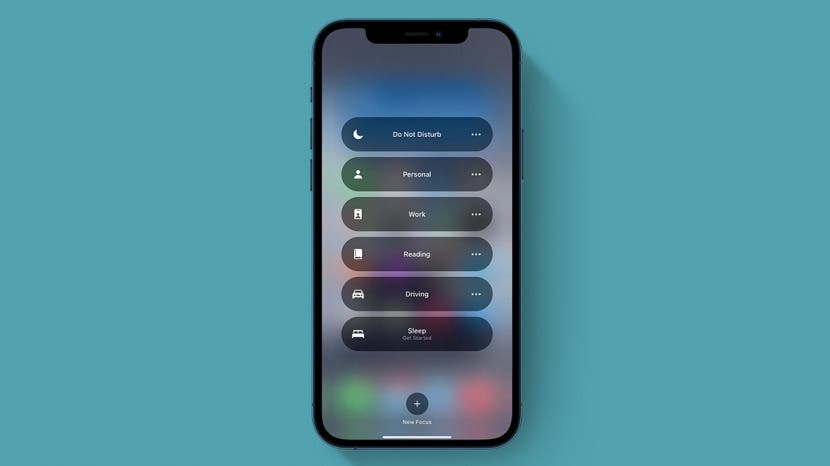
Focus in iOS 15 lets you customize many different modes to determine when you get notifications and from whom. But what happens when Focus mode doesn't work as intended: letting notifications through, not getting notifications you wanted, not showing notifications when your phone is unlocked. Let's go over what you can do about these common Focus mode issues.
Related: How to Retrieve Deleted Text Messages on iPhone
Why Am I Getting Notifications on Other Devices?
Why am i getting notifications from some apps, is the wrong focus mode on, why am i still getting calls from some people, why am i getting "time sensitive" notifications, why are repeated calls getting through, why am i getting silenced notifications on my lock screen, focus mode isn't on when it's supposed to be.
If you are still getting notifications on other devices when Focus mode is on, you may have turned off Share Across Devices . This setting, when on, makes sure your Focus settings are shared across any Apple devices you've signed into using your Apple ID. To learn more about new iOS 15 features, sign up for our Tip of the Day newsletter.
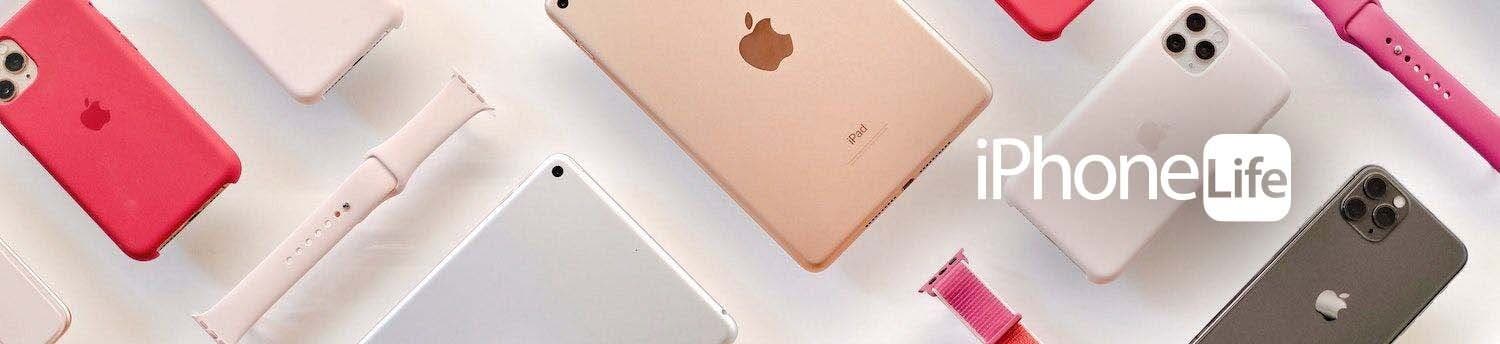
If you don't want Focus mode settings to be shared across all your Apple devices, you can turn this setting off.
When you set up a new Focus mode, your iPhone may suggest apps to "whitelist"—that is, apps that can still send you notifications even when Focus mode is on. If you did not remove those apps at setup or later, they'll still be able to send you notifications. You may have also whitelisted apps when you set up your Focus mode that you no longer want to see notifications for. Here's how to remove apps from your Focus mode whitelist:
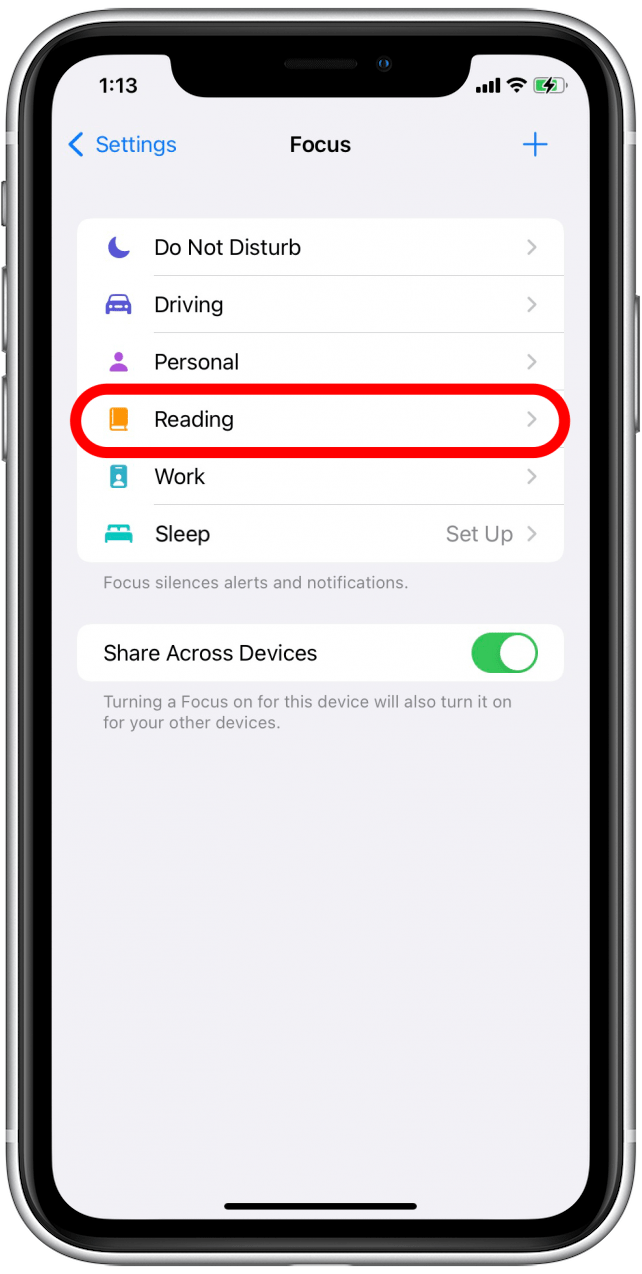
You may need to repeat this for all Focus modes you're still receiving app notifications in.
While having multiple different, customizable Focus modes gives you more flexibility, it can also be a little confusing. If you're getting unexpected notifications, you might want to check to see if you have the right Focus mode on. To check to see if you have the correct Focus mode on:
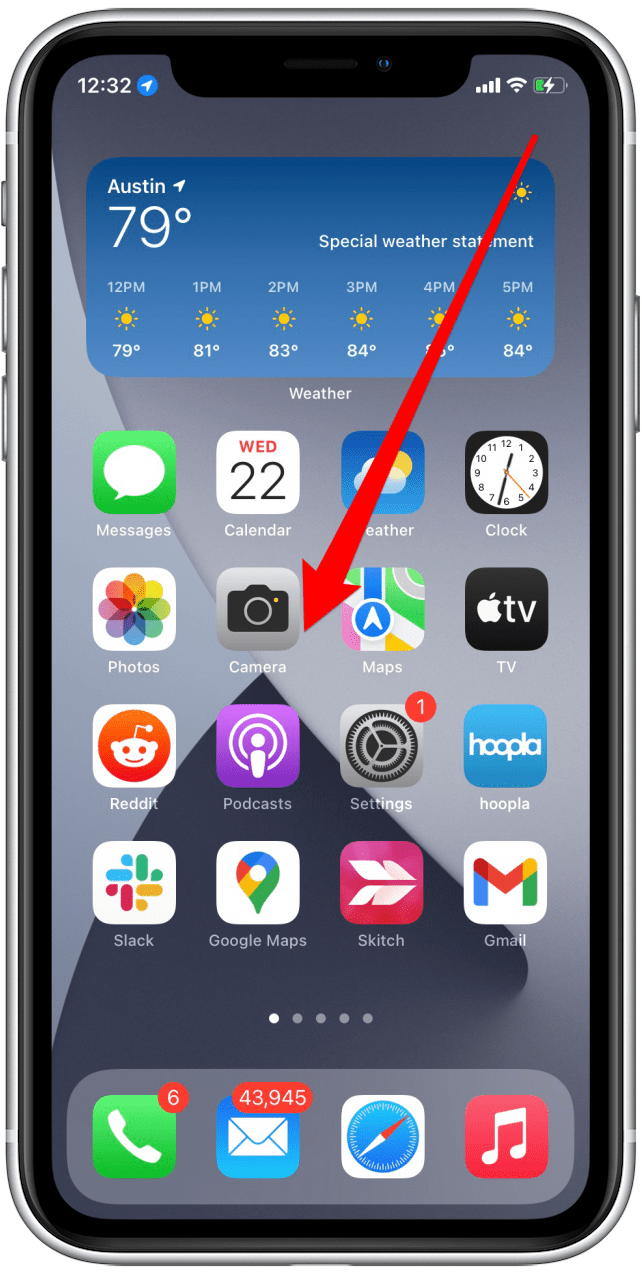
From there, you can see and customize which notifications are allowed in this Focus mode and add or change automations and other options.
If you did not add Allowed People while setting up your Focus mode, you still might have allowed calls from your Favorites. You might not have even noticed you were doing it:
Here's how to turn off calls from Favorites in Focus.
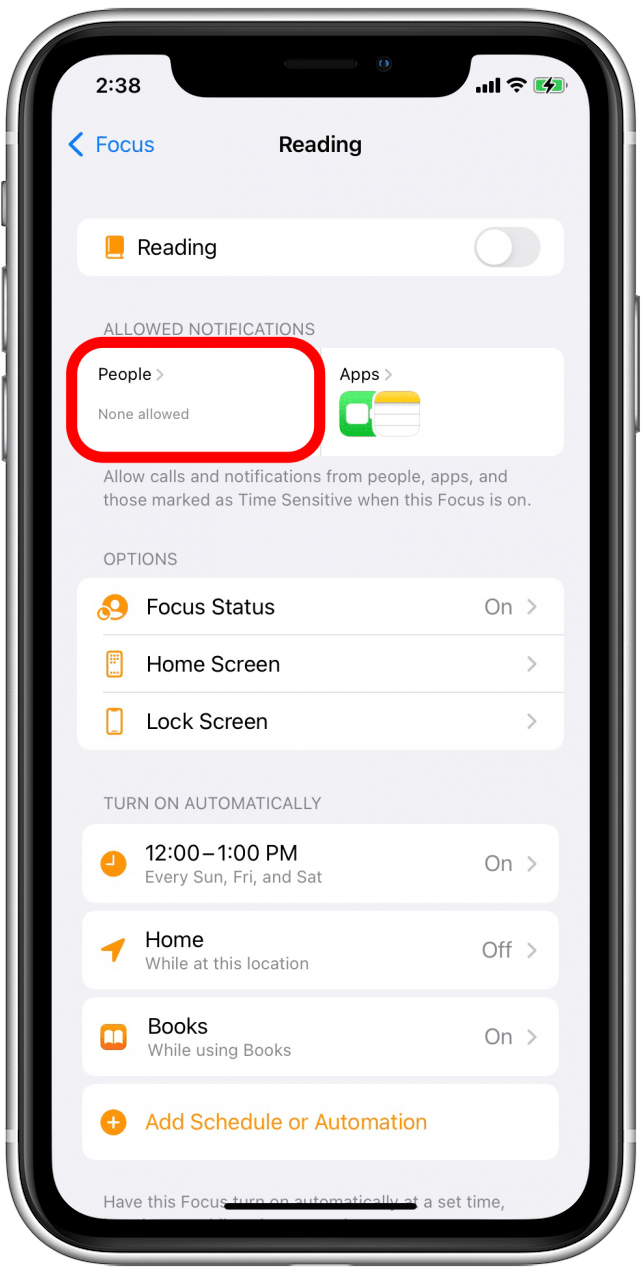
If your Focus mode is not working properly because it's still sending you notifications you didn't ask for, you might have allowed Time Sensitive notifications during setup. Time Sensitive notifications are notifications that could impact you directly or need your immediate attention, like when a package gets delivered or there's been suspicious activity on your bank account.
Because these notifications are often of critical importance, be cautious about disabling them in Focus mode. Apple has directed developers to be very judicious with what types of notifications they deem time sensitive, so you should not receive low-priority notifications this way. However, developers are able to set which notifications can break through Focus mode as time sensitive, so if you are getting less important notifications while in Focus mode, here's how you can turn Time Sensitive notifications off:
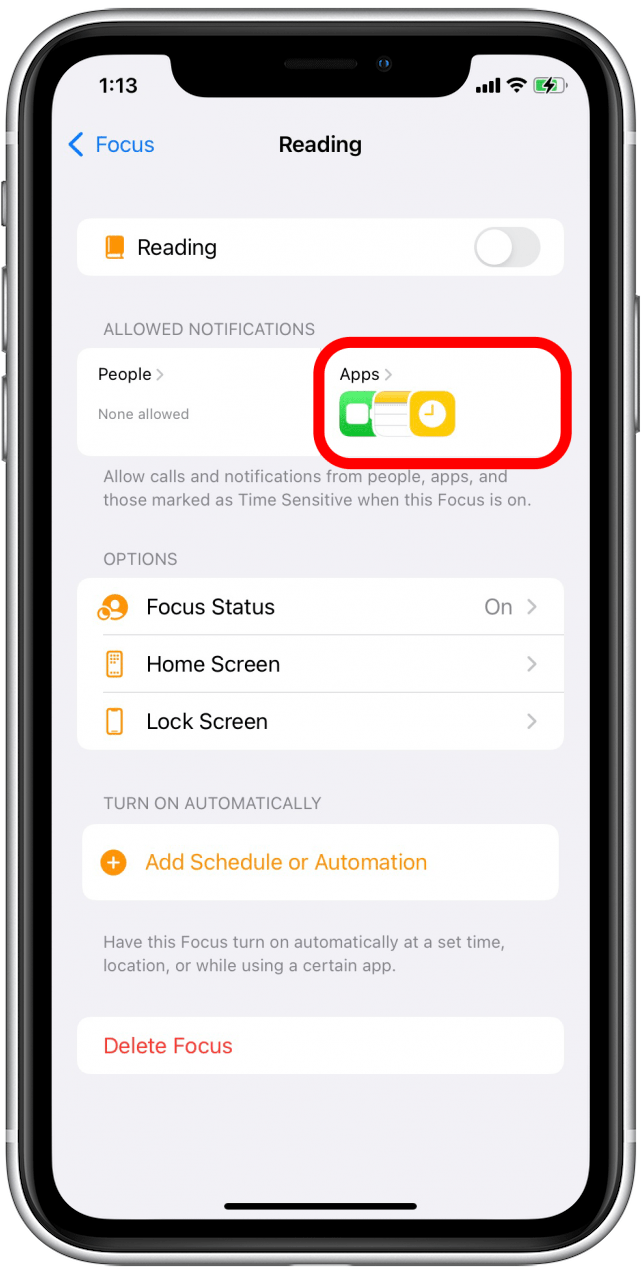
Similar to how Apple built a way for Time Sensitive notifications to get through Focus mode, it also built a safety valve in case someone needs to contact you in an emergency. If you have this setting enabled, when someone calls you multiple times within three minutes, their call will break through Focus mode. If you have contacts who tend to have a liberal definition of the term "emergency," you can disable this setting as well.
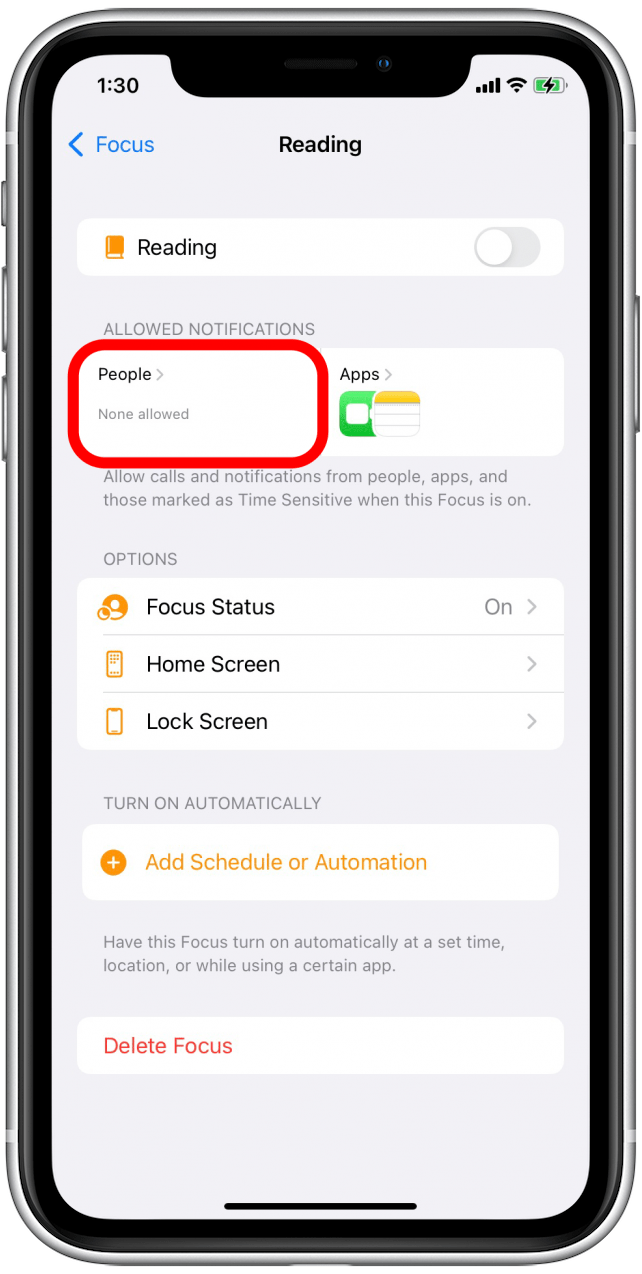
If you are seeing notifications on your Lock Screen but not hearing your notification sound when your phone is locked and in Focus mode, you may have allowed Show On Lock Screen in your Focus mode settings.
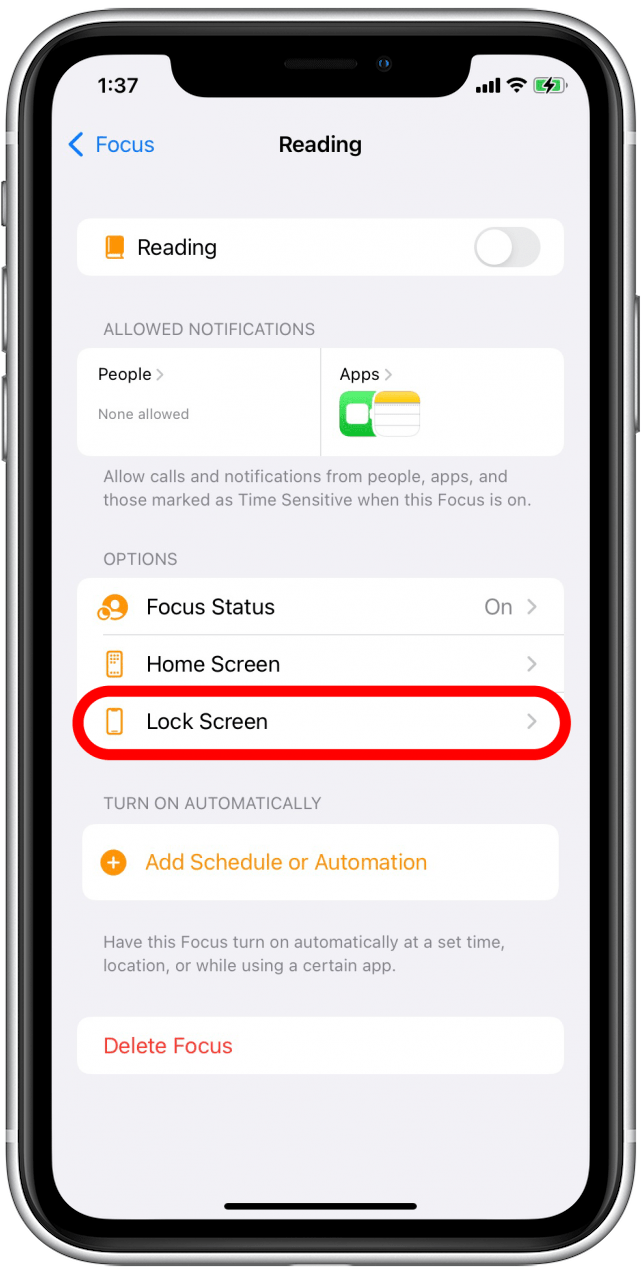
I hope that this guide has helped fix the issues you were having with Focus mode. If you are experiencing other Focus mode problems, you may need to restart or reset your iPhone or contact Apple Support for more guidance. If you have a Focus mode issue you don't see here, let us know about it in the comments. Next, find out how to set up profiles in Safari .
Author Details
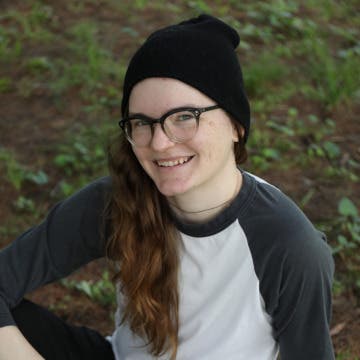
Elisabeth Garry
Elisabeth Garry is an Associate Editor for iPhone Life. Formerly of Gartner and Software Advice, they have six years of experience writing about technology for everyday users, specializing in iPhones, HomePods, and Apple TV. As a former college writing instructor, they are passionate about effective, accessible communication, which is perhaps why they love helping readers master the strongest communication tools they have available: their iPhones. They have a degree in Russian Literature and Language from Reed College.
When they’re not writing for iPhone Life, they’re reading about maritime disasters, writing fiction, rock climbing, or walking their adorable dog, Moosh.
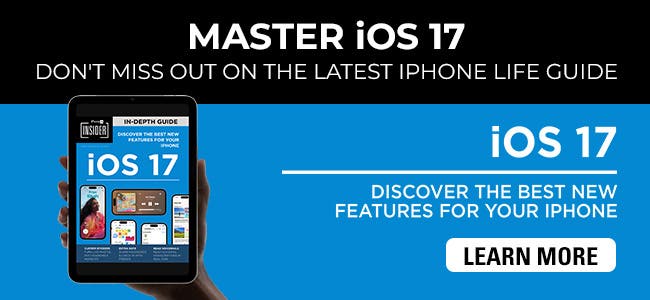
Featured Products
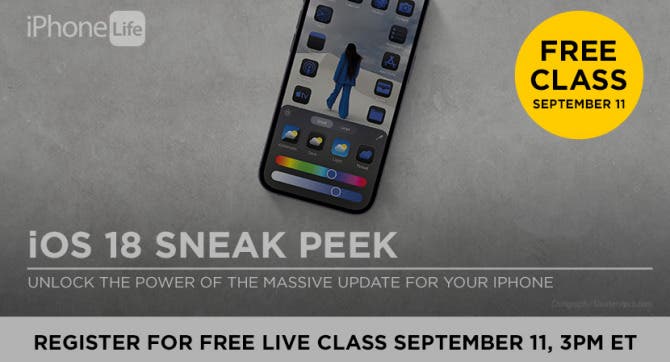
Apple is set to release iOS 18 in a couple of weeks. That's why I'm excited to invite you to our free iOS 18 Sneak Peek Class on Wednesday, September 11 at 3 p.m. ET.
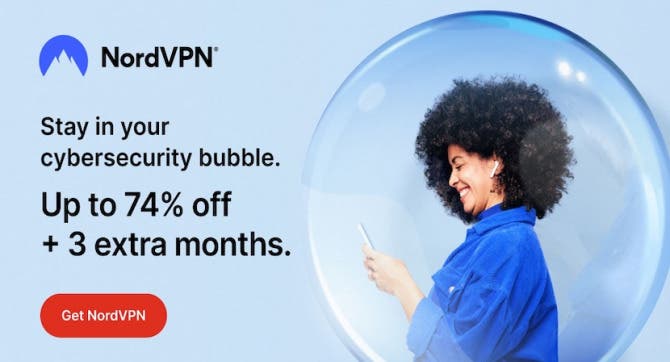
We have tried several VPN services over the years. NordVPN is the only one that doesn't slow down our internet speed and also provides useful security features like malware scanning.
Right now NordVPN is offering 73% off 2-year plans + 3 months exta with every 2-year plan (starting from $3.09/month).
Most Popular
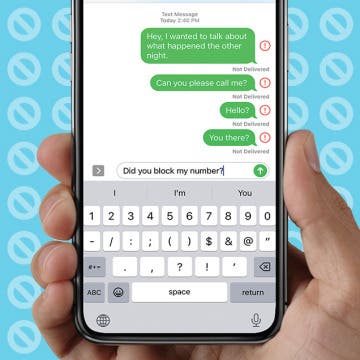
How to Tell If Someone Blocked Your Number on iPhone
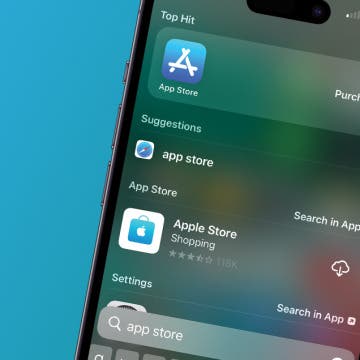
App Store Missing on iPhone? How To Get It Back
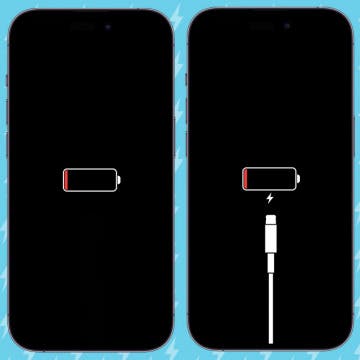
How to Tell If a Dead iPhone Is Charging
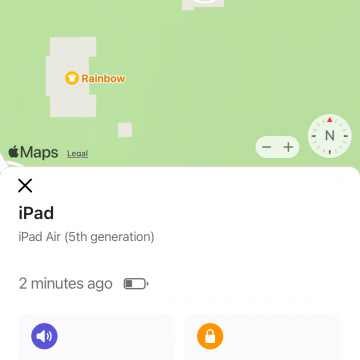
How To Find My iPhone From Another iPhone
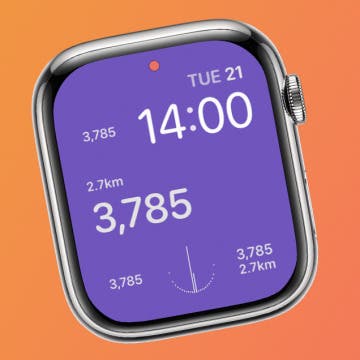
Step Counter: How To Show Steps on Apple Watch Face
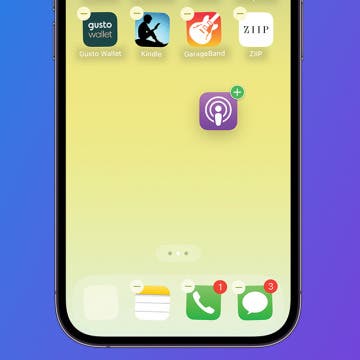
How To Get an App Back on Your Home Screen
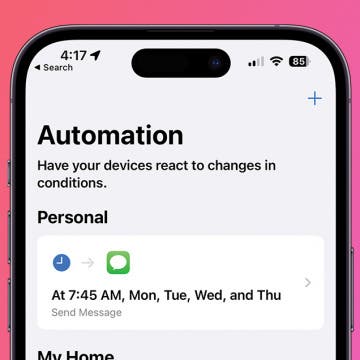
How to Schedule a Text Message on iPhone
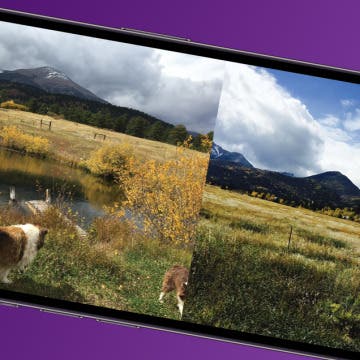
How To Put Two Pictures Together on iPhone
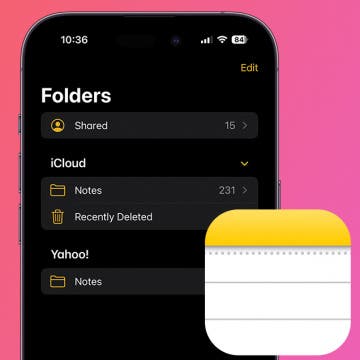
iPhone Notes Disappeared? Recover the App & Lost Notes
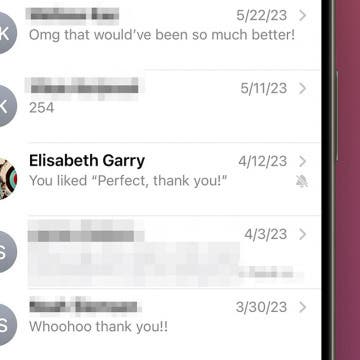
How to Silence a Contact on iPhone
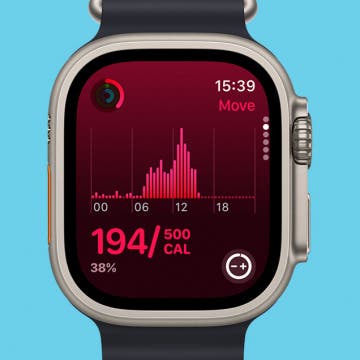
How Accurate Is Apple Watch Calories?
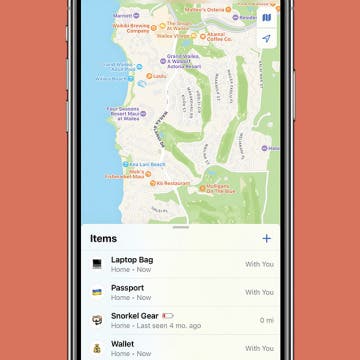
How to Refresh AirTag Location Manually & More Often
Featured articles.
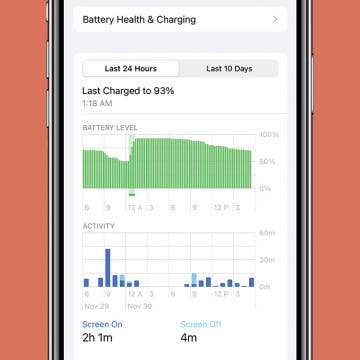
Why Is My iPhone Battery Draining So Fast? 13 Easy Fixes!
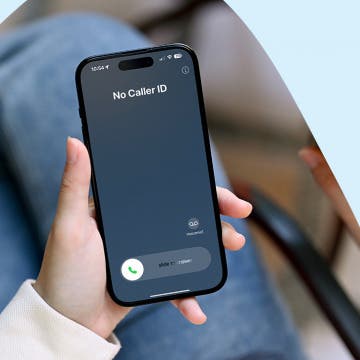
Identify Mystery Numbers: How to Find No Caller ID on iPhone
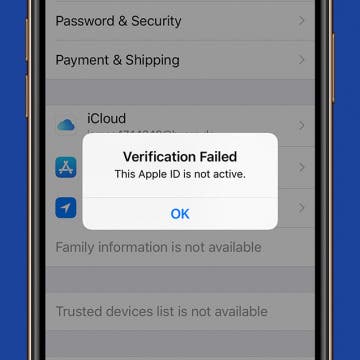
Apple ID Not Active? Here’s the Fix!
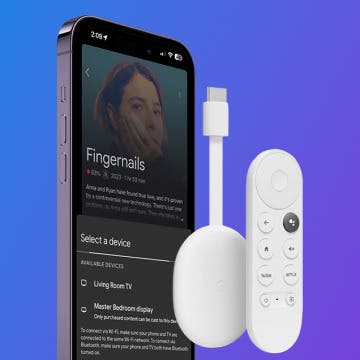
How to Cast Apple TV to Chromecast for Easy Viewing
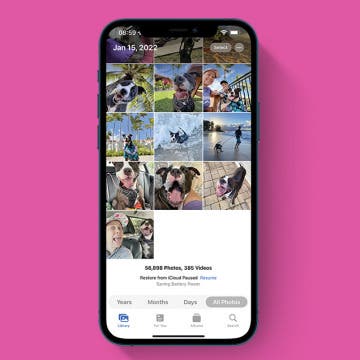
Fix Photos Not Uploading to iCloud Once & for All (iOS 17)
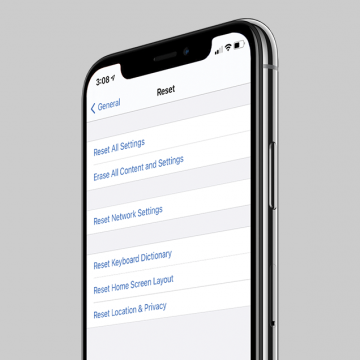
There Was an Error Connecting to the Apple ID Server: Fixed
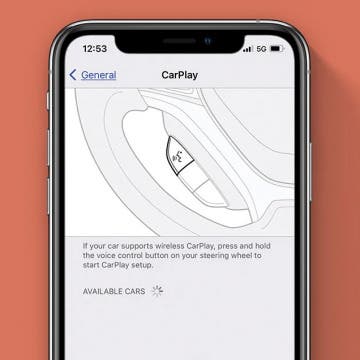
iPhone Charging but CarPlay Not Working? Here's the Fix!
Check out our sponsors.
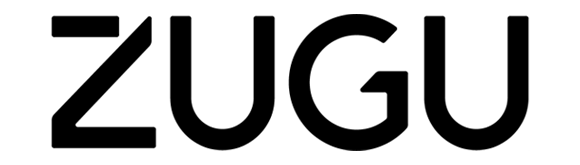
- Each email reveals new things you can do with your phone (and other devices) with easy-to-follow screenshots.
- Enter your email to get your first tip immediately!

10 Ways to Fix Focus Not Working on iPhone
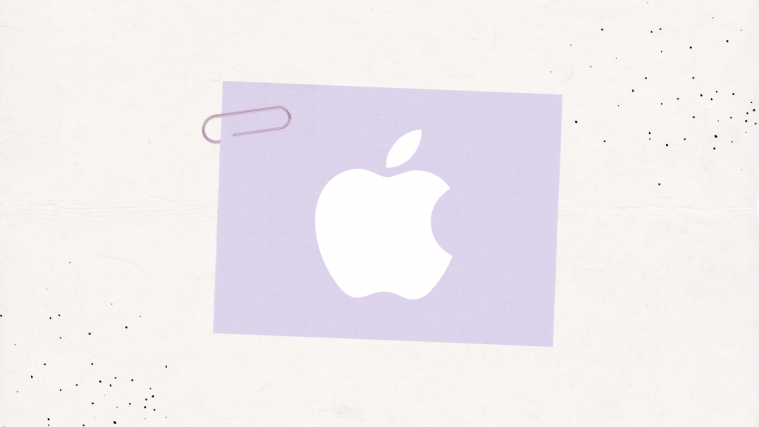
Apple offers several ways to improve your workflow and efficiency and the Focus feature inside iOS is one of them. Focus allows users to create customized routines for different schedules so that they can avoid distractions from apps and people when they’re busy.
If you are facing using the Focus feature on your iPhone, the following post should help you resolve them.
How to fix the Focus not working issue on iPhone [10 fixes]
If you’re unable to use Focus on your iPhone or if you’re having trouble getting it to work properly, you can try one of the fixes below to try and fix it.
Fix 1: Make sure you have not whitelisted the app
If an app can bypass your focus mode then you should go ahead and check if the app hasn’t been whitelisted by you by mistake. In case the app is whitelisted, it won’t be affected by your current Focus mode. Use the guide below to check for whitelisted apps.
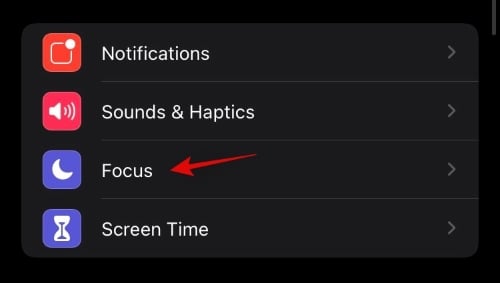
Once the app has been removed from your whitelist, it won’t be able to bypass your Focus mode anymore.
Fix 2: Make sure you have not whitelisted someone
Another thing to check would be your contact’s whitelist. If you have someone who can get through to you be it through calls or messages by bypassing your current Focus mode, then it is likely that the contact has been whitelisted. Use the guide below to remove the contact from your whitelist.
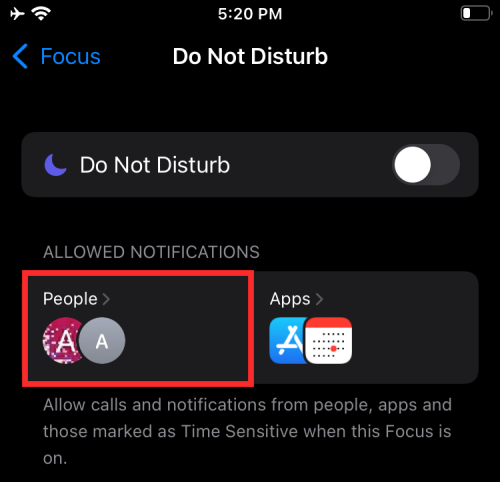
And that’s it! The concerned contact will now be removed from the whitelist and they will no longer be able to bypass your focus mode.
Fix 3: Make sure that another focus mode is not turned on
This might be a rare case scenario but in case an app or contact can bypass your Focus mode, then it would be a good idea to ensure that the correct focus mode is turned on.
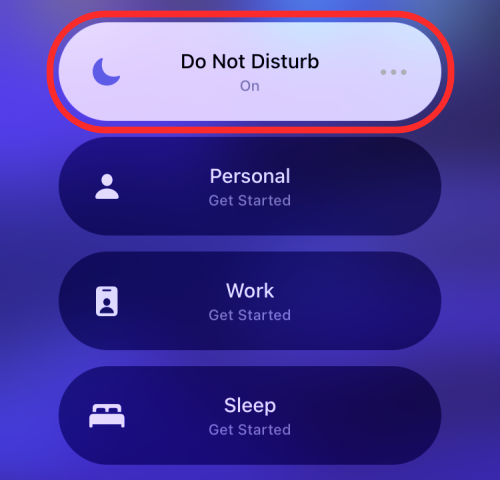
- If a different focus mode is activated then simply tap on the desired one to activate it from the list instead.
Fix 4: Make sure your focus sync is disabled
If you own multiple Apple devices then this is likely the cause of your issue. Apple has always held its ecosystem in divinity, be it restricting devices to the ecosystem or introducing new features that help form the ecosystem in the first place. As you might expect, Focus modes are also tied into the Apple ecosystem out of the box. This is achieved by syncing your current Focus mode across all your Apple devices associated with your Apple ID. This helps avoid distractions no matter how many devices you own with a simple tap. However, if you find that focus mode is not working as intended or a different one is always enabled on your device, then chances are that it is syncing to your other devices associated with your Apple ID. This can especially be the case if the other device is being used by someone else. You can disable cloud sync for Focus modes easily, by using the guide below.
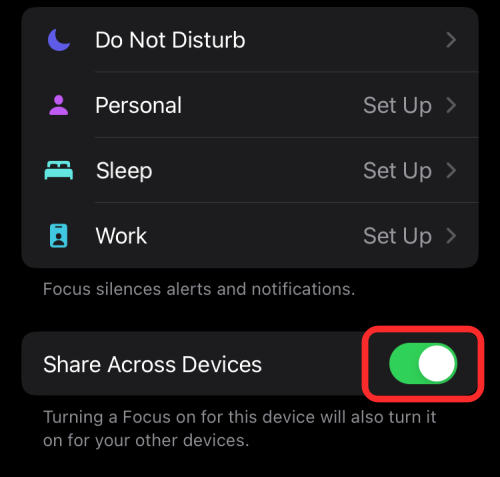
And that’s it! Your current device’s focus mode will no longer be synced across all your Apple devices.

Fix 5: Turn off ‘Repeated calls’ from getting through
If you have an issue with calls getting through your focus mode then it might be due to repeated calls being enabled by default in every focus mode. When this setting is turned on, a contact is let through if they try to get in touch with you multiple times regardless of the fact if they are whitelisted or not. This setting helps you get in touch with your loved ones in case of emergencies when you have Focus mode turned on. However, if you have contacts that seem to misuse this feature then you can turn it off using the guide below.
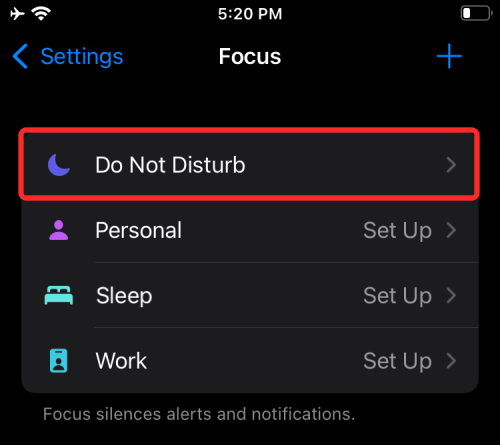
And that’s it! Repeated calls will now be disabled and they will no longer be let through to you in any Focus modes.
Fix 6: Turn off time-sensitive notifications
Apple uses Siri and machine learning to determine time-sensitive notifications and let them through regardless of your Focus mode. These notifications can be OTPs, account statements, amber alerts, important messages from emergency services, and more. This also includes reminders, pick-up notifications, and other alerts from important apps. If however, these notifications seem to annoy you and you are trying to avoid them through your Focus mode then you can turn them off in your settings using the guide below.
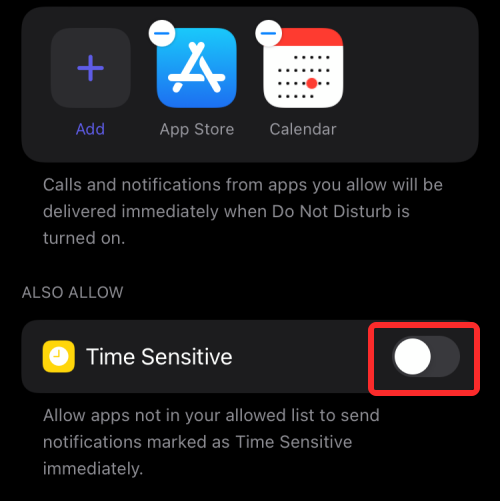
And that’s it! The time-sensitive notifications will no longer be able to bypass your Focus mode.
Fix 7: Make sure the Focus schedule time is correct
Are you using scheduled focus modes? Then chances are that some discrepancies in your schedule might be causing the Focus mode to be activated or deactivated at the wrong time. Verify your schedule for the focus mode to ensure that it is not getting turned off or on at the wrong time using the guide below.
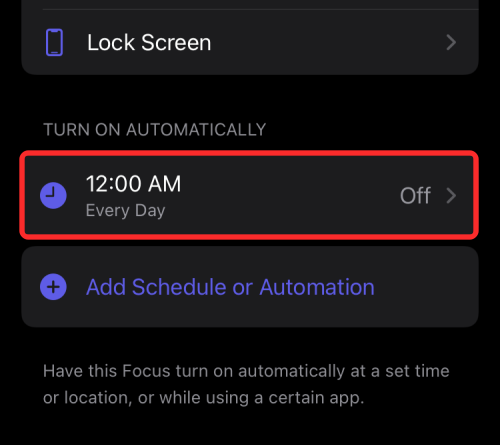
In case everything has been set correctly on your device then you can try one of the fixes below to fix the Focus mode on your device.
Fix 8: Make sure the Messages app is enabled inside privacy settings
If you wish to share your Focus Status with others through the Messages app, you will need to manually enable it inside iOS’ privacy settings.
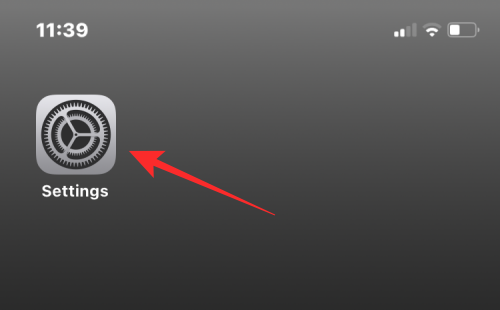
Fix 9: Ensure that the silent switch is not enabled
This is a long-standing iPhone issue. Have you tried everything but something is still keeping your phone silent? Well, iPhones have faced this issue for a long while but it is no longstanding bug or hardware issue. Have you checked the silent switch? In many cases, the silent switch is the cause of silence issues on an iPhone. Simply check the silent switch by toggling it and you should get a notification at the top sharing the current status of your iOS device. However, if your device wasn’t silenced by the silent switch then you can move to the last few fixes below.
Fix 10: Last Resort: Reset or Re-install
Well if the Focus mode doesn’t seem to work as intended, then the last thing you can do is try to reset your device so that there’s a fresh new copy of iOS on your iPhone. A clean install should help solve any background conflicts that might be stopping the Focus mode from working as intended.
To reset your iPhone, open the Settings app on your device and go to General > Transfer or Reset iPhone > Reset .
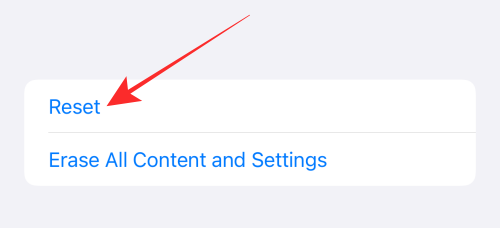
We hope you were able to easily fix your Focus mode issues using one of the fixes above. If you still face issues, feel free to drop us a message in the comments section below.
Spectroscopic collection of human cells trying to pave a path via words.
You may also like
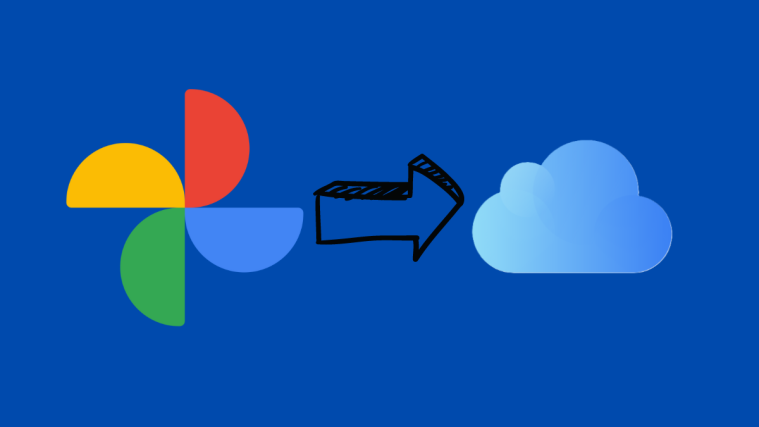
How to Transfer Google Photos to iCloud

All iPhone Models Are Cheaper by up to Rs 5,900
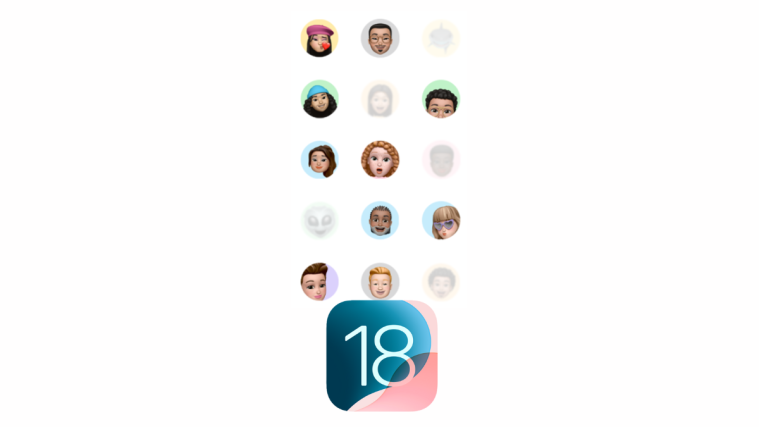
3 Ways to Control How You Share Contacts With Apps in iOS 18
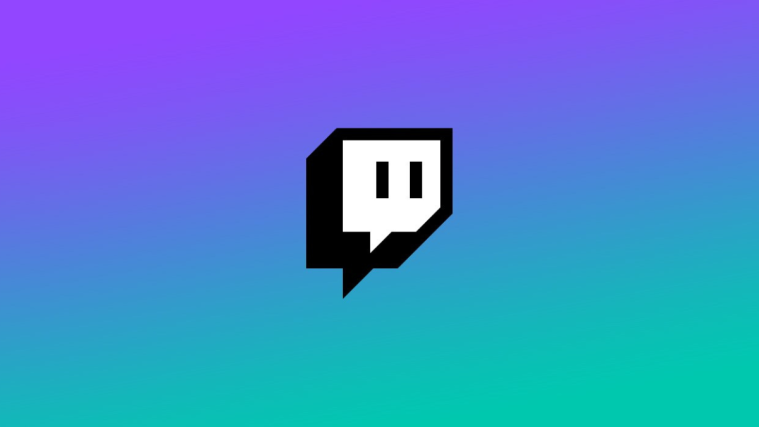
Twitch Subscribers on Mobile Will Soon Have to Pay More
11 comments.
Anyone else having an issue with Focus? For some reason, it won’t let me Share Across Devices because “Focus syncing requires an iCloud account.” However, I do have an iCloud account connected, and even if I had to connect it somewhere else, it’s been impossible to find. Also, under Focus Status, I can’t enable it because “”Share Across Devices” must be turned on to share your Focus status from this device.” However, it is on. I’ve been messing with this for about 30 minutes and I can’t seem to find a solution.
I have this same issue.
Good day, my issue it’s when i select focus and try to select my contact, the whole thing just freezes. Please to you have any idea on how to resolve this.?
I have another issue in iOS 15 after updating. I have two apple device. (iPhone 12 mini and iPad pro 12.9) I set the focus mode in my iPhone and sharing with my iPad.
My iPad is always at home. The focus mode location is determined to my iPad.
For example, my iPhone located at school and my iPhone located at home. The focus mode is not working in my iPhone.
So, I turn off the sharing between two device. But, it still not working.
It just working about my iPad location.
I have a school focus created and set to specific times while I’m in class and on campus. However, it’s still not allowing notifications or calls to come through when I’m at home outside of the school time frame and away from the location of campus.
Focus mode doesn’t notify people that I have it turned on… pulling out my hair trying to fix whatever bug is happening.
Same!!!! WTF!!!
I have the same issue – did you ever figure out a solution?
I have followed all these steps to get focus setup for Driving. Driving states it’s on, great. When someone texts me there is an automatic reply that is NOT being sent out. This use to work before this Focus was introduced. What the heck.
I have a problem with my focus where it doesn’t notify others that I’m on dnd and I can’t see when others on dnd when I and those people have the option on. Plus I went through everything with somebody to see if it was my setting and it wasn’t my share across devices is on and it’s on in messages I restarted my phone countless times and i every reset my setting but it’s still not there. In messages the option to share focus stratus I’d greyed out I cannot click it and I don’t know why .
my focus status is still not working and i tried everything here.
Comments are closed.
Input focus issue inside iframe
I currently developing a mobile website and found that Safari on iOS has some problems setting the focus on inputs when they are inside an iframe.
When in a page you have many inputs you can tap on a field and then use the next / previous buttons on the keyboard to navigate between the fields. When the focus move to another input Safari scroll automatically the page centering the field in the page. This is great and works well.
The problem is when the fields are inside a page of an iframe. In this case when you change focus from one field to another Safari has some kind of issue; the page “bounce” and the focused field is not centered in the page.
I have made a video of a simple page that has this issue. In the first part of the video the page without the iframe is loaded and the focus works correctly. In the second part the page with the iframe is loaded and the issue is visible.
http://www.dale1.ch/documents/safari_iframe_focus_issue.mov
The code of the first page (testinput.html) where the focus is correctly handled is this:
This is the code of the page that has the issue (testinput_iframe.html):
The issue is present in Safari on the iPhone, iPad and the xCode Simulator from version 8.4 to version 9.2.
Someone know if this is a bug? There is way to fix it in css or javascript?
Thanks and sorry for my english! 😉
I'm having the exact same problem and have not been able to find a solution. Have you filed a bug report?
Yes, I have filled a bug report and was marked as a duplicate but I encourage you to fill another one (more is better).
Would you please provide a link(s) to the bug report(s)?
Unfortunally bug reports are private and only visible to the person who create it.
fbsFrontEndDev, I really suggest you to open a new report, the more the better.
Just want to inform that the bug is present also on iOS 10 beta 1. 😟
I have the same problem on iOS 10.2.1, and I filled a bug for such. But based on this stack overflow, it appears to affect any element with a tabIndex which is not an INPUT element. http://stackoverflow.com/questions/40487279/calling-element-focus-in-iframe-scrolls-parent-page-to-random-position-in-safa
I am also having the same issue. I have a name and email field inside a iframe. When i load the HTML page i see the page render properly. Now when i click and type the name field it works properly. Now when i click on email field and start to type the cursor moves down and outside the input box. This is really weird and exactly same problem as this one. Have anyone find the solution for this? Any help is highly appreciable.
Apple, if you are reading, please fix this - it's been a known issue for a long time, and other browsers do not have this problem.
I am trying to roll out a new product that uses iframes for some fields, but this bug is going to cost extra time becaue we have to work around this. I have not found a workaround yet.

Try to just add touch-action: none; to input styles
Looks like no one’s replied in a while. To start the conversation again, simply ask a new question.
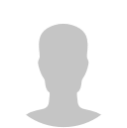
Is focus() broken in Safari?
MacBook Pro, Mac OS X (10.4.11)
Posted on Aug 29, 2009 12:56 AM
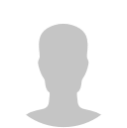
Posted on Aug 29, 2009 2:00 AM
Loading page content
Page content loaded
Aug 29, 2009 2:00 AM in response to Generic Nickname
Aug 29, 2009 12:33 PM in response to rabbitdog
Aug 30, 2009 6:39 PM in response to Generic Nickname
Aug 30, 2009 8:52 PM in response to rabbitdog
- a. Send us an email
- b. Anonymous form
- Buyer's Guide
- Upcoming Products
- Tips / Contact Us
- Podcast Instagram Facebook Twitter Mastodon YouTube Notifications RSS Newsletter
iOS 16: How to Limit Browsing to a Specific Safari Tab Group in Focus Mode
Apple in iOS 15 introduced Focus mode, an expansion of Do Not Disturb that allows you to set up different permissions for apps, contacts, and more depending on what you're doing. In iOS 16, Apple has expanded what Focus modes can do with new features, including the ability to add Focus Filters. This article explains how Focus Filters can be used to associate a Safari Tab Group with a Focus.
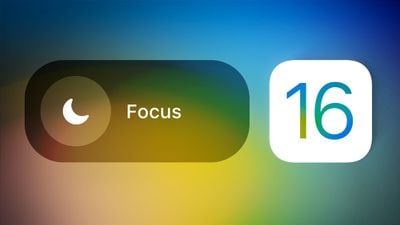
In iOS 16, you can set Safari to only show a specific Tab Group when a Focus is turned on. The idea is that by limiting your browsing to only the webpages in the Tab Group, you can avoid going down a rabbit hole of irrelevant links as well as the temptation of going on social media sites or browsing something equally unrelated to the task at hand.
Before you link a Tab Group to a Focus, you'll need to create a Tab Group ahead of time in the Safari app. Once that's done, you can associate the Tab Group with a Focus profile by following the steps below.
- Launch the Settings app on your iPhone , then tap Focus .
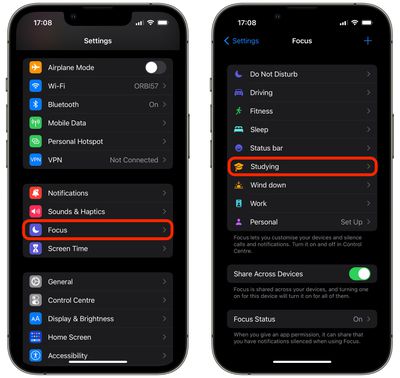
- Scroll down, and under "Focus Filters," tap Add Filter .
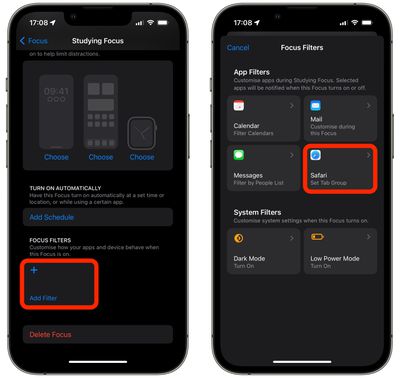
- Tap Choose .
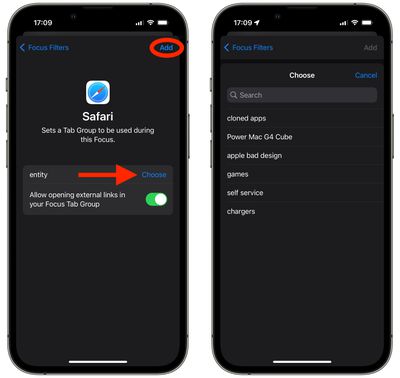
Your Safari Tab Group will now be active the next time you enable the Focus mode. Note that when you choose the Tab Group in Focus, you can also toggle a switch to enable or disable opening external links in your Focus Tab Group .
Get weekly top MacRumors stories in your inbox.
Popular Stories
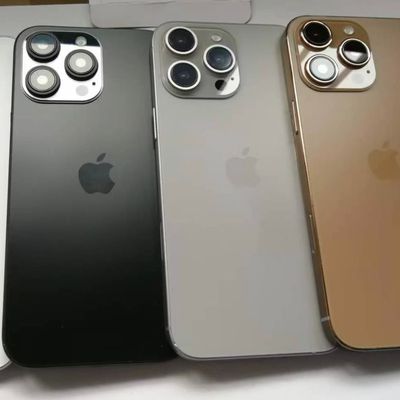
New iPhone 16 and iPhone 16 Pro Colors Revealed Ahead of Apple Event
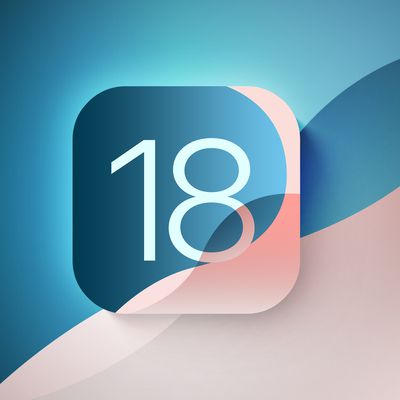
iOS 18 Coming Later This Month With These 8 New Features
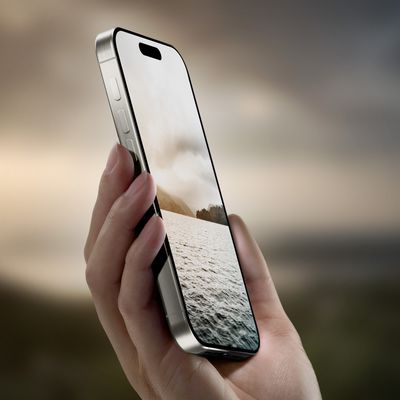
How Much Will the iPhone 16 Cost?
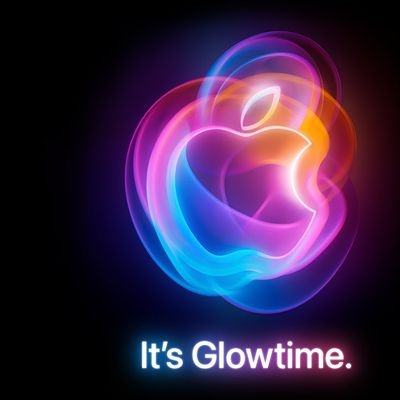
Report Details Last-Minute Apple Event Rumors About New iPhones, Apple Watches, and AirPods
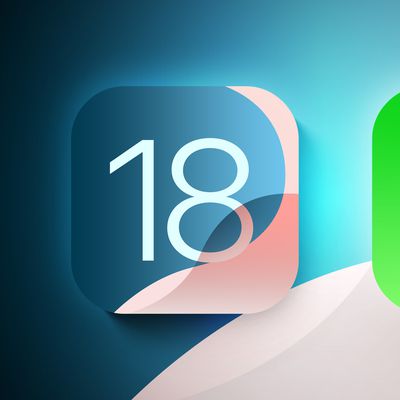
iOS 18 Adds These 6 New Features to CarPlay
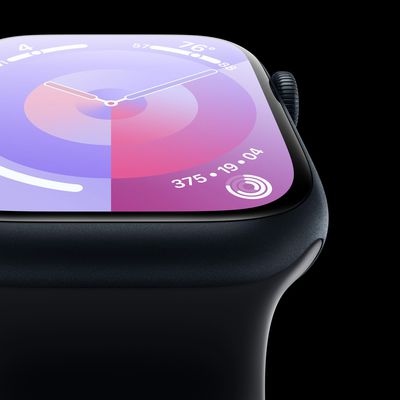
'Noticeably Thinner' Apple Watch Series 10 to Eventually Get Sleep Apnea Detection
Next article.
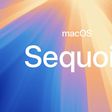
Our comprehensive guide highlighting every major new addition in iOS 17, plus how-tos that walk you through using the new features.
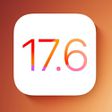
Update now for a number of important security fixes.
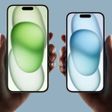
Get the most out your iPhone 15 with our complete guide to all the new features.
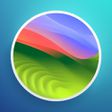
A deep dive into new features in macOS Sonoma, big and small.
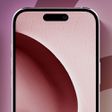
Action button expands to all models, new Capture button, camera improvements, and more.
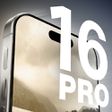
Larger displays, new Capture button, camera improvements, and more.
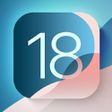
Updates to Mail, Photos, Messages, and more, with Apple Intelligence features throughout.
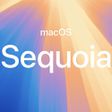
iPhone Mirroring, Safari improvements, new Passwords app, and Apple Intelligence features throughout.
Other Stories
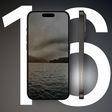
2 days ago by Joe Rossignol
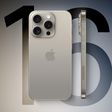
3 days ago by Joe Rossignol
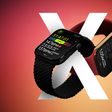
3 days ago by Tim Hardwick
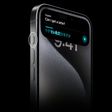
Navigation Menu
Search code, repositories, users, issues, pull requests..., provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications You must be signed in to change notification settings
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement . We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
autoFocus does not work on Safari mobile #3501
csantos1113 commented Apr 4, 2019
- 👀 2 reactions
Rall3n commented Apr 8, 2019 • edited Loading
Sorry, something went wrong.
csantos1113 commented Apr 9, 2019
- 👍 2 reactions
Rall3n commented Apr 9, 2019 • edited Loading
- 👍 4 reactions
karlingen commented Sep 27, 2019
Rall3n commented sep 27, 2019.
- 👍 15 reactions
csantos1113 commented Oct 2, 2019
pulkitaggarwal-nagarro-uf-srch commented Oct 20, 2022
dipanshumahla commented May 1, 2023
damianoc90 commented May 29, 2023
Hyodori04 commented Aug 29, 2023
samrahsajid commented Jul 4, 2024
- 👎 1 reaction
No branches or pull requests
- Skip to main content
- Switch language
- Skip to search
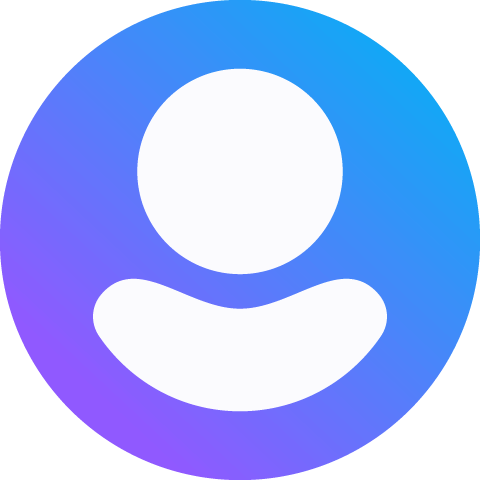
Search Support
- Firefox Focus
- Firefox Focus for iOS
Safari Integration in Firefox iOS
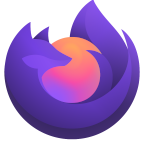
Firefox Focus for iOS makes it simple to use Safari as your preferred web browser while using Firefox Focus features.
Enable Firefox Focus in Safari
- Open FireFox Focus
- Next, tap on Settings
- Under Safari Integration, enable Firefox Focus in Safari by tapping on the switch on the right
- You can now use Safari with Firefox Focus
If your display indicates that Firefox Focus has not been enabled, you'll need to follow a few more steps to get it working properly.
- Tap on the Settings app on your device
- Scroll down on the through the menu on the left-hand side and tap on Safari
- Under General, tap on Extensions
- Tap the switch on the top right corner to enable Firefox Focus
Share this article: https://mzl.la/3zFbC2k
Was this article helpful?
These fine people helped write this article:
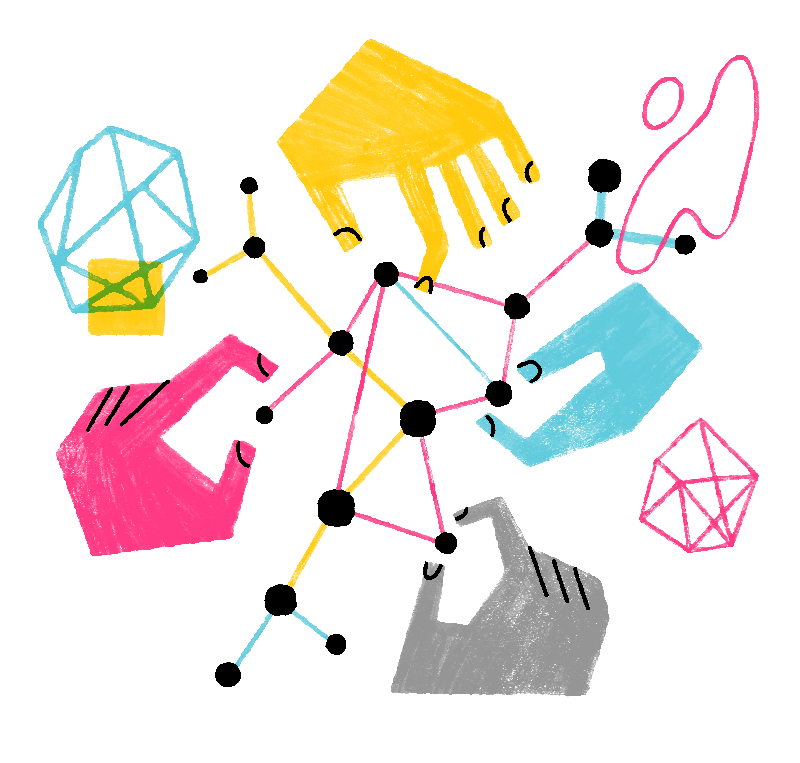
Grow and share your expertise with others. Answer questions and improve our knowledge base.
14 shortcuts for Safari Views, Tab Groups, and Focus Filters to use with iOS 16
Safari is getting easier to work with inside Shortcuts — these shortcuts will help you get started.
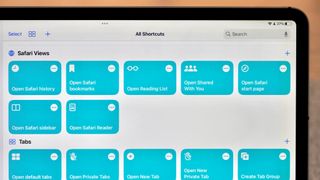
New actions
Safari views, focus filters.
In the iOS 16 public beta, Apple has added six new actions to the Shortcuts app that work with Safari on iPhone and iPad.
Currently only available for developers and public testers (but coming to everyone this fall), these actions are aimed at interacting with different "views" in Safari, plus creating tabs and doing basic Tab Group management.
To help you take full advantage of these, here are 14 shortcuts built out with each potential option across the actions so that you don't have to build them yourself — if you're brave enough to install the betas, that is (if not, just bookmark this story):
- Open Safari Bookmarks
- Open Safari History
- Open Reading List
- Open Shared With You
- Open Safari start page
- Open Safari sidebar
- Open Tab Overview
- Open new tab
- Open new private tab
- Open Default tabs
- Open Private tabs
- Create Tab Group
- Open Safari Tab Group
- Set Safari Focus Filter
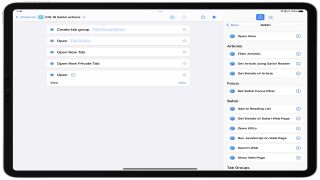
Released alongside 40 other actions , Safari has gained six actions of its own so far in the betas (descriptions from Apple):
1. Open View: Opens the view in Safari.
2. Open New Tab: Opens a new tab.
3. Open New Private Tab: Opens a new Private Tab.
Master your iPhone in minutes
iMore offers spot-on advice and guidance from our team of experts, with decades of Apple device experience to lean on. Learn more with iMore!
4. Create Tab Group: Creates an empty Tab Group.
5. Open Tab Group: Opens the selected Tab Group.
6. Set Safari Focus Filter: Sets the behavior of the safari when the given Focus in enabled.
In addition, Safari Reader actions are also now available on macOS , matching the functionality from iOS and iPadOS that's been missing the last year since Shortcuts for Mac launched — we'll cover how to take advantage of those in a future story.
The Open View action in Safari packs a lot of functionality into a single action, providing access to every screen you can find in the Safari app.
We've built shortcuts for each option, letting you speak the names to Siri and immediately access the specific portion of the app when needed. Plus, this set works great as a Shortcuts widget to quickly pick between every option and get to the right spot.
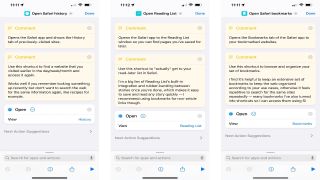
- Open Safari Bookmarks: Opens the Bookmarks tab of the Safari app to your bookmarked websites.
- Open Safari History: Opens the Safari app and shows the History tab of previously-visited sites. Use this shortcut to find a website that you visited earlier in the day/week/month and access it again — it works well if you remember looking something up recently but don't want to search the web for the same information again, like recipes for example.
- Open Reading List: Opens the Safari app to the Reading List window so you can find pages you've saved for later. Use this shortcut to actually get to your read-later list in Safari.
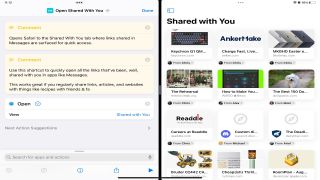
- Open Shared With You: Opens Safari to the Shared With You tab where links shared in Messages are surfaced for quick access. Use this shortcut to quickly open all the links that have been, well, shared with you in apps like Messages – this works great if you regularly share links, articles, and websites with things like recipes with friends and family from your favorite iPad .
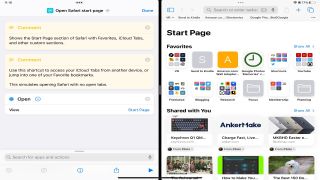
- Open Safari start page: Shows the Start Page section of Safari with Favorites, iCloud Tabs, and other custom sections. Use this shortcut to access your iCloud Tabs from another device or jump into one of your Favorite bookmarks.
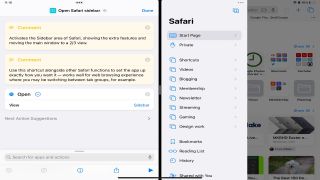
- Open Safari sidebar: Activates the Sidebar area of Safari, showing the extra features and moving the main window to a 2/3 view. Use this shortcut alongside other Safari functions to set the app up exactly how you want it — it works well for a web browsing experience where you may be switching between tab groups, for example.
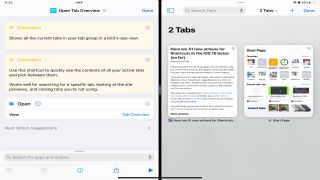
- Open Tab Overview: Shows all the current tabs in your tab group in a bird's eye view. Use this shortcut to quickly see the contents of all your active tabs and pick between them — it works well for searching for a specific tab, looking at the site previews, and closing tabs you're not using.
To work with tabs and tab groups, Safari has options to create new tabs, plus create a new tab group and reopen it as needed. We've included the Open View actions for Open Default Tab View and Open Private Tab View here as well since they align well with the New Tab and New Private Tab actions.
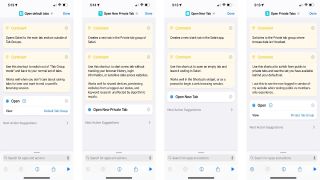
- Open new tab : Creates a new blank tab in the Safari app. Use this shortcut to open an empty tab and leave it waiting in Safari — it works well in the Shortcuts widget, or as a prompt to begin a web browsing session. Oddly, this doesn't let you specify a Tab Group to create it in (yet?).
- Open new private tab: Creates a new tab in the Private tab group of Safari.
- Open Default tabs: Opens Safari to the main tab section outside of Tab Groups. Use this shortcut to switch out of "Tab Group mode" and back to your normal set of tabs. Works well when you don't care about saving tabs for later and want to end a specific browsing session.
- Open Private tabs: Switches to the Private tab group where browse data isn't tracked. Use this shortcut to switch from public to private tabs and see the set you have available behind your default set. I use this to see the non-logged-in version of my website when testing the public vs. members-only experience.
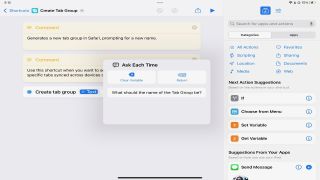
- Create Tab Group: Generates a new tab group in Safari, prompting for a new name. Use this shortcut when you want to set up a new Tab Group and keep track of specific tabs synced across devices over time.
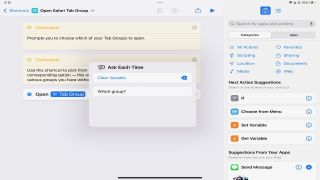
- Open Safari Tab Group: Prompts you to choose which of your Tab Groups to open. Use this shortcut to pick from your full list of tab groups and open the corresponding option — this works well as a Shortcuts widget to jump into the various groups you have without tapping around the Safari app multiple times.
Try duplicating this shortcut and create new shortcuts for each of your tab groups so you can access each of them with Siri too.
Finally, Safari is one of the apps that Apple added Focus Filters actions for (so far?) — this feature lets you automatically switch to a specific tab group when you activate a Focus mode, and this action lets you control that functionality entirely from within Shortcuts.
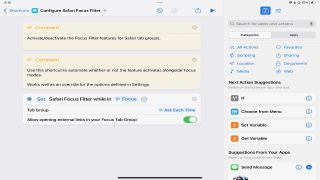
- Set Safari Focus Filter: Activate/deactivate the Focus Filter features for Safari tab groups by asking for a Focus, then which Tab Group to set. Use this shortcut to automate whether or not the feature activates alongside Focus modes — works well as an override for the Focus options defined in Settings.
These handy new Shortcuts make Safari a breeze
With that set of shortcuts, you now have a complete basis for interacting with Safari in iOS 16 — you can access everything, work with tabs, and get even deeper with Tab Groups and Focus Filters.
Currently, these actions do not work in the macOS Ventura betas — while I assume this is only temporary in the earlier betas, it's possible these may not come to the Mac. This story will be updated in future betas if more information is discovered.
This overall release is also missing a set of actions for "getting" the current tabs from Safari and your various tab groups. On the Mac, there is an action called Get Current Tab from Safari that has existed since last year that was brought over from Automator.
However, that action hasn't been brought to iOS, updated for Tab Groups, and doesn't let you get anything except the front-most tab. I hope Apple fixes this in the next few betas, as I'd like to see a Get Tabs From Safari action added in this iOS 16 release so Shortcuts users can truly take advantage of all their tabs and Tab Group setups to the fullest.
We'll be covering how to use the Safari Reader actions on the Mac in the future, plus sharing more advanced use cases for these actions near to the iOS 16 launch — until then, enjoy playing with these shortcuts on the betas.
Matthew Cassinelli is a writer, podcaster, video producer, and Shortcuts creator. After working on the Workflow app before it was acquired by Apple and turned into Shortcuts, Matthew now shares about how to use Shortcuts and how to get things done with Apple technology.
On his personal website MatthewCassinelli.com, Matthew has shared hundreds & hundreds of shortcuts that anyone can download, plus runs a membership program for more advanced Shortcuts users. He also publishes a weekly newsletter called “What’s New in Shortcuts.”
iOS 18 may actually make Live Activities less useful
Here's how to add some flair to your messages with new iOS 18 text effects
Every iPhone 16 event announcement just leaked, including Apple Watch X, Apple Watch SE, and budget AirPods
Most Popular
- 2 On eve of iPhone 16 launch, UGREEN announces new line of charging devices
- 3 Google Photos gets an Apple Intelligence-inspired update to help you find your favorite snaps
- 4 TIME names the 100 most influential people in AI, but Tim Cook is nowhere to be seen
- 5 The Powerbeats Pro 2 are real and they're coming next year
- apple event
- Services & Software
iOS 18 Beta: Easily Get Rid of Banners and More on Safari Webpages
Turn some website elements to dust in the blink of an eye, like they were snapped by Thanos.

- Apple software beta tester, "Helps make our computers and phones work!" - Zach's grandparents

Apple released iOS 18's sixth public beta on Aug. 29, a few days after the tech giant announced it would hold its iPhone launch event on Monday, Sept. 9 . The beta brings new features to the iPhones of developers and beta testers, like the ability to customize your home screen and RCS messaging . It also brings a new feature to your Safari app: Distraction Control, which lets you hide banners and other elements on websites you visit.
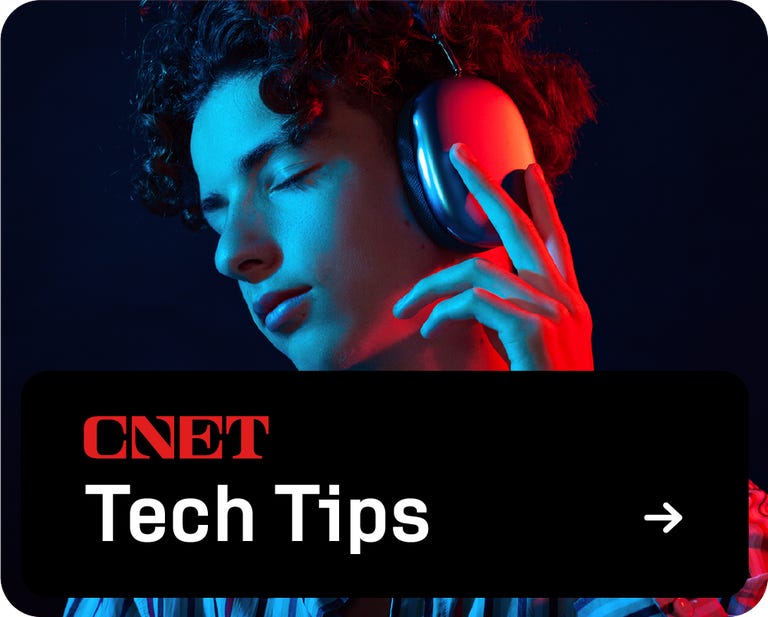
Read more: iPhone 16: What We Know About the Release Date, Leaks and More
Because this operating system is still in beta, I recommend downloading it only on something other than your primary device. Because this isn't the final version of iOS 18, the update might be buggy and battery life may be short, and it's best to keep those troubles on a secondary device. For example, I download and test iOS betas on an old iPhone XR to keep any issues away from my primary phone, an iPhone 14 Pro .
Here's what to know about Safari's Distraction Control feature.
Read more: Everything to Know About iOS 18's Public Beta
How to hide website elements with Distraction Control
1. Open Safari. 2. Go to a webpage. 3. Tap the Reader icon in your address bar -- it's on the left side of the bar and looks like a rectangle with two dashes beneath it. 4. Tap the three dots ( ... ). 5. Tap Hide Distracting Items .
Safari will take you back to the website, and you now have to select which items to hide. Once you've tapped an item, a perimeter will appear around it with the word Hide in the center. Tap Hide and the item will disappear like it was snapped by Thanos in the Avengers.

Already receive our newsletters and don't need to see the signup form anymore? Now you don't have to!
What Distraction Control hides
Distraction Control can hide certain banners, pop-ups and other items you might see on a webpage. For instance, if you visit a news site and always see a form to receive that site's newsletter, you can block the form from appearing now and every time you visit the website later. Once you enable Distraction Control, you have to select which items on a webpage the feature targets.
Will Distraction Control work on every website?
Distraction Control can work on every website, but you have to enable it on each individual webpage. For every site you visit, you have to turn Distraction Control on. That means you can't enable the feature once and be done.
Will Distraction Control always hide items?
Not necessarily. Distraction Control won't permanently remove ads or other areas that constantly change. For example, you can't hide an ad that's in a window within a webpage, but you can hide pop-up requests to create an account.
You can also see what Distraction Control is blocking if you want to take a peek. If you enable Distraction Control on a certain webpage, a little blue indicator will appear in your address bar to let you know the feature is working on that page. You can click this indicator and then click Show Hidden Items to reveal everything that Distraction Control is hiding.
Distraction Control is still in beta, so it might be buggy or not work properly. There will likely be more betas before the feature is released to the public, so there's plenty of time for Apple to address any issues. As of now, there's no word on when Apple will release Distraction Control to the general public.
For more on Apple, here's what to know about iOS 18 , why the iPhone 16 Plus could be Apple's last supersized smartphone and our iOS 18 cheat sheet . You can also check out what we might see at Apple's September iPhone event .

Services and Software Guides
- Best iPhone VPN
- Best Free VPN
- Best Android VPN
- Best Mac VPN
- Best Mobile VPN
- Best VPN for Firestick
- Best VPN for Windows
- Fastest VPN
- Best Cheap VPN
- Best Password Manager
- Best Antivirus
- Best Identity Theft Protection
- Best LastPass Alternative
- Best Live TV Streaming Service
- Best Streaming Service
- Best Free TV Streaming Service
- Best Music Streaming Services
- Best Web Hosting
- Best Minecraft Server Hosting
- Best Website Builder
- Best Dating Sites
- Best Language Learning Apps
- Best Weather App
- Best Stargazing Apps
- Best Cloud Storage
- Best Resume Writing Services
- New Coverage on Operating Systems

IMAGES
VIDEO
COMMENTS
Safari: focus event doesn't work on button element
Fixing Focus for Safari. In 2008, Apple's Safari team had a problem: web developers had noticed that links, buttons, and several input elements were not matching the :focus selector when clicked. Similarly, clicking on those elements did not fire a focus or focusin event. The developers dutifully submitted bugs against Webkit and were waiting ...
Oh well, no big deal, we can fix that with a little bit of Javascript. .filter((el) => el.hasAttribute('autofocus'))[0] .focus() To your great surprise, you discover that this is not working ...
Focus Mode Not Working? Try These 8 Troubleshooting Tips
Open the Settings app on your iPhone and tap on Focus. Now tap on the concerned focus mode that the app can bypass on your device. Tap on Apps under "Allowed Notifications" at the top of the screen. Now check to see if the current app is available under the 'Allowed Apps' section. If it is, tap on the minus (-) to remove it from the ...
In this case when you change focus from one field to another Safari has some kind of issue; the page "bounce" and the focused field is not centered in the page. I have made a video of a simple page that has this issue. In the first part of the video the page without the iframe is loaded and the focus works correctly.
offsky commented on Nov 10, 2011. When I call .focus () on one of my text inputs or textareas, the iPhone keyboard does not automatically appear. Tested on iPhone 4 running iOS5. This may be a jquery issue instead of jquerymobile, Im not sure. But, it would be nice if there was some workaround either way.
Safari Search bar, will not allow me to select anything below the first suggestion, automatically picks the top item. Whenever I type something in the search bar on Safari and the suggestions pop up and I scroll down with my keyboard, the highlighted line keeps returning to the top line and highlighting that one.
So I'm trying to get a web page to force browser focus onto a SWF once a page loads. The code works fine in Firefox (naturally) and in IE (very surprisingly). But focus() seems to not work at all in Safari. I've reinstalled Safari, yet the problem persists. Did Safari's developers decide to abandon focus()? Javascript code as follows:
Angular solution: on button click we need to create temporary input, append to existing container (close to our input) and focus on it. btnClicked() {. this.showModal = true; this.searchBar = this.renderer2.selectRootElement('#searchBar', true); // 2nd argument preserves existing content.
iOS 16: How to Limit Browsing to a Specific Safari Tab ...
@karlingen Yes, we have. There was a comment about it in a ticket for WebKit. The comment was created March 2019, and states the following: "We (Apple) like the current behavior and do not want programmatic focus to bring up the keyboard when [...] the programmatic focus was not invoked in response to a user gesture."
2: Safari is adding a solid 6-8rem height whitespace outside of the html block of the page, which then sits between the lower browser chrome and and actual bottom of the page. Of course everything is working as expected in Chrome on iPhone: Chrome (expected behavior):
Firefox Focus for iOS makes it simple to use Safari as your preferred web browser while using Firefox Focus features. Enable Firefox Focus in Safari. Open FireFox Focus. Tap the menu on the top right corner of the screen: Next, tap on Settings. Under Safari Integration, enable Firefox Focus in Safari by tapping on the switch on the right.
Under Allowed Apps, ensure that Safari is not restricted. Update iOS. Make sure your iPhone is running the latest version of iOS, as updates often include fixes for common issues: ... If Safari is ...
This is known not to work on ios safari. Safari specifically distinguishes between events generated by user interaction vs generated purely from the js itself, and won't deliver focus/select events this way. -
4. Create Tab Group: Creates an empty Tab Group. 5. Open Tab Group: Opens the selected Tab Group. 6. Set Safari Focus Filter: Sets the behavior of the safari when the given Focus in enabled. In addition, Safari Reader actions are also now available on macOS, matching the functionality from iOS and iPadOS that's been missing the last year since ...
For this particular field there are 4 text boxes. Upon entering details within one text box my focus should shift automatically to the next input box. I am currently using "$ ("#ATMCardNumber").focus ();" which seems to be working on Android devices however for the iOS devices the keyboard does not automatically open after the change of focus. 0.
44. So I've seen a lot of threads about the iOS issue with focusing on an input/textarea element. (See here and here) It seems that iOS will not let you manually focus on one of these elements, and requires it to be a genuine tap/click to focus on the element. I've tried simulating a click, triggering a click, simply doing click () straight ...
Distraction Control is still in beta, so it might be buggy or not work properly. There will likely be more betas before the feature is released to the public, so there's plenty of time for Apple ...
focus() is not working in ionic using ios 10 safari browser. It works when you use chrome emulator but not when use ios 10 safari browser. below is the code i'm using to focus element.
I'm building a counter component in a React application using framer-motion for the animation. The counter increments every second, and the animation works perfectly on web browsers like Chrome and Firefox, but sometimes the counter doesn't even appear on iOS (iPhone) Safari. Here is my code